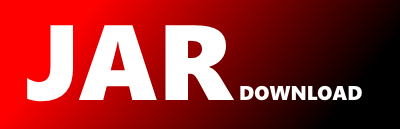
org.whispersystems.libsignal.ecc.ECPublicKey Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of signal-client-java Show documentation
Show all versions of signal-client-java Show documentation
Signal Protocol cryptography library for Java
The newest version!
/**
* Copyright (C) 2013-2016 Open Whisper Systems
*
* Licensed according to the LICENSE file in this repository.
*/
package org.whispersystems.libsignal.ecc;
import org.signal.client.internal.Native;
import org.signal.client.internal.NativeHandleGuard;
import java.util.Arrays;
public class ECPublicKey implements Comparable, NativeHandleGuard.Owner {
public static final int KEY_SIZE = 33;
private final long unsafeHandle;
public ECPublicKey(byte[] serialized, int offset) {
this.unsafeHandle = Native.ECPublicKey_Deserialize(serialized, offset);
}
public ECPublicKey(byte[] serialized) {
this.unsafeHandle = Native.ECPublicKey_Deserialize(serialized, 0);
}
static public ECPublicKey fromPublicKeyBytes(byte[] key) {
byte[] with_type = new byte[33];
with_type[0] = 0x05;
System.arraycopy(key, 0, with_type, 1, 32);
return new ECPublicKey(Native.ECPublicKey_Deserialize(with_type, 0));
}
public ECPublicKey(long nativeHandle) {
if (nativeHandle == 0) {
throw new NullPointerException();
}
this.unsafeHandle = nativeHandle;
}
@Override
protected void finalize() {
Native.ECPublicKey_Destroy(this.unsafeHandle);
}
public boolean verifySignature(byte[] message, byte[] signature) {
try (NativeHandleGuard guard = new NativeHandleGuard(this)) {
return Native.ECPublicKey_Verify(guard.nativeHandle(), message, signature);
}
}
public byte[] serialize() {
try (NativeHandleGuard guard = new NativeHandleGuard(this)) {
return Native.ECPublicKey_Serialize(guard.nativeHandle());
}
}
public byte[] getPublicKeyBytes() {
try (NativeHandleGuard guard = new NativeHandleGuard(this)) {
return Native.ECPublicKey_GetPublicKeyBytes(guard.nativeHandle());
}
}
public int getType() {
byte[] serialized = this.serialize();
return serialized[0];
}
public long unsafeNativeHandleWithoutGuard() {
return this.unsafeHandle;
}
@Override
public boolean equals(Object other) {
if (other == null) return false;
if (!(other instanceof ECPublicKey)) return false;
ECPublicKey that = (ECPublicKey)other;
return Arrays.equals(this.serialize(), that.serialize());
}
@Override
public int hashCode() {
return Arrays.hashCode(this.serialize());
}
@Override
public int compareTo(ECPublicKey another) {
try (
NativeHandleGuard guard = new NativeHandleGuard(this);
NativeHandleGuard otherGuard = new NativeHandleGuard(another);
) {
return Native.ECPublicKey_Compare(guard.nativeHandle(), otherGuard.nativeHandle());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy