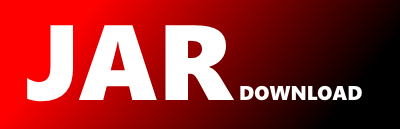
com.pulumi.vault.pkiSecret.kotlin.SecretBackendIntermediateSetSigned.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.pkiSecret.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
/**
* Builder for [SecretBackendIntermediateSetSigned].
*/
@PulumiTagMarker
public class SecretBackendIntermediateSetSignedResourceBuilder internal constructor() {
public var name: String? = null
public var args: SecretBackendIntermediateSetSignedArgs = SecretBackendIntermediateSetSignedArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend SecretBackendIntermediateSetSignedArgsBuilder.() -> Unit) {
val builder = SecretBackendIntermediateSetSignedArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): SecretBackendIntermediateSetSigned {
val builtJavaResource =
com.pulumi.vault.pkiSecret.SecretBackendIntermediateSetSigned(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return SecretBackendIntermediateSetSigned(builtJavaResource)
}
}
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const root = new vault.Mount("root", {
* path: "pki-root",
* type: "pki",
* description: "root",
* defaultLeaseTtlSeconds: 8640000,
* maxLeaseTtlSeconds: 8640000,
* });
* const intermediate = new vault.Mount("intermediate", {
* path: "pki-int",
* type: root.type,
* description: "intermediate",
* defaultLeaseTtlSeconds: 86400,
* maxLeaseTtlSeconds: 86400,
* });
* const example = new vault.pkisecret.SecretBackendRootCert("example", {
* backend: root.path,
* type: "internal",
* commonName: "RootOrg Root CA",
* ttl: "86400",
* format: "pem",
* privateKeyFormat: "der",
* keyType: "rsa",
* keyBits: 4096,
* excludeCnFromSans: true,
* ou: "Organizational Unit",
* organization: "RootOrg",
* country: "US",
* locality: "San Francisco",
* province: "CA",
* });
* const exampleSecretBackendIntermediateCertRequest = new vault.pkisecret.SecretBackendIntermediateCertRequest("example", {
* backend: intermediate.path,
* type: example.type,
* commonName: "SubOrg Intermediate CA",
* });
* const exampleSecretBackendRootSignIntermediate = new vault.pkisecret.SecretBackendRootSignIntermediate("example", {
* backend: root.path,
* csr: exampleSecretBackendIntermediateCertRequest.csr,
* commonName: "SubOrg Intermediate CA",
* excludeCnFromSans: true,
* ou: "SubUnit",
* organization: "SubOrg",
* country: "US",
* locality: "San Francisco",
* province: "CA",
* revoke: true,
* });
* const exampleSecretBackendIntermediateSetSigned = new vault.pkisecret.SecretBackendIntermediateSetSigned("example", {
* backend: intermediate.path,
* certificate: exampleSecretBackendRootSignIntermediate.certificate,
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* root = vault.Mount("root",
* path="pki-root",
* type="pki",
* description="root",
* default_lease_ttl_seconds=8640000,
* max_lease_ttl_seconds=8640000)
* intermediate = vault.Mount("intermediate",
* path="pki-int",
* type=root.type,
* description="intermediate",
* default_lease_ttl_seconds=86400,
* max_lease_ttl_seconds=86400)
* example = vault.pki_secret.SecretBackendRootCert("example",
* backend=root.path,
* type="internal",
* common_name="RootOrg Root CA",
* ttl="86400",
* format="pem",
* private_key_format="der",
* key_type="rsa",
* key_bits=4096,
* exclude_cn_from_sans=True,
* ou="Organizational Unit",
* organization="RootOrg",
* country="US",
* locality="San Francisco",
* province="CA")
* example_secret_backend_intermediate_cert_request = vault.pki_secret.SecretBackendIntermediateCertRequest("example",
* backend=intermediate.path,
* type=example.type,
* common_name="SubOrg Intermediate CA")
* example_secret_backend_root_sign_intermediate = vault.pki_secret.SecretBackendRootSignIntermediate("example",
* backend=root.path,
* csr=example_secret_backend_intermediate_cert_request.csr,
* common_name="SubOrg Intermediate CA",
* exclude_cn_from_sans=True,
* ou="SubUnit",
* organization="SubOrg",
* country="US",
* locality="San Francisco",
* province="CA",
* revoke=True)
* example_secret_backend_intermediate_set_signed = vault.pki_secret.SecretBackendIntermediateSetSigned("example",
* backend=intermediate.path,
* certificate=example_secret_backend_root_sign_intermediate.certificate)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var root = new Vault.Mount("root", new()
* {
* Path = "pki-root",
* Type = "pki",
* Description = "root",
* DefaultLeaseTtlSeconds = 8640000,
* MaxLeaseTtlSeconds = 8640000,
* });
* var intermediate = new Vault.Mount("intermediate", new()
* {
* Path = "pki-int",
* Type = root.Type,
* Description = "intermediate",
* DefaultLeaseTtlSeconds = 86400,
* MaxLeaseTtlSeconds = 86400,
* });
* var example = new Vault.PkiSecret.SecretBackendRootCert("example", new()
* {
* Backend = root.Path,
* Type = "internal",
* CommonName = "RootOrg Root CA",
* Ttl = "86400",
* Format = "pem",
* PrivateKeyFormat = "der",
* KeyType = "rsa",
* KeyBits = 4096,
* ExcludeCnFromSans = true,
* Ou = "Organizational Unit",
* Organization = "RootOrg",
* Country = "US",
* Locality = "San Francisco",
* Province = "CA",
* });
* var exampleSecretBackendIntermediateCertRequest = new Vault.PkiSecret.SecretBackendIntermediateCertRequest("example", new()
* {
* Backend = intermediate.Path,
* Type = example.Type,
* CommonName = "SubOrg Intermediate CA",
* });
* var exampleSecretBackendRootSignIntermediate = new Vault.PkiSecret.SecretBackendRootSignIntermediate("example", new()
* {
* Backend = root.Path,
* Csr = exampleSecretBackendIntermediateCertRequest.Csr,
* CommonName = "SubOrg Intermediate CA",
* ExcludeCnFromSans = true,
* Ou = "SubUnit",
* Organization = "SubOrg",
* Country = "US",
* Locality = "San Francisco",
* Province = "CA",
* Revoke = true,
* });
* var exampleSecretBackendIntermediateSetSigned = new Vault.PkiSecret.SecretBackendIntermediateSetSigned("example", new()
* {
* Backend = intermediate.Path,
* Certificate = exampleSecretBackendRootSignIntermediate.Certificate,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/pkiSecret"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* root, err := vault.NewMount(ctx, "root", &vault.MountArgs{
* Path: pulumi.String("pki-root"),
* Type: pulumi.String("pki"),
* Description: pulumi.String("root"),
* DefaultLeaseTtlSeconds: pulumi.Int(8640000),
* MaxLeaseTtlSeconds: pulumi.Int(8640000),
* })
* if err != nil {
* return err
* }
* intermediate, err := vault.NewMount(ctx, "intermediate", &vault.MountArgs{
* Path: pulumi.String("pki-int"),
* Type: root.Type,
* Description: pulumi.String("intermediate"),
* DefaultLeaseTtlSeconds: pulumi.Int(86400),
* MaxLeaseTtlSeconds: pulumi.Int(86400),
* })
* if err != nil {
* return err
* }
* example, err := pkiSecret.NewSecretBackendRootCert(ctx, "example", &pkiSecret.SecretBackendRootCertArgs{
* Backend: root.Path,
* Type: pulumi.String("internal"),
* CommonName: pulumi.String("RootOrg Root CA"),
* Ttl: pulumi.String("86400"),
* Format: pulumi.String("pem"),
* PrivateKeyFormat: pulumi.String("der"),
* KeyType: pulumi.String("rsa"),
* KeyBits: pulumi.Int(4096),
* ExcludeCnFromSans: pulumi.Bool(true),
* Ou: pulumi.String("Organizational Unit"),
* Organization: pulumi.String("RootOrg"),
* Country: pulumi.String("US"),
* Locality: pulumi.String("San Francisco"),
* Province: pulumi.String("CA"),
* })
* if err != nil {
* return err
* }
* exampleSecretBackendIntermediateCertRequest, err := pkiSecret.NewSecretBackendIntermediateCertRequest(ctx, "example", &pkiSecret.SecretBackendIntermediateCertRequestArgs{
* Backend: intermediate.Path,
* Type: example.Type,
* CommonName: pulumi.String("SubOrg Intermediate CA"),
* })
* if err != nil {
* return err
* }
* exampleSecretBackendRootSignIntermediate, err := pkiSecret.NewSecretBackendRootSignIntermediate(ctx, "example", &pkiSecret.SecretBackendRootSignIntermediateArgs{
* Backend: root.Path,
* Csr: exampleSecretBackendIntermediateCertRequest.Csr,
* CommonName: pulumi.String("SubOrg Intermediate CA"),
* ExcludeCnFromSans: pulumi.Bool(true),
* Ou: pulumi.String("SubUnit"),
* Organization: pulumi.String("SubOrg"),
* Country: pulumi.String("US"),
* Locality: pulumi.String("San Francisco"),
* Province: pulumi.String("CA"),
* Revoke: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* _, err = pkiSecret.NewSecretBackendIntermediateSetSigned(ctx, "example", &pkiSecret.SecretBackendIntermediateSetSignedArgs{
* Backend: intermediate.Path,
* Certificate: exampleSecretBackendRootSignIntermediate.Certificate,
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.Mount;
* import com.pulumi.vault.MountArgs;
* import com.pulumi.vault.pkiSecret.SecretBackendRootCert;
* import com.pulumi.vault.pkiSecret.SecretBackendRootCertArgs;
* import com.pulumi.vault.pkiSecret.SecretBackendIntermediateCertRequest;
* import com.pulumi.vault.pkiSecret.SecretBackendIntermediateCertRequestArgs;
* import com.pulumi.vault.pkiSecret.SecretBackendRootSignIntermediate;
* import com.pulumi.vault.pkiSecret.SecretBackendRootSignIntermediateArgs;
* import com.pulumi.vault.pkiSecret.SecretBackendIntermediateSetSigned;
* import com.pulumi.vault.pkiSecret.SecretBackendIntermediateSetSignedArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var root = new Mount("root", MountArgs.builder()
* .path("pki-root")
* .type("pki")
* .description("root")
* .defaultLeaseTtlSeconds(8640000)
* .maxLeaseTtlSeconds(8640000)
* .build());
* var intermediate = new Mount("intermediate", MountArgs.builder()
* .path("pki-int")
* .type(root.type())
* .description("intermediate")
* .defaultLeaseTtlSeconds(86400)
* .maxLeaseTtlSeconds(86400)
* .build());
* var example = new SecretBackendRootCert("example", SecretBackendRootCertArgs.builder()
* .backend(root.path())
* .type("internal")
* .commonName("RootOrg Root CA")
* .ttl(86400)
* .format("pem")
* .privateKeyFormat("der")
* .keyType("rsa")
* .keyBits(4096)
* .excludeCnFromSans(true)
* .ou("Organizational Unit")
* .organization("RootOrg")
* .country("US")
* .locality("San Francisco")
* .province("CA")
* .build());
* var exampleSecretBackendIntermediateCertRequest = new SecretBackendIntermediateCertRequest("exampleSecretBackendIntermediateCertRequest", SecretBackendIntermediateCertRequestArgs.builder()
* .backend(intermediate.path())
* .type(example.type())
* .commonName("SubOrg Intermediate CA")
* .build());
* var exampleSecretBackendRootSignIntermediate = new SecretBackendRootSignIntermediate("exampleSecretBackendRootSignIntermediate", SecretBackendRootSignIntermediateArgs.builder()
* .backend(root.path())
* .csr(exampleSecretBackendIntermediateCertRequest.csr())
* .commonName("SubOrg Intermediate CA")
* .excludeCnFromSans(true)
* .ou("SubUnit")
* .organization("SubOrg")
* .country("US")
* .locality("San Francisco")
* .province("CA")
* .revoke(true)
* .build());
* var exampleSecretBackendIntermediateSetSigned = new SecretBackendIntermediateSetSigned("exampleSecretBackendIntermediateSetSigned", SecretBackendIntermediateSetSignedArgs.builder()
* .backend(intermediate.path())
* .certificate(exampleSecretBackendRootSignIntermediate.certificate())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* root:
* type: vault:Mount
* properties:
* path: pki-root
* type: pki
* description: root
* defaultLeaseTtlSeconds: 8.64e+06
* maxLeaseTtlSeconds: 8.64e+06
* intermediate:
* type: vault:Mount
* properties:
* path: pki-int
* type: ${root.type}
* description: intermediate
* defaultLeaseTtlSeconds: 86400
* maxLeaseTtlSeconds: 86400
* example:
* type: vault:pkiSecret:SecretBackendRootCert
* properties:
* backend: ${root.path}
* type: internal
* commonName: RootOrg Root CA
* ttl: 86400
* format: pem
* privateKeyFormat: der
* keyType: rsa
* keyBits: 4096
* excludeCnFromSans: true
* ou: Organizational Unit
* organization: RootOrg
* country: US
* locality: San Francisco
* province: CA
* exampleSecretBackendIntermediateCertRequest:
* type: vault:pkiSecret:SecretBackendIntermediateCertRequest
* name: example
* properties:
* backend: ${intermediate.path}
* type: ${example.type}
* commonName: SubOrg Intermediate CA
* exampleSecretBackendRootSignIntermediate:
* type: vault:pkiSecret:SecretBackendRootSignIntermediate
* name: example
* properties:
* backend: ${root.path}
* csr: ${exampleSecretBackendIntermediateCertRequest.csr}
* commonName: SubOrg Intermediate CA
* excludeCnFromSans: true
* ou: SubUnit
* organization: SubOrg
* country: US
* locality: San Francisco
* province: CA
* revoke: true
* exampleSecretBackendIntermediateSetSigned:
* type: vault:pkiSecret:SecretBackendIntermediateSetSigned
* name: example
* properties:
* backend: ${intermediate.path}
* certificate: ${exampleSecretBackendRootSignIntermediate.certificate}
* ```
*
*/
public class SecretBackendIntermediateSetSigned internal constructor(
override val javaResource: com.pulumi.vault.pkiSecret.SecretBackendIntermediateSetSigned,
) : KotlinCustomResource(javaResource, SecretBackendIntermediateSetSignedMapper) {
/**
* The PKI secret backend the resource belongs to.
*/
public val backend: Output
get() = javaResource.backend().applyValue({ args0 -> args0 })
/**
* Specifies the PEM encoded certificate. May optionally append additional
* CA certificates to populate the whole chain, which will then enable returning the full chain from
* issue and sign operations.
*/
public val certificate: Output
get() = javaResource.certificate().applyValue({ args0 -> args0 })
/**
* The imported issuers indicating which issuers were created as part of
* this request.
*/
public val importedIssuers: Output>
get() = javaResource.importedIssuers().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* The imported keys indicating which keys were created as part of this request.
*/
public val importedKeys: Output>
get() = javaResource.importedKeys().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
public val namespace: Output?
get() = javaResource.namespace().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
}
public object SecretBackendIntermediateSetSignedMapper :
ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.vault.pkiSecret.SecretBackendIntermediateSetSigned::class == javaResource::class
override fun map(javaResource: Resource): SecretBackendIntermediateSetSigned =
SecretBackendIntermediateSetSigned(
javaResource as
com.pulumi.vault.pkiSecret.SecretBackendIntermediateSetSigned,
)
}
/**
* @see [SecretBackendIntermediateSetSigned].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [SecretBackendIntermediateSetSigned].
*/
public suspend fun secretBackendIntermediateSetSigned(
name: String,
block: suspend SecretBackendIntermediateSetSignedResourceBuilder.() -> Unit,
): SecretBackendIntermediateSetSigned {
val builder = SecretBackendIntermediateSetSignedResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [SecretBackendIntermediateSetSigned].
* @param name The _unique_ name of the resulting resource.
*/
public fun secretBackendIntermediateSetSigned(name: String): SecretBackendIntermediateSetSigned {
val builder = SecretBackendIntermediateSetSignedResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy