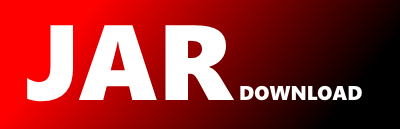
org.wicketstuff.jqplot.lib.chart.AbstractChart Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jqplot4java Show documentation
Show all versions of jqplot4java Show documentation
This project provides a Java library for utilizing JqPlot Pure Javascript (http://www.jqplot.com/).
/*
* Copyright 2011 Inaiat H. Moraes.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* under the License.
*/
package org.wicketstuff.jqplot.lib.chart;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import org.wicketstuff.jqplot.lib.Chart;
import org.wicketstuff.jqplot.lib.ChartConfiguration;
import org.wicketstuff.jqplot.lib.axis.Axis;
import org.wicketstuff.jqplot.lib.axis.XAxis;
import org.wicketstuff.jqplot.lib.axis.YAxis;
import org.wicketstuff.jqplot.lib.data.ChartData;
import org.wicketstuff.jqplot.lib.elements.Axes;
import org.wicketstuff.jqplot.lib.elements.CanvasOverlay;
import org.wicketstuff.jqplot.lib.elements.GridPadding;
import org.wicketstuff.jqplot.lib.elements.Legend;
import org.wicketstuff.jqplot.lib.elements.Serie;
import org.wicketstuff.jqplot.lib.elements.SeriesDefaults;
import org.wicketstuff.jqplot.lib.elements.Title;
/**
* Abstract class to help build charts.
*
* @author inaiat
*
* @param
* Type of {@link Axis}
*
*/
public abstract class AbstractChart, S extends Serializable>
implements Chart {
private static final long serialVersionUID = -5744130130488157491L;
public AbstractChart addSeriesColors(String... colors) {
getChartConfiguration().seriesColorsInstance().addAll(
Arrays.asList(colors));
return this;
}
public AbstractChart addSeriesColors(Collection colors) {
getChartConfiguration().seriesColorsInstance().addAll(colors);
return this;
}
public AbstractChart setSeriesColors(Collection colors) {
getChartConfiguration().setSeriesColors(colors);
return this;
}
public Collection getSeriesColors() {
return getChartConfiguration().seriesColorsInstance();
}
/**
* Adiciona uma serie
*
* @param serie Add serie of chart
* @return AbstractChart
*/
public AbstractChart addSerie(Serie serie) {
Collection series = getSeries();
if (series == null) {
series = new ArrayList<>();
}
series.add(serie);
return this;
}
/**
* Add a collection of series
*
* @param series Add series of chart
* @return AbstractChart
*/
public AbstractChart addSeries(Serie... series) {
Collection chartSeries = getSeries();
if (chartSeries == null) {
chartSeries = new ArrayList<>();
}
for (int i = series.length - 1; i >= 0; i--) {
chartSeries.add(series[i]);
}
return this;
}
/**
*
* @return chartConfiguration
*/
@Override
public abstract ChartConfiguration getChartConfiguration();
/**
*
* @param title Set title of chart
* @return AbstractChart
*/
public AbstractChart setSimpleTitle(String title) {
getChartConfiguration().setSimpleTitle(title);
return this;
}
/**
*
* @param label Set label for axis X
* @return AbstractChart
*/
public AbstractChart setLabelX(String label) {
getChartConfiguration().setLabelX(label);
return this;
}
/**
*
* @param label Set label for axis Y
* @return AbstractChart
*/
public AbstractChart setLabelY(String label) {
getChartConfiguration().setLabelY(label);
return this;
}
/**
* @return the series
*/
public Collection getSeries() {
return getChartConfiguration().seriesInstance();
}
/**
* @param series
* the series to set
*
* @return AbstractChart
*/
public AbstractChart setSeries(Collection series) {
this.getChartConfiguration().setSeries(series);
return this;
}
/**
* @return the title
*/
public Title getTitle() {
return getChartConfiguration().getTitle();
}
/**
* @param title
* the title to set
* @return AbstractChart
*/
public AbstractChart setTitle(Title title) {
getChartConfiguration().setTitle(title);
return this;
}
/**
* @return the axesDefaults
*/
public Axis getAxesDefaults() {
return getChartConfiguration().axesDefaultsInstance();
}
/**
* @param axesDefaults
* the axesDefaults to set
* @return AbstractChart
*/
public AbstractChart setAxesDefaults(Axis axesDefaults) {
getChartConfiguration().setAxesDefaults(axesDefaults);
return this;
}
/**
* @return the seriesDefaults
*/
public SeriesDefaults getSeriesDefaults() {
return getChartConfiguration().seriesDefaultsInstance();
}
/**
* @param seriesDefaults
* the seriesDefaults to set
* @return AbstractChart
*/
public AbstractChart setSeriesDefaults(SeriesDefaults seriesDefaults) {
getChartConfiguration().setSeriesDefaults(seriesDefaults);
return this;
}
/**
*
* @param values Set values for interval colors
* @return AbstractChart
*/
public AbstractChart addIntervalColors(String... values) {
getChartConfiguration().seriesDefaultsInstance().getRendererOptions()
.getIntervalColors().addAll(Arrays.asList(values));
return this;
}
/**
*
* @param values Set interval values
* @return AbstractChart
*/
public AbstractChart addIntervals(Integer... values) {
getChartConfiguration().seriesDefaultsInstance().getRendererOptions()
.getIntervals().addAll(Arrays.asList(values));
return this;
}
/**
*
* @param stackSeries Enable/Disable stackSeries
* @return AbstractChart
*/
public AbstractChart setStackSeries(Boolean stackSeries) {
getChartConfiguration().setStackSeries(stackSeries);
return this;
}
/**
*
* @param captureRightClick Enable/Disable captureRightClick
* @return AbstractChart
*/
public AbstractChart setCaptureRightClick(Boolean captureRightClick) {
getChartConfiguration().setCaptureRightClick(captureRightClick);
return this;
}
/**
*
* @param highlightMouseDown Enable/Disable highlighMouseDown
* @return AbstractChart
*/
public AbstractChart setHighlightMouseDown(Boolean highlightMouseDown) {
getChartConfiguration().seriesDefaultsInstance().rendererOptionsInstance()
.setHighlightMouseDown(highlightMouseDown);
return this;
}
/**
*
* @param margin Set value of margin
* @return AbstractChart
*/
public AbstractChart setBarMargin(Integer margin) {
getChartConfiguration().seriesDefaultsInstance().rendererOptionsInstance()
.setBarMargin(margin);
return this;
}
/**
*
* @param margin Set value o slice margin
* @return AbstractChart
*/
public AbstractChart setSliceMargin(Integer margin) {
getChartConfiguration().seriesDefaultsInstance().rendererOptionsInstance()
.setSliceMargin(margin);
return this;
}
/**
*
* @param dataLabels Set data labels
* @return AbstractChart
*/
public AbstractChart setDataLabels(String dataLabels) {
getChartConfiguration().seriesDefaultsInstance().rendererOptionsInstance()
.setDataLabels(dataLabels);
return this;
}
/**
*
* @param width Set line width
* @return AbstractChart
*/
public AbstractChart setLineWidth(Integer width) {
getChartConfiguration().seriesDefaultsInstance().rendererOptionsInstance()
.setLineWidth(width);
return this;
}
/**
*
* @param showDataLabels Enable/Disable show data labels
* @return AbstractChart
*/
public AbstractChart setShowDataLabels(Boolean showDataLabels) {
getChartConfiguration().seriesDefaultsInstance().rendererOptionsInstance()
.setShowDataLabels(showDataLabels);
return this;
}
/**
*
* @param fill Enable/Disable fill
* @return AbstractChart
*/
public AbstractChart setFill(Boolean fill) {
getChartConfiguration().seriesDefaultsInstance().rendererOptionsInstance()
.setFill(fill);
return this;
}
/**
*
* @param alpha Set value for bubble alpha
* @return AbstractChart
*/
public AbstractChart setBubbleAlpha(Float alpha) {
getChartConfiguration().seriesDefaultsInstance().rendererOptionsInstance()
.setBubbleAlpha(alpha);
return this;
}
/**
*
* @param alpha Set value for highlight alpha
* @return AbstractChart
*/
public AbstractChart setHighlightAlpha(Float alpha) {
getChartConfiguration().seriesDefaultsInstance().rendererOptionsInstance()
.setHighlightAlpha(alpha);
return this;
}
/**
*
* @param showLabels Enable/Disable show labels
* @return AbstractChart
*/
public AbstractChart setShowLabels(Boolean showLabels) {
getChartConfiguration().seriesDefaultsInstance().rendererOptionsInstance()
.setShowLables(showLabels);
return this;
}
/**
*
* @param alpha Set value for shadow alpha
* @return AbstractChart
*/
public AbstractChart setShadowAlpha(String alpha) {
getChartConfiguration().seriesDefaultsInstance().setShadowAlpha(alpha);
return this;
}
/**
*
* @param fillZero Enable/Disable fill zeros
* @return AbstractChart
*/
public AbstractChart setFillZero(Boolean fillZero) {
getChartConfiguration().seriesDefaultsInstance().rendererOptionsInstance()
.setFillZero(fillZero);
return this;
}
/**
*
* @param shadow Enable/Disable shadow
* @return AbstractChart
*/
public AbstractChart setShadow(Boolean shadow) {
getChartConfiguration().getSeriesDefaults().setShadow(shadow);
return this;
}
/**
*
* @param legend Set {@link Legend} object
* @return AbstractChart
*/
public AbstractChart setLegend(Legend legend) {
getChartConfiguration().setLegend(legend);
return this;
}
/**
*
* @param gridPadding Set {@link GridPadding} object
* @return AbstractChart
*/
public AbstractChart setGridPadding(GridPadding gridPadding) {
getChartConfiguration().setGridPadding(gridPadding);
return this;
}
public GridPadding getGridPadding() {
return getChartConfiguration().getGridPadding();
}
/**
* @return the axes
*/
public Axes getAxes() {
return getChartConfiguration().axesInstance();
}
/**
* @param axes
* the axes to set
* @return AbstractChart
*/
public AbstractChart setAxes(Axes axes) {
getChartConfiguration().setAxes(axes);
return this;
}
public XAxis getXAxis() {
return getChartConfiguration().xAxisInstance();
}
public YAxis getYAxis() {
return getChartConfiguration().yAxisInstance();
}
/**
* @return the canvas overlay
*/
public CanvasOverlay getCanvasOverlay() {
return getChartConfiguration().canvasOverlayInstance();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy