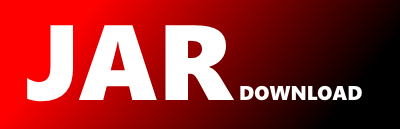
org.walkmod.Options Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of walkmod-core Show documentation
Show all versions of walkmod-core Show documentation
Open source project to apply coding conventions. This is the core component
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy