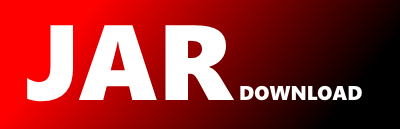
org.whispersystems.signalservice.api.messages.SignalServiceContent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of signal-service-java Show documentation
Show all versions of signal-service-java Show documentation
Signal Service communication library for Java
/*
* Copyright (C) 2014-2016 Open Whisper Systems
*
* Licensed according to the LICENSE file in this repository.
*/
package org.whispersystems.signalservice.api.messages;
import org.whispersystems.libsignal.util.guava.Optional;
import org.whispersystems.signalservice.api.messages.calls.SignalServiceCallMessage;
import org.whispersystems.signalservice.api.messages.multidevice.SignalServiceSyncMessage;
public class SignalServiceContent {
private final String sender;
private final int senderDevice;
private final long timestamp;
private final boolean needsReceipt;
private final Optional message;
private final Optional synchronizeMessage;
private final Optional callMessage;
private final Optional readMessage;
private final Optional typingMessage;
public SignalServiceContent(SignalServiceDataMessage message, String sender, int senderDevice, long timestamp, boolean needsReceipt) {
this.sender = sender;
this.senderDevice = senderDevice;
this.timestamp = timestamp;
this.needsReceipt = needsReceipt;
this.message = Optional.fromNullable(message);
this.synchronizeMessage = Optional.absent();
this.callMessage = Optional.absent();
this.readMessage = Optional.absent();
this.typingMessage = Optional.absent();
}
public SignalServiceContent(SignalServiceSyncMessage synchronizeMessage, String sender, int senderDevice, long timestamp, boolean needsReceipt) {
this.sender = sender;
this.senderDevice = senderDevice;
this.timestamp = timestamp;
this.needsReceipt = needsReceipt;
this.message = Optional.absent();
this.synchronizeMessage = Optional.fromNullable(synchronizeMessage);
this.callMessage = Optional.absent();
this.readMessage = Optional.absent();
this.typingMessage = Optional.absent();
}
public SignalServiceContent(SignalServiceCallMessage callMessage, String sender, int senderDevice, long timestamp, boolean needsReceipt) {
this.sender = sender;
this.senderDevice = senderDevice;
this.timestamp = timestamp;
this.needsReceipt = needsReceipt;
this.message = Optional.absent();
this.synchronizeMessage = Optional.absent();
this.callMessage = Optional.of(callMessage);
this.readMessage = Optional.absent();
this.typingMessage = Optional.absent();
}
public SignalServiceContent(SignalServiceReceiptMessage receiptMessage, String sender, int senderDevice, long timestamp, boolean needsReceipt) {
this.sender = sender;
this.senderDevice = senderDevice;
this.timestamp = timestamp;
this.needsReceipt = needsReceipt;
this.message = Optional.absent();
this.synchronizeMessage = Optional.absent();
this.callMessage = Optional.absent();
this.readMessage = Optional.of(receiptMessage);
this.typingMessage = Optional.absent();
}
public SignalServiceContent(SignalServiceTypingMessage typingMessage, String sender, int senderDevice, long timestamp, boolean needsReceipt) {
this.sender = sender;
this.senderDevice = senderDevice;
this.timestamp = timestamp;
this.needsReceipt = needsReceipt;
this.message = Optional.absent();
this.synchronizeMessage = Optional.absent();
this.callMessage = Optional.absent();
this.readMessage = Optional.absent();
this.typingMessage = Optional.of(typingMessage);
}
public Optional getDataMessage() {
return message;
}
public Optional getSyncMessage() {
return synchronizeMessage;
}
public Optional getCallMessage() {
return callMessage;
}
public Optional getReceiptMessage() {
return readMessage;
}
public Optional getTypingMessage() {
return typingMessage;
}
public String getSender() {
return sender;
}
public int getSenderDevice() {
return senderDevice;
}
public long getTimestamp() {
return timestamp;
}
public boolean isNeedsReceipt() {
return needsReceipt;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy