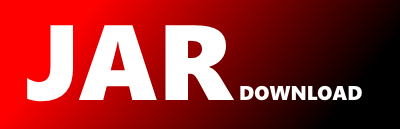
com.pulumi.vault.transform.kotlin.TransformationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.transform.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.transform.TransformationArgs.builder
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property allowedRoles The set of roles allowed to perform this transformation.
* @property deletionAllowed If true, this transform can be deleted.
* Otherwise, deletion is blocked while this value remains false. Default: `false`
* *Only supported on vault-1.12+*
* @property maskingCharacter The character used to replace data when in masking mode
* @property name The name of the transformation.
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property path Path to where the back-end is mounted within Vault.
* @property template The name of the template to use.
* @property templates Templates configured for transformation.
* @property tweakSource The source of where the tweak value comes from. Only valid when in FPE mode.
* @property type The type of transformation to perform.
*/
public data class TransformationArgs(
public val allowedRoles: Output>? = null,
public val deletionAllowed: Output? = null,
public val maskingCharacter: Output? = null,
public val name: Output? = null,
public val namespace: Output? = null,
public val path: Output? = null,
public val template: Output? = null,
public val templates: Output>? = null,
public val tweakSource: Output? = null,
public val type: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.transform.TransformationArgs =
com.pulumi.vault.transform.TransformationArgs.builder()
.allowedRoles(allowedRoles?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.deletionAllowed(deletionAllowed?.applyValue({ args0 -> args0 }))
.maskingCharacter(maskingCharacter?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.path(path?.applyValue({ args0 -> args0 }))
.template(template?.applyValue({ args0 -> args0 }))
.templates(templates?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.tweakSource(tweakSource?.applyValue({ args0 -> args0 }))
.type(type?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [TransformationArgs].
*/
@PulumiTagMarker
public class TransformationArgsBuilder internal constructor() {
private var allowedRoles: Output>? = null
private var deletionAllowed: Output? = null
private var maskingCharacter: Output? = null
private var name: Output? = null
private var namespace: Output? = null
private var path: Output? = null
private var template: Output? = null
private var templates: Output>? = null
private var tweakSource: Output? = null
private var type: Output? = null
/**
* @param value The set of roles allowed to perform this transformation.
*/
@JvmName("txhungugawwmdkcx")
public suspend fun allowedRoles(`value`: Output>) {
this.allowedRoles = value
}
@JvmName("hyglfscwcpuytehv")
public suspend fun allowedRoles(vararg values: Output) {
this.allowedRoles = Output.all(values.asList())
}
/**
* @param values The set of roles allowed to perform this transformation.
*/
@JvmName("ssdslmhoyqnihsdw")
public suspend fun allowedRoles(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy