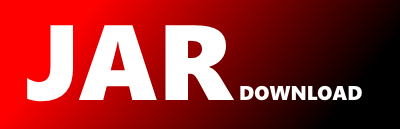
org.walkmod.javalang.ast.stmt.ForStmt Maven / Gradle / Ivy
/*
Copyright (C) 2013 Raquel Pau and Albert Coroleu.
Walkmod is free software: you can redistribute it and/or modify
it under the terms of the GNU Lesser General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
Walkmod is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public License
along with Walkmod. If not, see .*/
package org.walkmod.javalang.ast.stmt;
import java.util.LinkedList;
import java.util.List;
import org.walkmod.javalang.ast.Node;
import org.walkmod.javalang.ast.expr.Expression;
import org.walkmod.javalang.visitors.GenericVisitor;
import org.walkmod.javalang.visitors.VoidVisitor;
/**
* @author Julio Vilmar Gesser
*/
public final class ForStmt extends Statement {
private List init;
private Expression compare;
private List update;
private Statement body;
public ForStmt() {
}
public ForStmt(List init, Expression compare, List update, Statement body) {
setCompare(compare);
setInit(init);
setUpdate(update);
setBody(body);
}
public ForStmt(int beginLine, int beginColumn, int endLine, int endColumn, List init, Expression compare,
List update, Statement body) {
super(beginLine, beginColumn, endLine, endColumn);
setCompare(compare);
setInit(init);
setUpdate(update);
setBody(body);
}
@Override
public boolean removeChild(Node child) {
boolean result = false;
if (child != null) {
if (compare == child) {
compare = null;
result = true;
}
if (!result) {
if (init != null) {
if (child instanceof Expression) {
List initAux = new LinkedList(init);
result = initAux.remove(child);
init = initAux;
}
}
}
if (!result) {
if (update != null) {
if (child instanceof Expression) {
List updateAux = new LinkedList(update);
result = updateAux.remove(child);
update = updateAux;
}
}
}
if (!result) {
if (body == child) {
body = null;
result = true;
}
}
}
if(result){
updateReferences(child);
}
return result;
}
@Override
public List getChildren() {
List children = super.getChildren();
if (init != null) {
children.addAll(init);
}
if (compare != null) {
children.add(compare);
}
if (update != null) {
children.addAll(update);
}
if (body != null) {
children.add(body);
}
return children;
}
@Override
public R accept(GenericVisitor v, A arg) {
if (!check()) {
return null;
}
return v.visit(this, arg);
}
@Override
public void accept(VoidVisitor v, A arg) {
if (check()) {
v.visit(this, arg);
}
}
public Statement getBody() {
return body;
}
public Expression getCompare() {
return compare;
}
public List getInit() {
return init;
}
public List getUpdate() {
return update;
}
public void setBody(Statement body) {
if (this.body != null) {
updateReferences(this.body);
}
this.body = body;
setAsParentNodeOf(body);
}
public void setCompare(Expression compare) {
if (this.compare != null) {
updateReferences(this.compare);
}
this.compare = compare;
setAsParentNodeOf(compare);
}
public void setInit(List init) {
this.init = init;
setAsParentNodeOf(init);
}
public void setUpdate(List update) {
this.update = update;
setAsParentNodeOf(update);
}
@Override
public boolean replaceChildNode(Node oldChild, Node newChild) {
boolean updated = false;
if (oldChild == compare) {
setCompare((Expression) newChild);
updated = true;
}
if (!updated) {
if (oldChild == body) {
setBody((Statement) newChild);
updated = true;
}
if (!updated) {
updated = replaceChildNodeInList(oldChild, newChild, init);
if (!updated) {
updated = replaceChildNodeInList(oldChild, newChild, update);
}
}
}
return updated;
}
@Override
public ForStmt clone() throws CloneNotSupportedException {
return new ForStmt(clone(init), clone(compare), clone(update), clone(body));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy