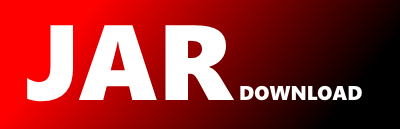
com.inmethod.grid.column.PropertyColumn Maven / Gradle / Ivy
package com.inmethod.grid.column;
import java.util.Locale;
import org.apache.wicket.Application;
import org.apache.wicket.Session;
import org.apache.wicket.core.util.lang.PropertyResolver;
import org.apache.wicket.extensions.markup.html.repeater.data.sort.ISortState;
import org.apache.wicket.model.IModel;
import org.apache.wicket.request.Response;
import org.apache.wicket.util.convert.IConverter;
import org.apache.wicket.util.string.Strings;
import com.inmethod.grid.IRenderable;
/**
* A lightweight column that displays a property of row object specified by an property expression.
*
* @param
* grid model object type
* @param
* row/item model object type
* @param
* type of the property
*
* @author Matej Knopp
*/
public class PropertyColumn extends AbstractLightWeightColumn
{
private static final long serialVersionUID = 1L;
private final String propertyExpression;
/**
* Constructor.
*
* @param columnId
* column identified (must be unique within the grid)
* @param headerModel
* model for column header
* @param propertyExpression
* property expression used to get the displayed value for row object
* @param sortProperty
* optional string that will be returned by {@link ISortState} to indicate that the
* column is being sorted
*/
public PropertyColumn(String columnId, IModel headerModel, String propertyExpression,
S sortProperty)
{
super(columnId, headerModel, sortProperty);
this.propertyExpression = propertyExpression;
}
/**
* Constructor.
*
* @param columnId
* column identified (must be unique within the grid)
* @param headerModel
* model for column header
* @param propertyExpression
* property expression used to get the displayed value for row object
*/
public PropertyColumn(String columnId, IModel headerModel, String propertyExpression)
{
this(columnId, headerModel, propertyExpression, null);
}
/**
* Constructor. The column id is omitted in this constructor, because the property expression is
* used as column id.
*
* @param headerModel
* model for column header
* @param propertyExpression
* property expression used to get the displayed value for row object
* @param sortProperty
* optional string that will be returned by {@link ISortState} to indicate that the
* column is being sorted
*/
public PropertyColumn(IModel headerModel, String propertyExpression, S sortProperty)
{
this(propertyExpression, headerModel, propertyExpression, sortProperty);
}
/**
* Constructor. The column id is omitted in this constructor, because the property expression is
* used as column id.
*
* @param headerModel
* model for column header
* @param propertyExpression
* property expression used to get the displayed value for row object
*/
public PropertyColumn(IModel headerModel, String propertyExpression)
{
this(propertyExpression, headerModel, propertyExpression);
}
private boolean escapeMarkup = true;
/**
* Sets whether the markup will be escaped. Set to false
if the property contains
* html snippets that need to be rendered as html (without being escaped).
*
* @param escape
* @return this
(useful for method chaining)
*/
public PropertyColumn setEscapeMarkup(boolean escape)
{
escapeMarkup = escape;
return this;
}
/**
* Returns whether the markup will be escaped.
*
* @return true
. if the markup will be escaped, false
otherwise
*/
public boolean isEscapeMarkup()
{
return escapeMarkup;
}
protected P getProperty(Object object, String propertyExpression)
{
return (P)PropertyResolver.getValue(propertyExpression, object);
}
protected I getModelObject(IModel rowModel)
{
return rowModel.getObject();
}
private CharSequence getValue(IModel rowModel)
{
I rowObject = getModelObject(rowModel);
P property = null;
if (rowObject != null)
{
try
{
property = getProperty(rowObject, getPropertyExpression());
}
catch (NullPointerException e)
{
}
}
CharSequence string = convertToString(property);
if (isEscapeMarkup() && string != null)
{
string = Strings.escapeMarkup(string.toString());
}
return string;
}
protected IConverter getConverter(Class type)
{
return Application.get().getConverterLocator().getConverter(type);
}
protected Locale getLocale()
{
return Session.get().getLocale();
}
protected CharSequence convertToString(C object)
{
if (object != null)
{
@SuppressWarnings("unchecked")
Class cKlazz = (Class)object.getClass();
IConverter converter = getConverter(cKlazz);
return converter.convertToString(object, getLocale());
}
return "";
}
/**
* {@inheritDoc}
*/
@Override
public IRenderable newCell(IModel rowModel)
{
return new IRenderable()
{
@Override
public void render(IModel rowModel, Response response)
{
CharSequence value = getValue(rowModel);
if (value != null)
{
response.write(value);
}
}
};
}
/**
* Returns the property expression.
*
* @return property expression
*/
public String getPropertyExpression()
{
return propertyExpression;
}
}