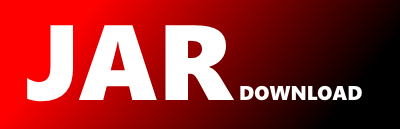
com.pulumi.vault.rabbitMq.kotlin.SecretBackendArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.rabbitMq.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.rabbitMq.SecretBackendArgs.builder
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const rabbitmq = new vault.rabbitmq.SecretBackend("rabbitmq", {
* connectionUri: "https://.....",
* username: "user",
* password: "password",
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* rabbitmq = vault.rabbit_mq.SecretBackend("rabbitmq",
* connection_uri="https://.....",
* username="user",
* password="password")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var rabbitmq = new Vault.RabbitMQ.SecretBackend("rabbitmq", new()
* {
* ConnectionUri = "https://.....",
* Username = "user",
* Password = "password",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/rabbitMq"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := rabbitMq.NewSecretBackend(ctx, "rabbitmq", &rabbitMq.SecretBackendArgs{
* ConnectionUri: pulumi.String("https://....."),
* Username: pulumi.String("user"),
* Password: pulumi.String("password"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.rabbitMq.SecretBackend;
* import com.pulumi.vault.rabbitMq.SecretBackendArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var rabbitmq = new SecretBackend("rabbitmq", SecretBackendArgs.builder()
* .connectionUri("https://.....")
* .username("user")
* .password("password")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* rabbitmq:
* type: vault:rabbitMq:SecretBackend
* properties:
* connectionUri: https://.....
* username: user
* password: password
* ```
*
* ## Import
* RabbitMQ secret backends can be imported using the `path`, e.g.
* ```sh
* $ pulumi import vault:rabbitMq/secretBackend:SecretBackend rabbitmq rabbitmq
* ```
* @property connectionUri Specifies the RabbitMQ connection URI.
* @property defaultLeaseTtlSeconds The default TTL for credentials
* issued by this backend.
* @property description A human-friendly description for this backend.
* @property disableRemount If set, opts out of mount migration on path updates.
* See here for more info on [Mount Migration](https://www.vaultproject.io/docs/concepts/mount-migration)
* @property maxLeaseTtlSeconds The maximum TTL that can be requested
* for credentials issued by this backend.
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property password Specifies the RabbitMQ management administrator password.
* @property passwordPolicy Specifies a password policy to use when creating dynamic credentials. Defaults to generating an alphanumeric password if not set.
* @property path The unique path this backend should be mounted at. Must
* not begin or end with a `/`. Defaults to `rabbitmq`.
* @property username Specifies the RabbitMQ management administrator username.
* @property usernameTemplate Template describing how dynamic usernames are generated.
* @property verifyConnection Specifies whether to verify connection URI, username, and password.
* Defaults to `true`.
*/
public data class SecretBackendArgs(
public val connectionUri: Output? = null,
public val defaultLeaseTtlSeconds: Output? = null,
public val description: Output? = null,
public val disableRemount: Output? = null,
public val maxLeaseTtlSeconds: Output? = null,
public val namespace: Output? = null,
public val password: Output? = null,
public val passwordPolicy: Output? = null,
public val path: Output? = null,
public val username: Output? = null,
public val usernameTemplate: Output? = null,
public val verifyConnection: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.rabbitMq.SecretBackendArgs =
com.pulumi.vault.rabbitMq.SecretBackendArgs.builder()
.connectionUri(connectionUri?.applyValue({ args0 -> args0 }))
.defaultLeaseTtlSeconds(defaultLeaseTtlSeconds?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.disableRemount(disableRemount?.applyValue({ args0 -> args0 }))
.maxLeaseTtlSeconds(maxLeaseTtlSeconds?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.password(password?.applyValue({ args0 -> args0 }))
.passwordPolicy(passwordPolicy?.applyValue({ args0 -> args0 }))
.path(path?.applyValue({ args0 -> args0 }))
.username(username?.applyValue({ args0 -> args0 }))
.usernameTemplate(usernameTemplate?.applyValue({ args0 -> args0 }))
.verifyConnection(verifyConnection?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SecretBackendArgs].
*/
@PulumiTagMarker
public class SecretBackendArgsBuilder internal constructor() {
private var connectionUri: Output? = null
private var defaultLeaseTtlSeconds: Output? = null
private var description: Output? = null
private var disableRemount: Output? = null
private var maxLeaseTtlSeconds: Output? = null
private var namespace: Output? = null
private var password: Output? = null
private var passwordPolicy: Output? = null
private var path: Output? = null
private var username: Output? = null
private var usernameTemplate: Output? = null
private var verifyConnection: Output? = null
/**
* @param value Specifies the RabbitMQ connection URI.
*/
@JvmName("pfxsshnrepuvhirj")
public suspend fun connectionUri(`value`: Output) {
this.connectionUri = value
}
/**
* @param value The default TTL for credentials
* issued by this backend.
*/
@JvmName("dgiiepuargkxtlse")
public suspend fun defaultLeaseTtlSeconds(`value`: Output) {
this.defaultLeaseTtlSeconds = value
}
/**
* @param value A human-friendly description for this backend.
*/
@JvmName("nkakucbaxrtdxhup")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value If set, opts out of mount migration on path updates.
* See here for more info on [Mount Migration](https://www.vaultproject.io/docs/concepts/mount-migration)
*/
@JvmName("tihnqdamdivnlvuf")
public suspend fun disableRemount(`value`: Output) {
this.disableRemount = value
}
/**
* @param value The maximum TTL that can be requested
* for credentials issued by this backend.
*/
@JvmName("pklblboudeocpmuw")
public suspend fun maxLeaseTtlSeconds(`value`: Output) {
this.maxLeaseTtlSeconds = value
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("rwsmdxtrdkuwmyex")
public suspend fun namespace(`value`: Output) {
this.namespace = value
}
/**
* @param value Specifies the RabbitMQ management administrator password.
*/
@JvmName("mnxxaeflmrpimtvn")
public suspend fun password(`value`: Output) {
this.password = value
}
/**
* @param value Specifies a password policy to use when creating dynamic credentials. Defaults to generating an alphanumeric password if not set.
*/
@JvmName("tyfsbrrhyirsactv")
public suspend fun passwordPolicy(`value`: Output) {
this.passwordPolicy = value
}
/**
* @param value The unique path this backend should be mounted at. Must
* not begin or end with a `/`. Defaults to `rabbitmq`.
*/
@JvmName("jsojdxjycqktdmrq")
public suspend fun path(`value`: Output) {
this.path = value
}
/**
* @param value Specifies the RabbitMQ management administrator username.
*/
@JvmName("vrqataqchranuasa")
public suspend fun username(`value`: Output) {
this.username = value
}
/**
* @param value Template describing how dynamic usernames are generated.
*/
@JvmName("dmmrmwmspctfurma")
public suspend fun usernameTemplate(`value`: Output) {
this.usernameTemplate = value
}
/**
* @param value Specifies whether to verify connection URI, username, and password.
* Defaults to `true`.
*/
@JvmName("wtmqgedoqysevwhn")
public suspend fun verifyConnection(`value`: Output) {
this.verifyConnection = value
}
/**
* @param value Specifies the RabbitMQ connection URI.
*/
@JvmName("kudsqmrgpnsyfwig")
public suspend fun connectionUri(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectionUri = mapped
}
/**
* @param value The default TTL for credentials
* issued by this backend.
*/
@JvmName("eoxmgumybliqtxmy")
public suspend fun defaultLeaseTtlSeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.defaultLeaseTtlSeconds = mapped
}
/**
* @param value A human-friendly description for this backend.
*/
@JvmName("vddncbwpvdrijdwk")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value If set, opts out of mount migration on path updates.
* See here for more info on [Mount Migration](https://www.vaultproject.io/docs/concepts/mount-migration)
*/
@JvmName("yfhdhthkjklxgsxj")
public suspend fun disableRemount(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.disableRemount = mapped
}
/**
* @param value The maximum TTL that can be requested
* for credentials issued by this backend.
*/
@JvmName("jarfuvajcvpckliv")
public suspend fun maxLeaseTtlSeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxLeaseTtlSeconds = mapped
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("kyvvnwenpkhywmsh")
public suspend fun namespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.namespace = mapped
}
/**
* @param value Specifies the RabbitMQ management administrator password.
*/
@JvmName("ldlxyexeigrcksph")
public suspend fun password(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.password = mapped
}
/**
* @param value Specifies a password policy to use when creating dynamic credentials. Defaults to generating an alphanumeric password if not set.
*/
@JvmName("wwubndqrpthncwqm")
public suspend fun passwordPolicy(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.passwordPolicy = mapped
}
/**
* @param value The unique path this backend should be mounted at. Must
* not begin or end with a `/`. Defaults to `rabbitmq`.
*/
@JvmName("wkjaypndcimyefyx")
public suspend fun path(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.path = mapped
}
/**
* @param value Specifies the RabbitMQ management administrator username.
*/
@JvmName("mqnujfohkjgricax")
public suspend fun username(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.username = mapped
}
/**
* @param value Template describing how dynamic usernames are generated.
*/
@JvmName("gwuyhdlygvmrbbff")
public suspend fun usernameTemplate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.usernameTemplate = mapped
}
/**
* @param value Specifies whether to verify connection URI, username, and password.
* Defaults to `true`.
*/
@JvmName("lmdgwiykwwbvtnpi")
public suspend fun verifyConnection(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.verifyConnection = mapped
}
internal fun build(): SecretBackendArgs = SecretBackendArgs(
connectionUri = connectionUri,
defaultLeaseTtlSeconds = defaultLeaseTtlSeconds,
description = description,
disableRemount = disableRemount,
maxLeaseTtlSeconds = maxLeaseTtlSeconds,
namespace = namespace,
password = password,
passwordPolicy = passwordPolicy,
path = path,
username = username,
usernameTemplate = usernameTemplate,
verifyConnection = verifyConnection,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy