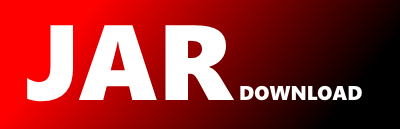
com.pulumi.vault.transform.kotlin.TransformFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.transform.kotlin
import com.pulumi.vault.transform.TransformFunctions.getDecodePlain
import com.pulumi.vault.transform.TransformFunctions.getEncodePlain
import com.pulumi.vault.transform.kotlin.inputs.GetDecodePlainArgs
import com.pulumi.vault.transform.kotlin.inputs.GetDecodePlainArgsBuilder
import com.pulumi.vault.transform.kotlin.inputs.GetEncodePlainArgs
import com.pulumi.vault.transform.kotlin.inputs.GetEncodePlainArgsBuilder
import com.pulumi.vault.transform.kotlin.outputs.GetDecodeResult
import com.pulumi.vault.transform.kotlin.outputs.GetEncodeResult
import kotlinx.coroutines.future.await
import kotlin.Any
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.vault.transform.kotlin.outputs.GetDecodeResult.Companion.toKotlin as getDecodeResultToKotlin
import com.pulumi.vault.transform.kotlin.outputs.GetEncodeResult.Companion.toKotlin as getEncodeResultToKotlin
public object TransformFunctions {
/**
* This data source supports the "/transform/decode/{role_name}" Vault endpoint.
* It decodes the provided value using a named role.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const transform = new vault.Mount("transform", {
* path: "transform",
* type: "transform",
* });
* const ccn_fpe = new vault.transform.Transformation("ccn-fpe", {
* path: transform.path,
* name: "ccn-fpe",
* type: "fpe",
* template: "builtin/creditcardnumber",
* tweakSource: "internal",
* allowedRoles: ["payments"],
* });
* const payments = new vault.transform.Role("payments", {
* path: ccn_fpe.path,
* name: "payments",
* transformations: ["ccn-fpe"],
* });
* const test = vault.transform.getDecodeOutput({
* path: payments.path,
* roleName: "payments",
* value: "9300-3376-4943-8903",
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* transform = vault.Mount("transform",
* path="transform",
* type="transform")
* ccn_fpe = vault.transform.Transformation("ccn-fpe",
* path=transform.path,
* name="ccn-fpe",
* type="fpe",
* template="builtin/creditcardnumber",
* tweak_source="internal",
* allowed_roles=["payments"])
* payments = vault.transform.Role("payments",
* path=ccn_fpe.path,
* name="payments",
* transformations=["ccn-fpe"])
* test = vault.transform.get_decode_output(path=payments.path,
* role_name="payments",
* value="9300-3376-4943-8903")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var transform = new Vault.Mount("transform", new()
* {
* Path = "transform",
* Type = "transform",
* });
* var ccn_fpe = new Vault.Transform.Transformation("ccn-fpe", new()
* {
* Path = transform.Path,
* Name = "ccn-fpe",
* Type = "fpe",
* Template = "builtin/creditcardnumber",
* TweakSource = "internal",
* AllowedRoles = new[]
* {
* "payments",
* },
* });
* var payments = new Vault.Transform.Role("payments", new()
* {
* Path = ccn_fpe.Path,
* Name = "payments",
* Transformations = new[]
* {
* "ccn-fpe",
* },
* });
* var test = Vault.Transform.GetDecode.Invoke(new()
* {
* Path = payments.Path,
* RoleName = "payments",
* Value = "9300-3376-4943-8903",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/transform"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* transform, err := vault.NewMount(ctx, "transform", &vault.MountArgs{
* Path: pulumi.String("transform"),
* Type: pulumi.String("transform"),
* })
* if err != nil {
* return err
* }
* _, err = transform.NewTransformation(ctx, "ccn-fpe", &transform.TransformationArgs{
* Path: transform.Path,
* Name: pulumi.String("ccn-fpe"),
* Type: pulumi.String("fpe"),
* Template: pulumi.String("builtin/creditcardnumber"),
* TweakSource: pulumi.String("internal"),
* AllowedRoles: pulumi.StringArray{
* pulumi.String("payments"),
* },
* })
* if err != nil {
* return err
* }
* payments, err := transform.NewRole(ctx, "payments", &transform.RoleArgs{
* Path: ccn_fpe.Path,
* Name: pulumi.String("payments"),
* Transformations: pulumi.StringArray{
* pulumi.String("ccn-fpe"),
* },
* })
* if err != nil {
* return err
* }
* _ = transform.GetDecodeOutput(ctx, transform.GetDecodeOutputArgs{
* Path: payments.Path,
* RoleName: pulumi.String("payments"),
* Value: pulumi.String("9300-3376-4943-8903"),
* }, nil)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.Mount;
* import com.pulumi.vault.MountArgs;
* import com.pulumi.vault.transform.Transformation;
* import com.pulumi.vault.transform.TransformationArgs;
* import com.pulumi.vault.transform.Role;
* import com.pulumi.vault.transform.RoleArgs;
* import com.pulumi.vault.transform.TransformFunctions;
* import com.pulumi.vault.transform.inputs.GetDecodeArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var transform = new Mount("transform", MountArgs.builder()
* .path("transform")
* .type("transform")
* .build());
* var ccn_fpe = new Transformation("ccn-fpe", TransformationArgs.builder()
* .path(transform.path())
* .name("ccn-fpe")
* .type("fpe")
* .template("builtin/creditcardnumber")
* .tweakSource("internal")
* .allowedRoles("payments")
* .build());
* var payments = new Role("payments", RoleArgs.builder()
* .path(ccn_fpe.path())
* .name("payments")
* .transformations("ccn-fpe")
* .build());
* final var test = TransformFunctions.getDecode(GetDecodeArgs.builder()
* .path(payments.path())
* .roleName("payments")
* .value("9300-3376-4943-8903")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* transform:
* type: vault:Mount
* properties:
* path: transform
* type: transform
* ccn-fpe:
* type: vault:transform:Transformation
* properties:
* path: ${transform.path}
* name: ccn-fpe
* type: fpe
* template: builtin/creditcardnumber
* tweakSource: internal
* allowedRoles:
* - payments
* payments:
* type: vault:transform:Role
* properties:
* path: ${["ccn-fpe"].path}
* name: payments
* transformations:
* - ccn-fpe
* variables:
* test:
* fn::invoke:
* Function: vault:transform:getDecode
* Arguments:
* path: ${payments.path}
* roleName: payments
* value: 9300-3376-4943-8903
* ```
*
* @param argument A collection of arguments for invoking getDecode.
* @return A collection of values returned by getDecode.
*/
public suspend fun getDecode(argument: GetDecodePlainArgs): GetDecodeResult =
getDecodeResultToKotlin(getDecodePlain(argument.toJava()).await())
/**
* @see [getDecode].
* @param batchInputs Specifies a list of items to be decoded in a single batch. If this parameter is set, the top-level parameters 'value', 'transformation' and 'tweak' will be ignored. Each batch item within the list can specify these parameters instead.
* @param batchResults The result of decoding a batch.
* @param decodedValue The result of decoding a value.
* @param namespace The namespace of the target resource.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @param path Path to where the back-end is mounted within Vault.
* @param roleName The name of the role.
* @param transformation The transformation to perform. If no value is provided and the role contains a single transformation, this value will be inferred from the role.
* @param tweak The tweak value to use. Only applicable for FPE transformations
* @param value The value in which to decode.
* @return A collection of values returned by getDecode.
*/
public suspend fun getDecode(
batchInputs: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy