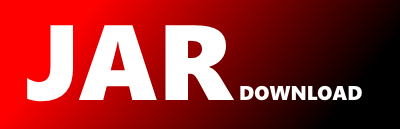
vite.api.service.ViteWebSocketDemo Maven / Gradle / Ivy
The newest version!
package vite.api.service;
import com.alibaba.fastjson.JSONObject;
import com.alibaba.fastjson.TypeReference;
import org.java_websocket.client.WebSocketClient;
import org.java_websocket.handshake.ServerHandshake;
import vite.api.vo.RequestJson;
import vite.api.vo.SnapshotBlock;
import vite.api.vo.SnapshotBlockSubscriptions;
import vite.api.vo.SubscribeCallback;
import java.net.URI;
import java.net.URISyntaxException;
import java.nio.ByteBuffer;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.UUID;
public class ViteWebSocketDemo extends ViteWebSocket {
public ViteWebSocketDemo(String url) throws URISyntaxException {
super(url);
// 连接地址
}
@Override
public void onOpen(ServerHandshake shake) {
System.out.println("客户端连接了服务器");
}
@Override
public void onMessage(String paramString) {
System.out.println(paramString);
JSONObject jsonObject = JSONObject.parseObject(paramString);
if (jsonObject != null && jsonObject.getString("id") != null) {
if (jsonObject.getString("id").startsWith("accountBlock")) {
} else if (jsonObject.getString("id").startsWith("snapshotBlock")) {
SnapshotBlock snapshotBlock = jsonObject.getJSONObject("result").toJavaObject(SnapshotBlock.class);
Map map = snapshotBlock.getSnapshotContent();
if (map != null) {
for (Map.Entry entry : map.entrySet()) {
String address = entry.getKey();
SnapshotBlock.Item item = entry.getValue();
String hash = item.getHash();
long height = item.getHeight();
RequestJson requestJson = new RequestJson();
requestJson.setMethod("ledger_getBlocksByHash");
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy