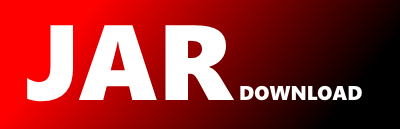
org.whispersystems.libsignal.state.PreKeyBundle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of signal-client-java Show documentation
Show all versions of signal-client-java Show documentation
Signal Protocol cryptography library for Java
The newest version!
/**
* Copyright (C) 2014-2016 Open Whisper Systems
*
* Licensed according to the LICENSE file in this repository.
*/
package org.whispersystems.libsignal.state;
import org.signal.client.internal.Native;
import org.signal.client.internal.NativeHandleGuard;
import org.whispersystems.libsignal.IdentityKey;
import org.whispersystems.libsignal.ecc.ECPublicKey;
/**
* A class that contains a remote PreKey and collection
* of associated items.
*
* @author Moxie Marlinspike
*/
public class PreKeyBundle implements NativeHandleGuard.Owner {
private final long unsafeHandle;
@Override
protected void finalize() {
Native.PreKeyBundle_Destroy(this.unsafeHandle);
}
public PreKeyBundle(int registrationId, int deviceId, int preKeyId, ECPublicKey preKeyPublic,
int signedPreKeyId, ECPublicKey signedPreKeyPublic, byte[] signedPreKeySignature,
IdentityKey identityKey)
{
try (
NativeHandleGuard preKeyPublicGuard = new NativeHandleGuard(preKeyPublic);
NativeHandleGuard signedPreKeyPublicGuard = new NativeHandleGuard(signedPreKeyPublic);
NativeHandleGuard identityKeyGuard = new NativeHandleGuard(identityKey.getPublicKey());
) {
this.unsafeHandle = Native.PreKeyBundle_New(
registrationId,
deviceId,
preKeyId,
preKeyPublicGuard.nativeHandle(),
signedPreKeyId,
signedPreKeyPublicGuard.nativeHandle(),
signedPreKeySignature,
identityKeyGuard.nativeHandle());
}
}
/**
* @return the device ID this PreKey belongs to.
*/
public int getDeviceId() {
try (NativeHandleGuard guard = new NativeHandleGuard(this)) {
return Native.PreKeyBundle_GetDeviceId(guard.nativeHandle());
}
}
/**
* @return the unique key ID for this PreKey.
*/
public int getPreKeyId() {
try (NativeHandleGuard guard = new NativeHandleGuard(this)) {
return Native.PreKeyBundle_GetPreKeyId(guard.nativeHandle());
}
}
/**
* @return the public key for this PreKey.
*/
public ECPublicKey getPreKey() {
try (NativeHandleGuard guard = new NativeHandleGuard(this)) {
long handle = Native.PreKeyBundle_GetPreKeyPublic(guard.nativeHandle());
if (handle != 0) {
return new ECPublicKey(handle);
}
return null;
}
}
/**
* @return the unique key ID for this signed prekey.
*/
public int getSignedPreKeyId() {
try (NativeHandleGuard guard = new NativeHandleGuard(this)) {
return Native.PreKeyBundle_GetSignedPreKeyId(guard.nativeHandle());
}
}
/**
* @return the signed prekey for this PreKeyBundle.
*/
public ECPublicKey getSignedPreKey() {
try (NativeHandleGuard guard = new NativeHandleGuard(this)) {
return new ECPublicKey(Native.PreKeyBundle_GetSignedPreKeyPublic(guard.nativeHandle()));
}
}
/**
* @return the signature over the signed prekey.
*/
public byte[] getSignedPreKeySignature() {
try (NativeHandleGuard guard = new NativeHandleGuard(this)) {
return Native.PreKeyBundle_GetSignedPreKeySignature(guard.nativeHandle());
}
}
/**
* @return the {@link org.whispersystems.libsignal.IdentityKey} of this PreKeys owner.
*/
public IdentityKey getIdentityKey() {
try (NativeHandleGuard guard = new NativeHandleGuard(this)) {
return new IdentityKey(new ECPublicKey(Native.PreKeyBundle_GetIdentityKey(guard.nativeHandle())));
}
}
/**
* @return the registration ID associated with this PreKey.
*/
public int getRegistrationId() {
try (NativeHandleGuard guard = new NativeHandleGuard(this)) {
return Native.PreKeyBundle_GetRegistrationId(guard.nativeHandle());
}
}
public long unsafeNativeHandleWithoutGuard() {
return this.unsafeHandle;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy