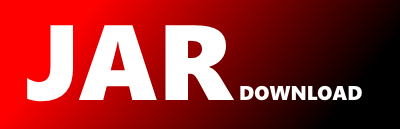
org.vx68k.bitbucket.api.client.ClientUtilities Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bitbucket-api-client Show documentation
Show all versions of bitbucket-api-client Show documentation
This project builds a client API implementation for Bitbucket
Cloud.
/*
* ClientUtilities
* Copyright (C) 2015 Nishimura Software Studio
*
* This program is free software: you can redistribute it and/or modify it
* under the terms of the GNU Affero General Public License as published by the
* Free Software Foundation, either version 3 of the License, or (at your
* option) any later version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Affero General Public License
* for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.vx68k.bitbucket.api.client;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.HashMap;
import java.util.Map;
import java.util.UUID;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.json.JsonObject;
import javax.json.JsonValue;
/**
* Collection of static utility methods.
* @author Kaz Nishimura
* @since 4.0
*/
public class ClientUtilities {
private static final String PACKAGE_NAME =
ClientUtilities.class.getPackage().getName();
private ClientUtilities() {
}
/**
* Returns a logger.
* @return logger for this package
*/
public static Logger getLogger() {
return Logger.getLogger(
PACKAGE_NAME, PACKAGE_NAME + ".resources.LogMessages");
}
/**
* Parses a string into a UUID.
* The string representation of a UUID may be enclosed in braces.
* @param string string that represents a UUID
* @return {@link UUID} object, or null
if the string is
* null
* @throws IllegalArgumentException if the string could not parsed as a
* UUID.
*/
public static UUID parseUUID(String string) {
if (string == null) {
return null;
}
if (string.startsWith("{") && string.endsWith("}")) {
string = string.substring(1, string.length() - 1);
}
return UUID.fromString(string);
}
/**
* Parses a JSON object into a map of links.
* @param jsonObject JSON object
* @return map of links
*/
public static Map parseLinks(JsonObject jsonObject) {
Map links = new HashMap();
for (Map.Entry entry : jsonObject.entrySet()) {
try {
URL link = parseLink((JsonObject) entry.getValue());
links.put(entry.getKey(), link);
} catch (MalformedURLException exception) {
getLogger().log(
Level.WARNING, "Could not parse a URL value",
exception);
}
}
return links;
}
/**
* Parses a JSON object into a URL.
* @param jsonObject JSON object
* @return link
* @throws MalformedURLException if the href
value could not
* be parsed as a URL.
*/
protected static URL parseLink(JsonObject jsonObject)
throws MalformedURLException {
return parseURL(jsonObject.getString(JsonKeys.HREF));
}
/**
* Parses a string into a URL.
* @param string string that represents a URL
* @return parsed {@link URL} object, or null
if the string
* is null
* @throws MalformedURLException if the string could not parsed as a URL.
*/
public static URL parseURL(String string) throws MalformedURLException {
if (string == null) {
return null;
}
return new URL(string);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy