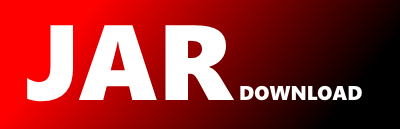
com.pulumi.vault.transit.kotlin.SecretBackendKeyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.transit.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.vault.transit.SecretBackendKeyArgs.builder
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Creates an Encryption Keyring on a Transit Secret Backend for Vault.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const transit = new vault.Mount("transit", {
* path: "transit",
* type: "transit",
* description: "Example description",
* defaultLeaseTtlSeconds: 3600,
* maxLeaseTtlSeconds: 86400,
* });
* const key = new vault.transit.SecretBackendKey("key", {
* backend: transit.path,
* name: "my_key",
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* transit = vault.Mount("transit",
* path="transit",
* type="transit",
* description="Example description",
* default_lease_ttl_seconds=3600,
* max_lease_ttl_seconds=86400)
* key = vault.transit.SecretBackendKey("key",
* backend=transit.path,
* name="my_key")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var transit = new Vault.Mount("transit", new()
* {
* Path = "transit",
* Type = "transit",
* Description = "Example description",
* DefaultLeaseTtlSeconds = 3600,
* MaxLeaseTtlSeconds = 86400,
* });
* var key = new Vault.Transit.SecretBackendKey("key", new()
* {
* Backend = transit.Path,
* Name = "my_key",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/transit"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* transit, err := vault.NewMount(ctx, "transit", &vault.MountArgs{
* Path: pulumi.String("transit"),
* Type: pulumi.String("transit"),
* Description: pulumi.String("Example description"),
* DefaultLeaseTtlSeconds: pulumi.Int(3600),
* MaxLeaseTtlSeconds: pulumi.Int(86400),
* })
* if err != nil {
* return err
* }
* _, err = transit.NewSecretBackendKey(ctx, "key", &transit.SecretBackendKeyArgs{
* Backend: transit.Path,
* Name: pulumi.String("my_key"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.Mount;
* import com.pulumi.vault.MountArgs;
* import com.pulumi.vault.transit.SecretBackendKey;
* import com.pulumi.vault.transit.SecretBackendKeyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var transit = new Mount("transit", MountArgs.builder()
* .path("transit")
* .type("transit")
* .description("Example description")
* .defaultLeaseTtlSeconds(3600)
* .maxLeaseTtlSeconds(86400)
* .build());
* var key = new SecretBackendKey("key", SecretBackendKeyArgs.builder()
* .backend(transit.path())
* .name("my_key")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* transit:
* type: vault:Mount
* properties:
* path: transit
* type: transit
* description: Example description
* defaultLeaseTtlSeconds: 3600
* maxLeaseTtlSeconds: 86400
* key:
* type: vault:transit:SecretBackendKey
* properties:
* backend: ${transit.path}
* name: my_key
* ```
*
* ## Import
* Transit secret backend keys can be imported using the `path`, e.g.
* ```sh
* $ pulumi import vault:transit/secretBackendKey:SecretBackendKey key transit/keys/my_key
* ```
* @property allowPlaintextBackup Enables taking backup of entire keyring in the plaintext format. Once set, this cannot be disabled.
* * Refer to Vault API documentation on key backups for more information: [Backup Key](https://www.vaultproject.io/api-docs/secret/transit#backup-key)
* @property autoRotatePeriod Amount of seconds the key should live before being automatically rotated.
* A value of 0 disables automatic rotation for the key.
* @property backend The path the transit secret backend is mounted at, with no leading or trailing `/`s.
* @property convergentEncryption Whether or not to support convergent encryption, where the same plaintext creates the same ciphertext. This requires `derived` to be set to `true`.
* @property deletionAllowed Specifies if the key is allowed to be deleted.
* @property derived Specifies if key derivation is to be used. If enabled, all encrypt/decrypt requests to this key must provide a context which is used for key derivation.
* @property exportable Enables keys to be exportable. This allows for all valid private keys in the keyring to be exported. Once set, this cannot be disabled.
* @property keySize The key size in bytes for algorithms that allow variable key sizes. Currently only applicable to HMAC, where it must be between 32 and 512 bytes.
* @property minDecryptionVersion Minimum key version to use for decryption.
* @property minEncryptionVersion Minimum key version to use for encryption
* @property name The name to identify this key within the backend. Must be unique within the backend.
* @property namespace The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
* @property type Specifies the type of key to create. The currently-supported types are: `aes128-gcm96`, `aes256-gcm96` (default), `chacha20-poly1305`, `ed25519`, `ecdsa-p256`, `ecdsa-p384`, `ecdsa-p521`, `hmac`, `rsa-2048`, `rsa-3072` and `rsa-4096`.
* * Refer to the Vault documentation on transit key types for more information: [Key Types](https://www.vaultproject.io/docs/secrets/transit#key-types)
*/
public data class SecretBackendKeyArgs(
public val allowPlaintextBackup: Output? = null,
public val autoRotatePeriod: Output? = null,
public val backend: Output? = null,
public val convergentEncryption: Output? = null,
public val deletionAllowed: Output? = null,
public val derived: Output? = null,
public val exportable: Output? = null,
public val keySize: Output? = null,
public val minDecryptionVersion: Output? = null,
public val minEncryptionVersion: Output? = null,
public val name: Output? = null,
public val namespace: Output? = null,
public val type: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.vault.transit.SecretBackendKeyArgs =
com.pulumi.vault.transit.SecretBackendKeyArgs.builder()
.allowPlaintextBackup(allowPlaintextBackup?.applyValue({ args0 -> args0 }))
.autoRotatePeriod(autoRotatePeriod?.applyValue({ args0 -> args0 }))
.backend(backend?.applyValue({ args0 -> args0 }))
.convergentEncryption(convergentEncryption?.applyValue({ args0 -> args0 }))
.deletionAllowed(deletionAllowed?.applyValue({ args0 -> args0 }))
.derived(derived?.applyValue({ args0 -> args0 }))
.exportable(exportable?.applyValue({ args0 -> args0 }))
.keySize(keySize?.applyValue({ args0 -> args0 }))
.minDecryptionVersion(minDecryptionVersion?.applyValue({ args0 -> args0 }))
.minEncryptionVersion(minEncryptionVersion?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.type(type?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SecretBackendKeyArgs].
*/
@PulumiTagMarker
public class SecretBackendKeyArgsBuilder internal constructor() {
private var allowPlaintextBackup: Output? = null
private var autoRotatePeriod: Output? = null
private var backend: Output? = null
private var convergentEncryption: Output? = null
private var deletionAllowed: Output? = null
private var derived: Output? = null
private var exportable: Output? = null
private var keySize: Output? = null
private var minDecryptionVersion: Output? = null
private var minEncryptionVersion: Output? = null
private var name: Output? = null
private var namespace: Output? = null
private var type: Output? = null
/**
* @param value Enables taking backup of entire keyring in the plaintext format. Once set, this cannot be disabled.
* * Refer to Vault API documentation on key backups for more information: [Backup Key](https://www.vaultproject.io/api-docs/secret/transit#backup-key)
*/
@JvmName("ybsqwyfcscfpvwaj")
public suspend fun allowPlaintextBackup(`value`: Output) {
this.allowPlaintextBackup = value
}
/**
* @param value Amount of seconds the key should live before being automatically rotated.
* A value of 0 disables automatic rotation for the key.
*/
@JvmName("knjwkogffikusbon")
public suspend fun autoRotatePeriod(`value`: Output) {
this.autoRotatePeriod = value
}
/**
* @param value The path the transit secret backend is mounted at, with no leading or trailing `/`s.
*/
@JvmName("oqtwihewjabqqdpa")
public suspend fun backend(`value`: Output) {
this.backend = value
}
/**
* @param value Whether or not to support convergent encryption, where the same plaintext creates the same ciphertext. This requires `derived` to be set to `true`.
*/
@JvmName("hjocithqugnmlnhn")
public suspend fun convergentEncryption(`value`: Output) {
this.convergentEncryption = value
}
/**
* @param value Specifies if the key is allowed to be deleted.
*/
@JvmName("tvvjbvwmpffnkwes")
public suspend fun deletionAllowed(`value`: Output) {
this.deletionAllowed = value
}
/**
* @param value Specifies if key derivation is to be used. If enabled, all encrypt/decrypt requests to this key must provide a context which is used for key derivation.
*/
@JvmName("xdnaiqggqcoxtxpg")
public suspend fun derived(`value`: Output) {
this.derived = value
}
/**
* @param value Enables keys to be exportable. This allows for all valid private keys in the keyring to be exported. Once set, this cannot be disabled.
*/
@JvmName("vaajattuvhigyqmg")
public suspend fun exportable(`value`: Output) {
this.exportable = value
}
/**
* @param value The key size in bytes for algorithms that allow variable key sizes. Currently only applicable to HMAC, where it must be between 32 and 512 bytes.
*/
@JvmName("rfvoymglwprqqxpm")
public suspend fun keySize(`value`: Output) {
this.keySize = value
}
/**
* @param value Minimum key version to use for decryption.
*/
@JvmName("gjlhbtrbpciuljcg")
public suspend fun minDecryptionVersion(`value`: Output) {
this.minDecryptionVersion = value
}
/**
* @param value Minimum key version to use for encryption
*/
@JvmName("hhsuiblcvonqbikq")
public suspend fun minEncryptionVersion(`value`: Output) {
this.minEncryptionVersion = value
}
/**
* @param value The name to identify this key within the backend. Must be unique within the backend.
*/
@JvmName("bkmetosjgxhhxush")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("vclmawelfojxrqee")
public suspend fun namespace(`value`: Output) {
this.namespace = value
}
/**
* @param value Specifies the type of key to create. The currently-supported types are: `aes128-gcm96`, `aes256-gcm96` (default), `chacha20-poly1305`, `ed25519`, `ecdsa-p256`, `ecdsa-p384`, `ecdsa-p521`, `hmac`, `rsa-2048`, `rsa-3072` and `rsa-4096`.
* * Refer to the Vault documentation on transit key types for more information: [Key Types](https://www.vaultproject.io/docs/secrets/transit#key-types)
*/
@JvmName("dsdbtpgafvdthvdn")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value Enables taking backup of entire keyring in the plaintext format. Once set, this cannot be disabled.
* * Refer to Vault API documentation on key backups for more information: [Backup Key](https://www.vaultproject.io/api-docs/secret/transit#backup-key)
*/
@JvmName("nusyinijmjjmtrgy")
public suspend fun allowPlaintextBackup(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.allowPlaintextBackup = mapped
}
/**
* @param value Amount of seconds the key should live before being automatically rotated.
* A value of 0 disables automatic rotation for the key.
*/
@JvmName("watcnubqsyrrvxoy")
public suspend fun autoRotatePeriod(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.autoRotatePeriod = mapped
}
/**
* @param value The path the transit secret backend is mounted at, with no leading or trailing `/`s.
*/
@JvmName("pkuvfsysipjdkjoi")
public suspend fun backend(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.backend = mapped
}
/**
* @param value Whether or not to support convergent encryption, where the same plaintext creates the same ciphertext. This requires `derived` to be set to `true`.
*/
@JvmName("fhydgjowwjnxkgbq")
public suspend fun convergentEncryption(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.convergentEncryption = mapped
}
/**
* @param value Specifies if the key is allowed to be deleted.
*/
@JvmName("xrnbbpqmoshrlgmr")
public suspend fun deletionAllowed(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deletionAllowed = mapped
}
/**
* @param value Specifies if key derivation is to be used. If enabled, all encrypt/decrypt requests to this key must provide a context which is used for key derivation.
*/
@JvmName("hdmqcnqgmngwfvol")
public suspend fun derived(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.derived = mapped
}
/**
* @param value Enables keys to be exportable. This allows for all valid private keys in the keyring to be exported. Once set, this cannot be disabled.
*/
@JvmName("sxeyplyqsnwuybic")
public suspend fun exportable(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.exportable = mapped
}
/**
* @param value The key size in bytes for algorithms that allow variable key sizes. Currently only applicable to HMAC, where it must be between 32 and 512 bytes.
*/
@JvmName("shbmfooonydjmhol")
public suspend fun keySize(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.keySize = mapped
}
/**
* @param value Minimum key version to use for decryption.
*/
@JvmName("bxhggosoxoqambae")
public suspend fun minDecryptionVersion(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minDecryptionVersion = mapped
}
/**
* @param value Minimum key version to use for encryption
*/
@JvmName("lgsyqmrgnyktyddb")
public suspend fun minEncryptionVersion(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minEncryptionVersion = mapped
}
/**
* @param value The name to identify this key within the backend. Must be unique within the backend.
*/
@JvmName("rkwbqqpjnbpjixet")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
@JvmName("eflqrohxtablmrdl")
public suspend fun namespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.namespace = mapped
}
/**
* @param value Specifies the type of key to create. The currently-supported types are: `aes128-gcm96`, `aes256-gcm96` (default), `chacha20-poly1305`, `ed25519`, `ecdsa-p256`, `ecdsa-p384`, `ecdsa-p521`, `hmac`, `rsa-2048`, `rsa-3072` and `rsa-4096`.
* * Refer to the Vault documentation on transit key types for more information: [Key Types](https://www.vaultproject.io/docs/secrets/transit#key-types)
*/
@JvmName("bcjudfispbdasbpi")
public suspend fun type(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.type = mapped
}
internal fun build(): SecretBackendKeyArgs = SecretBackendKeyArgs(
allowPlaintextBackup = allowPlaintextBackup,
autoRotatePeriod = autoRotatePeriod,
backend = backend,
convergentEncryption = convergentEncryption,
deletionAllowed = deletionAllowed,
derived = derived,
exportable = exportable,
keySize = keySize,
minDecryptionVersion = minDecryptionVersion,
minEncryptionVersion = minEncryptionVersion,
name = name,
namespace = namespace,
type = type,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy