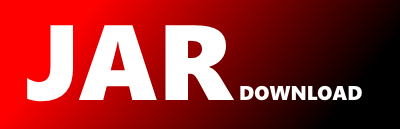
com.pulumi.vault.pkiSecret.kotlin.SecretBackendRole.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.pkiSecret.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import com.pulumi.vault.pkiSecret.kotlin.outputs.SecretBackendRolePolicyIdentifier
import com.pulumi.vault.pkiSecret.kotlin.outputs.SecretBackendRolePolicyIdentifier.Companion.toKotlin
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
/**
* Builder for [SecretBackendRole].
*/
@PulumiTagMarker
public class SecretBackendRoleResourceBuilder internal constructor() {
public var name: String? = null
public var args: SecretBackendRoleArgs = SecretBackendRoleArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend SecretBackendRoleArgsBuilder.() -> Unit) {
val builder = SecretBackendRoleArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): SecretBackendRole {
val builtJavaResource = com.pulumi.vault.pkiSecret.SecretBackendRole(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return SecretBackendRole(builtJavaResource)
}
}
/**
* Creates a role on an PKI Secret Backend for Vault.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const pki = new vault.Mount("pki", {
* path: "pki",
* type: "pki",
* defaultLeaseTtlSeconds: 3600,
* maxLeaseTtlSeconds: 86400,
* });
* const role = new vault.pkisecret.SecretBackendRole("role", {
* backend: pki.path,
* name: "my_role",
* ttl: "3600",
* allowIpSans: true,
* keyType: "rsa",
* keyBits: 4096,
* allowedDomains: [
* "example.com",
* "my.domain",
* ],
* allowSubdomains: true,
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* pki = vault.Mount("pki",
* path="pki",
* type="pki",
* default_lease_ttl_seconds=3600,
* max_lease_ttl_seconds=86400)
* role = vault.pki_secret.SecretBackendRole("role",
* backend=pki.path,
* name="my_role",
* ttl="3600",
* allow_ip_sans=True,
* key_type="rsa",
* key_bits=4096,
* allowed_domains=[
* "example.com",
* "my.domain",
* ],
* allow_subdomains=True)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var pki = new Vault.Mount("pki", new()
* {
* Path = "pki",
* Type = "pki",
* DefaultLeaseTtlSeconds = 3600,
* MaxLeaseTtlSeconds = 86400,
* });
* var role = new Vault.PkiSecret.SecretBackendRole("role", new()
* {
* Backend = pki.Path,
* Name = "my_role",
* Ttl = "3600",
* AllowIpSans = true,
* KeyType = "rsa",
* KeyBits = 4096,
* AllowedDomains = new[]
* {
* "example.com",
* "my.domain",
* },
* AllowSubdomains = true,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/pkiSecret"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* pki, err := vault.NewMount(ctx, "pki", &vault.MountArgs{
* Path: pulumi.String("pki"),
* Type: pulumi.String("pki"),
* DefaultLeaseTtlSeconds: pulumi.Int(3600),
* MaxLeaseTtlSeconds: pulumi.Int(86400),
* })
* if err != nil {
* return err
* }
* _, err = pkiSecret.NewSecretBackendRole(ctx, "role", &pkiSecret.SecretBackendRoleArgs{
* Backend: pki.Path,
* Name: pulumi.String("my_role"),
* Ttl: pulumi.String("3600"),
* AllowIpSans: pulumi.Bool(true),
* KeyType: pulumi.String("rsa"),
* KeyBits: pulumi.Int(4096),
* AllowedDomains: pulumi.StringArray{
* pulumi.String("example.com"),
* pulumi.String("my.domain"),
* },
* AllowSubdomains: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.Mount;
* import com.pulumi.vault.MountArgs;
* import com.pulumi.vault.pkiSecret.SecretBackendRole;
* import com.pulumi.vault.pkiSecret.SecretBackendRoleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var pki = new Mount("pki", MountArgs.builder()
* .path("pki")
* .type("pki")
* .defaultLeaseTtlSeconds(3600)
* .maxLeaseTtlSeconds(86400)
* .build());
* var role = new SecretBackendRole("role", SecretBackendRoleArgs.builder()
* .backend(pki.path())
* .name("my_role")
* .ttl(3600)
* .allowIpSans(true)
* .keyType("rsa")
* .keyBits(4096)
* .allowedDomains(
* "example.com",
* "my.domain")
* .allowSubdomains(true)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* pki:
* type: vault:Mount
* properties:
* path: pki
* type: pki
* defaultLeaseTtlSeconds: 3600
* maxLeaseTtlSeconds: 86400
* role:
* type: vault:pkiSecret:SecretBackendRole
* properties:
* backend: ${pki.path}
* name: my_role
* ttl: 3600
* allowIpSans: true
* keyType: rsa
* keyBits: 4096
* allowedDomains:
* - example.com
* - my.domain
* allowSubdomains: true
* ```
*
* ## Import
* PKI secret backend roles can be imported using the `path`, e.g.
* ```sh
* $ pulumi import vault:pkiSecret/secretBackendRole:SecretBackendRole role pki/roles/my_role
* ```
*/
public class SecretBackendRole internal constructor(
override val javaResource: com.pulumi.vault.pkiSecret.SecretBackendRole,
) : KotlinCustomResource(javaResource, SecretBackendRoleMapper) {
/**
* Flag to allow any name
*/
public val allowAnyName: Output?
get() = javaResource.allowAnyName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Flag to allow certificates matching the actual domain
*/
public val allowBareDomains: Output?
get() = javaResource.allowBareDomains().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Flag to allow names containing glob patterns.
*/
public val allowGlobDomains: Output?
get() = javaResource.allowGlobDomains().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Flag to allow IP SANs
*/
public val allowIpSans: Output?
get() = javaResource.allowIpSans().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Flag to allow certificates for localhost
*/
public val allowLocalhost: Output?
get() = javaResource.allowLocalhost().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Flag to allow certificates matching subdomains
*/
public val allowSubdomains: Output?
get() = javaResource.allowSubdomains().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Flag to allow wildcard certificates.
*/
public val allowWildcardCertificates: Output?
get() = javaResource.allowWildcardCertificates().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* List of allowed domains for certificates
*/
public val allowedDomains: Output>?
get() = javaResource.allowedDomains().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* Flag, if set, `allowed_domains` can be specified using identity template expressions such as `{{identity.entity.aliases..name}}`.
*/
public val allowedDomainsTemplate: Output?
get() = javaResource.allowedDomainsTemplate().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Defines allowed custom SANs
*/
public val allowedOtherSans: Output>?
get() = javaResource.allowedOtherSans().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* An array of allowed serial numbers to put in Subject
*/
public val allowedSerialNumbers: Output>?
get() = javaResource.allowedSerialNumbers().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* Defines allowed URI SANs
*/
public val allowedUriSans: Output>?
get() = javaResource.allowedUriSans().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* Flag, if set, `allowed_uri_sans` can be specified using identity template expressions such as `{{identity.entity.aliases..name}}`.
*/
public val allowedUriSansTemplate: Output
get() = javaResource.allowedUriSansTemplate().applyValue({ args0 -> args0 })
/**
* Defines allowed User IDs
*/
public val allowedUserIds: Output>?
get() = javaResource.allowedUserIds().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* The path the PKI secret backend is mounted at, with no leading or trailing `/`s.
*/
public val backend: Output
get() = javaResource.backend().applyValue({ args0 -> args0 })
/**
* Flag to mark basic constraints valid when issuing non-CA certificates
*/
public val basicConstraintsValidForNonCa: Output?
get() = javaResource.basicConstraintsValidForNonCa().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Flag to specify certificates for client use
*/
public val clientFlag: Output?
get() = javaResource.clientFlag().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Flag to specify certificates for code signing use
*/
public val codeSigningFlag: Output?
get() = javaResource.codeSigningFlag().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The country of generated certificates
*/
public val countries: Output>?
get() = javaResource.countries().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0
})
}).orElse(null)
})
/**
* Flag to specify certificates for email protection use
*/
public val emailProtectionFlag: Output?
get() = javaResource.emailProtectionFlag().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Flag to allow only valid host names
*/
public val enforceHostnames: Output?
get() = javaResource.enforceHostnames().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Specify the allowed extended key usage OIDs constraint on issued certificates
*/
public val extKeyUsageOids: Output>?
get() = javaResource.extKeyUsageOids().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* Specify the allowed extended key usage constraint on issued certificates
*/
public val extKeyUsages: Output>?
get() = javaResource.extKeyUsages().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0
})
}).orElse(null)
})
/**
* Flag to generate leases with certificates
*/
public val generateLease: Output?
get() = javaResource.generateLease().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Specifies the default issuer of this request. May
* be the value `default`, a name, or an issuer ID. Use ACLs to prevent access to
* the `/pki/issuer/:issuer_ref/{issue,sign}/:name` paths to prevent users
* overriding the role's `issuer_ref` value.
*/
public val issuerRef: Output
get() = javaResource.issuerRef().applyValue({ args0 -> args0 })
/**
* The number of bits of generated keys
*/
public val keyBits: Output?
get() = javaResource.keyBits().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The generated key type, choices: `rsa`, `ec`, `ed25519`, `any`
* Defaults to `rsa`
*/
public val keyType: Output?
get() = javaResource.keyType().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Specify the allowed key usage constraint on issued
* certificates. Defaults to `["DigitalSignature", "KeyAgreement", "KeyEncipherment"])`.
* To specify no default key usage constraints, set this to an empty list `[]`.
*/
public val keyUsages: Output>
get() = javaResource.keyUsages().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* The locality of generated certificates
*/
public val localities: Output>?
get() = javaResource.localities().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0
})
}).orElse(null)
})
/**
* The maximum lease TTL, in seconds, for the role.
*/
public val maxTtl: Output
get() = javaResource.maxTtl().applyValue({ args0 -> args0 })
/**
* The name to identify this role within the backend. Must be unique within the backend.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
public val namespace: Output?
get() = javaResource.namespace().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Flag to not store certificates in the storage backend
*/
public val noStore: Output?
get() = javaResource.noStore().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Specifies the duration by which to backdate the NotBefore property.
*/
public val notBeforeDuration: Output
get() = javaResource.notBeforeDuration().applyValue({ args0 -> args0 })
/**
* The organization unit of generated certificates
*/
public val organizationUnit: Output>?
get() = javaResource.organizationUnit().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* The organization of generated certificates
*/
public val organizations: Output>?
get() = javaResource.organizations().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* (Vault 1.11+ only) A block for specifying policy identifers. The `policy_identifier` block can be repeated, and supports the following arguments:
*/
public val policyIdentifier: Output>?
get() = javaResource.policyIdentifier().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0.let({ args0 -> toKotlin(args0) }) })
}).orElse(null)
})
/**
* Specify the list of allowed policies OIDs. Use with Vault 1.10 or before. For Vault 1.11+, use `policy_identifier` blocks instead
*/
public val policyIdentifiers: Output>?
get() = javaResource.policyIdentifiers().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* The postal code of generated certificates
*/
public val postalCodes: Output>?
get() = javaResource.postalCodes().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0
})
}).orElse(null)
})
/**
* The province of generated certificates
*/
public val provinces: Output>?
get() = javaResource.provinces().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0
})
}).orElse(null)
})
/**
* Flag to force CN usage
*/
public val requireCn: Output?
get() = javaResource.requireCn().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Flag to specify certificates for server use
*/
public val serverFlag: Output?
get() = javaResource.serverFlag().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The street address of generated certificates
*/
public val streetAddresses: Output>?
get() = javaResource.streetAddresses().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* The TTL, in seconds, for any certificate issued against this role.
*/
public val ttl: Output
get() = javaResource.ttl().applyValue({ args0 -> args0 })
/**
* Flag to use the CN in the CSR
*/
public val useCsrCommonName: Output?
get() = javaResource.useCsrCommonName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Flag to use the SANs in the CSR
*/
public val useCsrSans: Output?
get() = javaResource.useCsrSans().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
}
public object SecretBackendRoleMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.vault.pkiSecret.SecretBackendRole::class == javaResource::class
override fun map(javaResource: Resource): SecretBackendRole = SecretBackendRole(
javaResource as
com.pulumi.vault.pkiSecret.SecretBackendRole,
)
}
/**
* @see [SecretBackendRole].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [SecretBackendRole].
*/
public suspend fun secretBackendRole(
name: String,
block: suspend SecretBackendRoleResourceBuilder.() -> Unit,
): SecretBackendRole {
val builder = SecretBackendRoleResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [SecretBackendRole].
* @param name The _unique_ name of the resulting resource.
*/
public fun secretBackendRole(name: String): SecretBackendRole {
val builder = SecretBackendRoleResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy