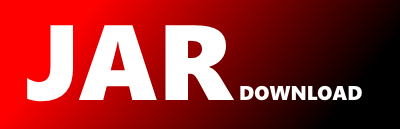
org.wicketstuff.datatables.Options Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wicketstuff-datatables Show documentation
Show all versions of wicketstuff-datatables Show documentation
DataTables jQuery integration for Wicket
package org.wicketstuff.datatables;
import de.agilecoders.wicket.jquery.AbstractConfig;
import de.agilecoders.wicket.jquery.IKey;
import de.agilecoders.wicket.jquery.Key;
import de.agilecoders.wicket.jquery.util.Json;
import org.apache.wicket.util.lang.Args;
/**
*
*/
public class Options extends AbstractConfig {
/**
* https://datatables.net/examples/basic_init/alt_pagination.html
*/
public enum PagingType {
/**
* 'Previous' and 'Next' buttons only
*/
simple,
/**
* 'Previous' and 'Next' buttons, plus page numbers
*/
simple_numbers,
/**
* 'First', 'Previous', 'Next' and 'Last' buttons
*/
full,
/**
* 'First', 'Previous', 'Next' and 'Last' buttons, plus page numbers
*/
full_numbers
}
/**
* https://datatables.net/examples/basic_init/table_sorting.html
*/
private static final IKey Order = new Key("order", null);
/**
* https://datatables.net/examples/basic_init/state_save.html
*/
private static final IKey StateSave = new Key("stateSave", false);
/**
* In seconds.
*
* https://datatables.net/examples/basic_init/state_save.html
* https://datatables.net/reference/option/stateDuration
*/
private static final IKey StateDuration = new Key("stateDuration", 7200);
/**
* https://datatables.net/examples/basic_init/alt_pagination.html
*/
private static final IKey _PagingType = new Key("pagingType", PagingType.simple_numbers);
/**
* https://datatables.net/reference/option/paging
*/
private static final IKey Paging = new Key("paging", true);
/**
* https://datatables.net/examples/basic_init/scroll_y.html
*/
private static final IKey ScrollY = new Key("scrollY", null);
/**
* https://datatables.net/examples/basic_init/scroll_y.html
* https://datatables.net/reference/option/scrollCollapse
*/
private static final IKey ScrollCollapse = new Key("scrollCollapse", false);
/**
* https://datatables.net/examples/basic_init/scroll_x.html
* https://datatables.net/reference/option/scrollX
*/
private static final IKey ScrollX = new Key("scrollX", false);
/**
* https://datatables.net/examples/server_side/select_rows.html
* https://datatables.net/reference/option/rowCallback
*/
private static final IKey RowCallback = new Key("rowCallback", null);
/**
*
* https://datatables.net/reference/option/deferLoading
*/
private static final IKey DeferLoading = new Key("deferLoading", null);
/**
* https://datatables.net/examples/advanced_init/row_callback.html
* https://datatables.net/reference/option/createdRow
*/
private static final IKey CreatedRow = new Key("createdRow", null);
/**
* https://datatables.net/examples/advanced_init/length_menu.html
* https://datatables.net/reference/option/lengthMenu
*/
private static final IKey
© 2015 - 2025 Weber Informatics LLC | Privacy Policy