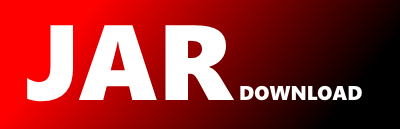
org.voovan.docker.message.container.ContainerCreate Maven / Gradle / Ivy
Show all versions of JDocker Show documentation
package org.voovan.docker.message.container;
import org.voovan.docker.message.container.atom.Config;
import org.voovan.docker.message.container.atom.Healthcheck;
import org.voovan.docker.message.container.atom.HostConfig;
import org.voovan.tools.TObject;
import org.voovan.tools.json.JSON;
import org.voovan.tools.log.Logger;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
*
* @author helyho
*
* DockerFly Framework.
* WebSite: https://git.oschina.net/helyho/JDocker
* Licence: Apache v2 License
*/
public class ContainerCreate extends Config {
private HostConfig hostConfig;
private Boolean networkDisabled;
private String shell;
//1.25
private Integer stopTimeout;
public ContainerCreate() {
this.setAttachStdin(false);
this.setAttachStdout(true);
this.setAttachStderr(true);
this.setTty(false);
this.setOpenStdin(false);
this.setStdinOnce(false);
this.setCmd(new ArrayList());
this.setEnv(new ArrayList());
this.setLabels(new HashMap());
this.setExposedPorts(new HashMap>());
this.setHostConfig(new HostConfig());
this.setHealthcheck(new Healthcheck());
this.setEntrypoint(new ArrayList());
this.setOnBuild(new ArrayList());
this.setStopSignal("SIGTERM");
}
public HostConfig getHostConfig() {
return hostConfig;
}
public void setHostConfig(HostConfig hostConfig) {
this.hostConfig = hostConfig;
}
public Boolean getNetworkDisabled() {
return networkDisabled;
}
public void setNetworkDisabled(Boolean networkDisabled) {
this.networkDisabled = networkDisabled;
}
public String getShell() {
return shell;
}
public void setShell(String shell) {
this.shell = shell;
}
//v1.25
public Integer getStopTimeout() {
return stopTimeout;
}
//v1.25
public void setStopTimeout(Integer stopTimeout) {
this.stopTimeout = stopTimeout;
}
/**
* 增加端口绑定
*
* @param containerPort 容器端口
* @param protocol 协议
* @param hostPortStrs 主机端口 127.0.0.1:3000
*/
public void addPortBind(int containerPort, String protocol, String... hostPortStrs) {
String containerPortAndProtcol = containerPort + "/" + protocol;
//端口绑定需要设置两个节点,并且要对应
//1.设置 ExposedPorts 节点
this.getExposedPorts().put(containerPortAndProtcol, new HashMap());
//2.设置HostConfig.PortBindings 节点
List