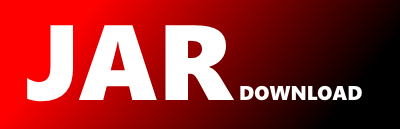
com.pulumi.vault.pkiSecret.kotlin.SecretBackendConfigUrlsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.pkiSecret.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.pkiSecret.SecretBackendConfigUrlsArgs.builder
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Allows setting the issuing certificate endpoints, CRL distribution points, and OCSP server endpoints that will be encoded into issued certificates.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const root = new vault.Mount("root", {
* path: "pki-root",
* type: "pki",
* description: "root PKI",
* defaultLeaseTtlSeconds: 8640000,
* maxLeaseTtlSeconds: 8640000,
* });
* const example = new vault.pkisecret.SecretBackendConfigUrls("example", {
* backend: root.path,
* issuingCertificates: ["http://127.0.0.1:8200/v1/pki/ca"],
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* root = vault.Mount("root",
* path="pki-root",
* type="pki",
* description="root PKI",
* default_lease_ttl_seconds=8640000,
* max_lease_ttl_seconds=8640000)
* example = vault.pki_secret.SecretBackendConfigUrls("example",
* backend=root.path,
* issuing_certificates=["http://127.0.0.1:8200/v1/pki/ca"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var root = new Vault.Mount("root", new()
* {
* Path = "pki-root",
* Type = "pki",
* Description = "root PKI",
* DefaultLeaseTtlSeconds = 8640000,
* MaxLeaseTtlSeconds = 8640000,
* });
* var example = new Vault.PkiSecret.SecretBackendConfigUrls("example", new()
* {
* Backend = root.Path,
* IssuingCertificates = new[]
* {
* "http://127.0.0.1:8200/v1/pki/ca",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/pkiSecret"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* root, err := vault.NewMount(ctx, "root", &vault.MountArgs{
* Path: pulumi.String("pki-root"),
* Type: pulumi.String("pki"),
* Description: pulumi.String("root PKI"),
* DefaultLeaseTtlSeconds: pulumi.Int(8640000),
* MaxLeaseTtlSeconds: pulumi.Int(8640000),
* })
* if err != nil {
* return err
* }
* _, err = pkiSecret.NewSecretBackendConfigUrls(ctx, "example", &pkiSecret.SecretBackendConfigUrlsArgs{
* Backend: root.Path,
* IssuingCertificates: pulumi.StringArray{
* pulumi.String("http://127.0.0.1:8200/v1/pki/ca"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.Mount;
* import com.pulumi.vault.MountArgs;
* import com.pulumi.vault.pkiSecret.SecretBackendConfigUrls;
* import com.pulumi.vault.pkiSecret.SecretBackendConfigUrlsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var root = new Mount("root", MountArgs.builder()
* .path("pki-root")
* .type("pki")
* .description("root PKI")
* .defaultLeaseTtlSeconds(8640000)
* .maxLeaseTtlSeconds(8640000)
* .build());
* var example = new SecretBackendConfigUrls("example", SecretBackendConfigUrlsArgs.builder()
* .backend(root.path())
* .issuingCertificates("http://127.0.0.1:8200/v1/pki/ca")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* root:
* type: vault:Mount
* properties:
* path: pki-root
* type: pki
* description: root PKI
* defaultLeaseTtlSeconds: 8.64e+06
* maxLeaseTtlSeconds: 8.64e+06
* example:
* type: vault:pkiSecret:SecretBackendConfigUrls
* properties:
* backend: ${root.path}
* issuingCertificates:
* - http://127.0.0.1:8200/v1/pki/ca
* ```
*
* ## Import
* The PKI config URLs can be imported using the resource's `id`.
* In the case of the example above the `id` would be `pki-root/config/urls`,
* where the `pki-root` component is the resource's `backend`, e.g.
* ```sh
* $ pulumi import vault:pkiSecret/secretBackendConfigUrls:SecretBackendConfigUrls example pki-root/config/urls
* ```
* @property backend The path the PKI secret backend is mounted at, with no leading or trailing `/`s.
* @property crlDistributionPoints Specifies the URL values for the CRL Distribution Points field.
* @property enableTemplating Specifies that templating of AIA fields is allowed.
* @property issuingCertificates Specifies the URL values for the Issuing Certificate field.
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property ocspServers Specifies the URL values for the OCSP Servers field.
*/
public data class SecretBackendConfigUrlsArgs(
public val backend: Output? = null,
public val crlDistributionPoints: Output>? = null,
public val enableTemplating: Output? = null,
public val issuingCertificates: Output>? = null,
public val namespace: Output? = null,
public val ocspServers: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.pkiSecret.SecretBackendConfigUrlsArgs =
com.pulumi.vault.pkiSecret.SecretBackendConfigUrlsArgs.builder()
.backend(backend?.applyValue({ args0 -> args0 }))
.crlDistributionPoints(crlDistributionPoints?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.enableTemplating(enableTemplating?.applyValue({ args0 -> args0 }))
.issuingCertificates(issuingCertificates?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.ocspServers(ocspServers?.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [SecretBackendConfigUrlsArgs].
*/
@PulumiTagMarker
public class SecretBackendConfigUrlsArgsBuilder internal constructor() {
private var backend: Output? = null
private var crlDistributionPoints: Output>? = null
private var enableTemplating: Output? = null
private var issuingCertificates: Output>? = null
private var namespace: Output? = null
private var ocspServers: Output>? = null
/**
* @param value The path the PKI secret backend is mounted at, with no leading or trailing `/`s.
*/
@JvmName("tdksypkwdlggtxhi")
public suspend fun backend(`value`: Output) {
this.backend = value
}
/**
* @param value Specifies the URL values for the CRL Distribution Points field.
*/
@JvmName("olxpgrkuvymevqol")
public suspend fun crlDistributionPoints(`value`: Output>) {
this.crlDistributionPoints = value
}
@JvmName("jqdkyswuwwavuils")
public suspend fun crlDistributionPoints(vararg values: Output) {
this.crlDistributionPoints = Output.all(values.asList())
}
/**
* @param values Specifies the URL values for the CRL Distribution Points field.
*/
@JvmName("vcgjfgkmlgkfkhoj")
public suspend fun crlDistributionPoints(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy