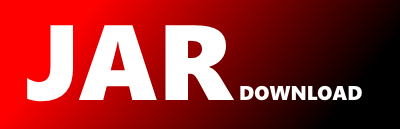
com.inmethod.grid.common.AbstractGridRow Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wicketstuff-inmethod-grid Show documentation
Show all versions of wicketstuff-inmethod-grid Show documentation
Advanced grid components for Apache Wicket
The newest version!
package com.inmethod.grid.common;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import org.apache.wicket.Component;
import org.apache.wicket.behavior.Behavior;
import org.apache.wicket.markup.ComponentTag;
import org.apache.wicket.markup.IMarkupFragment;
import org.apache.wicket.markup.html.WebMarkupContainer;
import org.apache.wicket.model.IModel;
import org.apache.wicket.request.Response;
import org.apache.wicket.request.cycle.RequestCycle;
import com.inmethod.grid.IGridColumn;
import com.inmethod.grid.IGridSortState;
import com.inmethod.grid.IRenderable;
/**
* Represents container of cell items in one row.
*
* @param
* grid model object type
* @param
* row/item model object type
*
* @author Matej Knopp
*/
public abstract class AbstractGridRow extends WebMarkupContainer
{
private static final long serialVersionUID = 1L;
/**
* Constructor
*
* @param id
* @param model
*/
public AbstractGridRow(String id, IModel model)
{
super(id, model);
}
@Override
protected void onBeforeRender()
{ // make sure that the child component match currently active columns
Collection> activeColumns = getActiveColumns();
// remove unneeded components
for (Iterator> i = iterator(); i.hasNext();)
{
Component component = (Component)i.next();
if (isComponentNeeded(component.getId(), activeColumns) == false)
{
i.remove();
}
}
List components = new ArrayList(activeColumns.size());
// create components that might be needed
for (IGridColumn column : activeColumns)
{
String componentId = componentId(column.getId());
Component component = null;
if (!column.isLightWeight(getDefaultRowModel()) &&
(component = get(componentId)) == null)
{
component = column.newCell(this, componentId, getDefaultRowModel());
add(component);
}
if (component != null)
{
components.add(component);
}
}
super.onBeforeRender();
// delay adding the actual behaviors
for (Component component : components)
{
for (IGridColumn column : activeColumns)
{
if (component.getId().equals(componentId(column.getId())))
{
component.add(new InnerDivClassBehavior(column));
}
}
}
}
/**
* Returns true if the column is currently being the one sorted with highest priority (i.e. the
* most recent one the user clicked)
*
* @param column
* @return
*/
private boolean isColumnBeingSorted(IGridColumn column)
{
if (column.getSortProperty() != null)
{
AbstractGrid dataGrid = findParent(AbstractGrid.class);
IGridSortState sortState = dataGrid.getSortState();
return sortState.getColumns().size() > 0 &&
sortState.getColumns().get(0).getPropertyName().equals(column.getSortProperty());
}
else
{
return false;
}
}
/**
* Renders the table cell opening tag.
*
* @param column
* @param i
* column index in row
* @param columnsSize
* @param response
* @param hide
* how many cells remain to be hidden (because some of the previous cells had colspan
* set)
* @return
*/
private int renderOpenTag(IGridColumn column, int i, int columnsSize, Response response,
int hide)
{
int originalColspan = column.getColSpan(getDefaultRowModel());
int colspan = originalColspan;
// render the opening tag
if (hide > 0)
{
response.write("> getActiveColumns();
protected abstract int getRowNumber();
/**
* Returns whether a component with given is needed for any of the columns.
*
* @param componentId
* @param activeColumns
* @return
*/
private boolean isComponentNeeded(final String componentId,
Collection> activeColumns)
{
for (IGridColumn column : activeColumns)
{
if (componentId(column.getId()).equals(componentId) &&
!column.isLightWeight(getDefaultRowModel()))
{
return true;
}
}
return false;
}
protected IModel getDefaultRowModel()
{
return (IModel)getDefaultModel();
}
}
");
++i;
}
}
private String componentId(String columnId)
{
return columnId;
}
protected abstract Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy