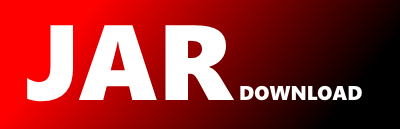
org.whitesource.agent.api.model.ScanSummaryInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wss-agent-api Show documentation
Show all versions of wss-agent-api Show documentation
Java bindings for White Source API
package org.whitesource.agent.api.model;
import java.io.Serializable;
import java.util.*;
/**
* @author chen.luigi
*/
public class ScanSummaryInfo implements Serializable {
/* --- Static members --- */
private static final long serialVersionUID = -3801913583253812987L;
/* --- Private Members --- */
private long totalElapsedTime;
private Collection stepsSummaryInfo;
private List packageManagers;
private List sourceControlManagers;
private List containerRegistries;
private List otherIntegrationTypes;
private boolean isPrivileged;
private ScanMethod scanMethod;
private Map scanStatistics;
/* --- Constructors --- */
public ScanSummaryInfo() {
stepsSummaryInfo = new LinkedList<>();
packageManagers = new LinkedList<>();
containerRegistries = new LinkedList<>();
sourceControlManagers = new LinkedList<>();
otherIntegrationTypes = new LinkedList<>();
scanStatistics = new HashMap<>();
}
public ScanSummaryInfo(long totalElapsedTime, Collection stepsSummaryInfo) {
this();
this.totalElapsedTime = totalElapsedTime;
this.stepsSummaryInfo = stepsSummaryInfo;
}
/* --- Overridden methods --- */
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof ScanSummaryInfo)) return false;
ScanSummaryInfo that = (ScanSummaryInfo) o;
return totalElapsedTime == that.totalElapsedTime &&
Objects.equals(stepsSummaryInfo, that.stepsSummaryInfo);
}
@Override
public int hashCode() {
return Objects.hash(totalElapsedTime, stepsSummaryInfo);
}
/* --- Getters / Setters --- */
public Map getScanStatistics() {
return scanStatistics;
}
public void setScanStatistics(Map scanStatistics) {
this.scanStatistics = scanStatistics;
}
public long getTotalElapsedTime() {
return totalElapsedTime;
}
public void setTotalElapsedTime(long totalElapsedTime) {
this.totalElapsedTime = totalElapsedTime;
}
public Collection getStepsSummaryInfo() {
return stepsSummaryInfo;
}
public void setStepsSummaryInfo(Collection stepsSummaryInfo) {
this.stepsSummaryInfo = stepsSummaryInfo;
}
public List getPackageManagers() {
return packageManagers;
}
public void setPackageManagers(List packageManagers) {
this.packageManagers = packageManagers;
}
public List getSourceControlManagers() {
return sourceControlManagers;
}
public void setSourceControlManagers(List sourceControlManagers) {
this.sourceControlManagers = sourceControlManagers;
}
public List getContainerRegistries() {
return containerRegistries;
}
public void setContainerRegistries(List containerRegistries) {
this.containerRegistries = containerRegistries;
}
public List getOtherIntegrationTypes() {
return otherIntegrationTypes;
}
public void setOtherIntegrationTypes(List otherIntegrationTypes) {
this.otherIntegrationTypes = otherIntegrationTypes;
}
public boolean isPrivileged() {
return isPrivileged;
}
public void setPrivileged(boolean privileged) {
isPrivileged = privileged;
}
public ScanMethod getScanMethod() {
return scanMethod;
}
public void setScanMethod(ScanMethod scanMethod) {
this.scanMethod = scanMethod;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy