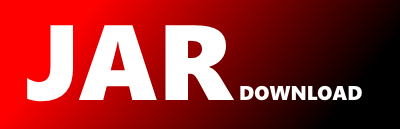
org.webpieces.templating.impl.ProdTemplateService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of http-templating Show documentation
Show all versions of http-templating Show documentation
Templating library using groovy as the scripting language
package org.webpieces.templating.impl;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import javax.inject.Inject;
import javax.inject.Singleton;
import org.webpieces.ctx.api.extension.HtmlTagCreator;
import org.webpieces.templating.api.HtmlTagLookup;
import org.webpieces.templating.api.RouterLookup;
import org.webpieces.templating.api.Template;
import org.webpieces.templating.api.TemplateResult;
import org.webpieces.templating.api.TemplateService;
import org.webpieces.templating.api.TemplateUtil;
import org.webpieces.util.exceptions.SneakyThrow;
@Singleton
public class ProdTemplateService extends AbstractTemplateService implements TemplateService {
protected HtmlTagLookup lookup;
private boolean isInitialized = false;
protected RouterLookup urlLookup;
@Inject
public ProdTemplateService(RouterLookup urlLookup, HtmlTagLookup lookup) {
this.urlLookup = urlLookup;
this.lookup = lookup;
}
@Override
public void loadAndRunTemplateImpl(String templatePath, StringWriter out, Map pageArgs) {
Template template = loadTemplate(templatePath);
runTemplate(template, out, pageArgs);
}
@Override
public String loadAndRunTemplate(String templatePath, Map pageArgs,
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy