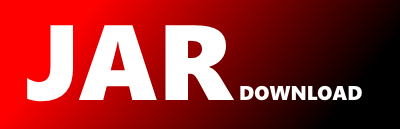
com.inmethod.grid.column.editable.DropDownChoiceColumn Maven / Gradle / Ivy
package com.inmethod.grid.column.editable;
import java.util.List;
import org.apache.wicket.extensions.markup.html.repeater.data.sort.ISortState;
import org.apache.wicket.markup.html.form.IChoiceRenderer;
import org.apache.wicket.model.IModel;
import org.apache.wicket.model.Model;
/**
* Property column that uses a {@link DropDownChoicePanel} as cell component
* when the item is selected.
*
* @author Tom Burton
*/
public class DropDownChoiceColumn extends EditablePropertyColumn
{
private static final long serialVersionUID = 1L;
/** following {@Link AbstractChoice}'s example
* and using {@link Model#ofList(List)} as default model*/
private IModel extends List extends T>> choicesModel;
private IChoiceRenderer choiceRenderer = null;
/**
* Constructor.
*
* @param columnId
* column identified (must be unique within the grid)
* @param headerModel
* model for column header
* @param propertyExpression
* property expression used to get the displayed value for row object
* @param sortProperty
* optional string that will be returned by {@link ISortState} to indicate that the
* column is being sorted
*/
public DropDownChoiceColumn(String columnId, IModel headerModel, String propertyExpression, S sortProperty)
{
super(columnId, headerModel, propertyExpression, sortProperty);
}
/**
* Constructor.
*
* @param columnId
* column identified (must be unique within the grid)
* @param headerModel
* model for column header
* @param propertyExpression
* property expression used to get the displayed value for row
* object
*/
public DropDownChoiceColumn(String columnId, IModel headerModel, String propertyExpression)
{
super(columnId, headerModel, propertyExpression);
}
/**
* Constructor. The column id is omitted in this constructor,
* because the property expression is used as column id.
*
* @param headerModel
* model for column header
* @param propertyExpression
* property expression used to get the displayed value for row object
* @param sortProperty
* optional string that will be returned by {@link ISortState}
* to indicate that the
* column is being sorted
*/
public DropDownChoiceColumn(IModel headerModel, String propertyExpression, S sortProperty)
{
super(headerModel, propertyExpression, sortProperty);
}
/**
* Constructor. The column id is omitted in this constructor, because the property expression is
* used as column id.
*
* @param headerModel
* model for column header
* @param propertyExpression
* property expression used to get the displayed value for row object
*/
public DropDownChoiceColumn(IModel headerModel, String propertyExpression)
{
super(headerModel, propertyExpression);
}
/**
* Constructor.
*
* @param columnId
* column identified (must be unique within the grid)
* @param headerModel
* model for column header
* @param propertyExpression
* property expression used to get the displayed value for row object
* @param sortProperty
* optional string that will be returned by {@link ISortState} to indicate that the
* column is being sorted
* @param choices
* The collection of choices in the drop down when the cell becomes editable
*/
public DropDownChoiceColumn(String columnId, IModel headerModel, String propertyExpression, S sortProperty,
IModel extends List extends T>> choices)
{
super(columnId, headerModel, propertyExpression, sortProperty);
choicesModel = choices;
}
/**
* Constructor.
*
* @param columnId
* column identified (must be unique within the grid)
* @param headerModel
* model for column header
* @param propertyExpression
* property expression used to get the displayed value for row
* object
* @param choices
* The collection of choices in the drop down when the cell becomes editable
*/
public DropDownChoiceColumn(String columnId, IModel headerModel,
String propertyExpression,
IModel extends List extends T>> choices)
{
super(columnId, headerModel, propertyExpression);
choicesModel = choices;
}
/**
* Constructor. The column id is omitted in this constructor,
* because the property expression is used as column id.
*
* @param headerModel
* model for column header
* @param propertyExpression
* property expression used to get the displayed value for row object
* @param sortProperty
* optional string that will be returned by {@link ISortState}
* to indicate that the
* column is being sorted
* @param choices
* The collection of choices in the drop down when the cell becomes editable
*/
public DropDownChoiceColumn(IModel headerModel, String propertyExpression, S sortProperty,
IModel extends List extends T>> choices)
{
super(headerModel, propertyExpression, sortProperty);
choicesModel = choices;
}
/**
* Constructor. The column id is omitted in this constructor, because the property expression is
* used as column id.
*
* @param headerModel
* model for column header
* @param propertyExpression
* property expression used to get the displayed value for row object
* @param choices
* The collection of choices in the drop down when the cell becomes editable
*/
public DropDownChoiceColumn(IModel headerModel, String propertyExpression,
IModel extends List extends T>> choices)
{
super(headerModel, propertyExpression);
choicesModel = choices;
}
/**
* Constructor.
*
* @param columnId
* column identified (must be unique within the grid)
* @param headerModel
* model for column header
* @param propertyExpression
* property expression used to get the displayed value for row object
* @param sortProperty
* optional string that will be returned by {@link ISortState} to indicate that the
* column is being sorted
* @param choices
* The collection of choices in the drop down when the cell becomes editable
* @param renderer
* The rendering engine
*/
public DropDownChoiceColumn(String columnId, IModel headerModel,
String propertyExpression, S sortProperty,
IModel extends List extends T>> choices,
IChoiceRenderer renderer)
{
super(columnId, headerModel, propertyExpression, sortProperty);
choicesModel = choices;
choiceRenderer = renderer;
}
/**
* Constructor.
*
* @param columnId
* column identified (must be unique within the grid)
* @param headerModel
* model for column header
* @param propertyExpression
* property expression used to get the displayed value for row
* object
* @param choices
* The collection of choices in the drop down when the cell becomes editable
* @param renderer
* The rendering engine
*/
public DropDownChoiceColumn(String columnId, IModel headerModel,
String propertyExpression,
IModel extends List extends T>> choices,
IChoiceRenderer renderer)
{
super(columnId, headerModel, propertyExpression);
choicesModel = choices;
choiceRenderer = renderer;
}
/**
* Constructor. The column id is omitted in this constructor,
* because the property expression is used as column id.
*
* @param headerModel
* model for column header
* @param propertyExpression
* property expression used to get the displayed value for row object
* @param sortProperty
* optional string that will be returned by {@link ISortState}
* to indicate that the
* column is being sorted
* @param choices
* The collection of choices in the drop down when the cell becomes editable
* @param renderer
* The rendering engine
*/
public DropDownChoiceColumn(IModel headerModel, String propertyExpression,
S sortProperty,
IModel extends List extends T>> choices,
IChoiceRenderer renderer)
{
super(headerModel, propertyExpression, sortProperty);
choicesModel = choices;
choiceRenderer = renderer;
}
/**
* Constructor. The column id is omitted in this constructor, because the property expression is
* used as column id.
*
* @param headerModel
* model for column header
* @param propertyExpression
* property expression used to get the displayed value for row object
* @param choices
* The collection of choices in the drop down when the cell becomes editable
* @param renderer
* The rendering engine
*/
public DropDownChoiceColumn(IModel headerModel, String propertyExpression,
IModel extends List extends T>> choices,
IChoiceRenderer renderer)
{
super(headerModel, propertyExpression);
choicesModel = choices;
choiceRenderer = renderer;
}
/**
* Constructor.
*
* @param columnId
* column identified (must be unique within the grid)
* @param headerModel
* model for column header
* @param propertyExpression
* property expression used to get the displayed value for row object
* @param sortProperty
* optional string that will be returned by {@link ISortState} to indicate that the
* column is being sorted
* @param choices
* The collection of choices in the drop down when the cell becomes editable
*/
public DropDownChoiceColumn(String columnId, IModel headerModel,
String propertyExpression, S sortProperty,
List choices)
{
super(columnId, headerModel, propertyExpression, sortProperty);
choicesModel = Model.ofList(choices);
}
/**
* Constructor.
*
* @param columnId
* column identified (must be unique within the grid)
* @param headerModel
* model for column header
* @param propertyExpression
* property expression used to get the displayed value for row
* object
* @param choices
* The collection of choices in the drop down when the cell becomes editable
*/
public DropDownChoiceColumn(String columnId, IModel headerModel,
String propertyExpression, List choices)
{
super(columnId, headerModel, propertyExpression);
choicesModel = Model.ofList(choices);
}
/**
* Constructor. The column id is omitted in this constructor,
* because the property expression is used as column id.
*
* @param headerModel
* model for column header
* @param propertyExpression
* property expression used to get the displayed value for row object
* @param sortProperty
* optional string that will be returned by {@link ISortState}
* to indicate that the
* column is being sorted
* @param choices
* The collection of choices in the drop down when the cell becomes editable
*/
public DropDownChoiceColumn(IModel headerModel,
String propertyExpression, S sortProperty,
List choices)
{
super(headerModel, propertyExpression, sortProperty);
choicesModel = Model.ofList(choices);
}
/**
* Constructor. The column id is omitted in this constructor, because the property expression is
* used as column id.
*
* @param headerModel
* model for column header
* @param propertyExpression
* property expression used to get the displayed value for row object
* @param choices
* The collection of choices in the drop down when the cell becomes editable
*/
public DropDownChoiceColumn(IModel headerModel, String propertyExpression,
List choices)
{
super(headerModel, propertyExpression);
choicesModel = Model.ofList(choices);
}
/**
* Constructor.
*
* @param columnId
* column identified (must be unique within the grid)
* @param headerModel
* model for column header
* @param propertyExpression
* property expression used to get the displayed value for row object
* @param sortProperty
* optional string that will be returned by {@link ISortState} to indicate that the
* column is being sorted
* @param choices
* The collection of choices in the drop down when the cell becomes editable
* @param renderer
* The renderer to use for displaying the data
*/
public DropDownChoiceColumn(String columnId, IModel headerModel,
String propertyExpression, S sortProperty,
List choices, IChoiceRenderer renderer)
{
super(columnId, headerModel, propertyExpression, sortProperty);
choicesModel = Model.ofList(choices);
choiceRenderer = renderer;
}
/**
* Constructor.
*
* @param columnId
* column identified (must be unique within the grid)
* @param headerModel
* model for column header
* @param propertyExpression
* property expression used to get the displayed value for row
* object
* @param choices
* The collection of choices in the drop down when the cell becomes editable
* @param renderer
* The rendering engine
*/
public DropDownChoiceColumn(String columnId, IModel headerModel,
String propertyExpression,
List choices,
IChoiceRenderer renderer)
{
super(columnId, headerModel, propertyExpression);
choicesModel = Model.ofList(choices);
choiceRenderer = renderer;
}
/**
* Constructor. The column id is omitted in this constructor,
* because the property expression is used as column id.
*
* @param headerModel
* model for column header
* @param propertyExpression
* property expression used to get the displayed value for row object
* @param sortProperty
* optional string that will be returned by {@link ISortState}
* to indicate that the
* column is being sorted
* @param choices
* The collection of choices in the drop down when the cell becomes editable
* @param renderer
* The renderer to use for displaying the data
*/
public DropDownChoiceColumn(IModel headerModel, String propertyExpression,
S sortProperty,
List choices, IChoiceRenderer renderer)
{
super(headerModel, propertyExpression, sortProperty);
choicesModel = Model.ofList(choices);
choiceRenderer = renderer;
}
/**
* Constructor. The column id is omitted in this constructor,
* because the property expression is used as column id.
*
* @param headerModel
* model for column header
* @param propertyExpression
* property expression used to get the displayed value for row object
* @param choices
* The collection of choices in the drop down when the cell becomes editable
* @param renderer
* The rendering engine
*/
public DropDownChoiceColumn(IModel headerModel, String propertyExpression,
List choices, IChoiceRenderer renderer)
{
super(headerModel, propertyExpression);
choicesModel = Model.ofList(choices);
choiceRenderer = renderer;
}
/** {@inheritDoc} **/
@Override
protected EditableCellPanel newCellPanel(String componentId, IModel rowModel,
IModel cellModel)
{
return new DropDownChoicePanel(componentId, cellModel, rowModel, this,
choicesModel, choiceRenderer);
}
/** {@inheritDoc} */
@Override
protected CharSequence convertToString(C obj)
{
if (null != obj && null != choiceRenderer)
{
return getConverter((Class)obj.getClass())
.convertToString((C)choiceRenderer.getDisplayValue((T)obj),
getLocale());
}
else if ( null != obj) { return super.convertToString(obj); }
else { return ""; }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy