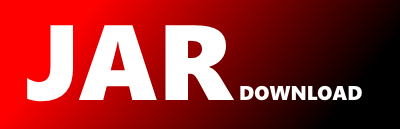
org.wildfly.clustering.context.Contextualizer Maven / Gradle / Ivy
/*
* Copyright The WildFly Authors
* SPDX-License-Identifier: Apache-2.0
*/
package org.wildfly.clustering.context;
import java.util.List;
import java.util.concurrent.Callable;
import java.util.function.BiConsumer;
import java.util.function.BiFunction;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Supplier;
import org.wildfly.common.function.ExceptionBiConsumer;
import org.wildfly.common.function.ExceptionBiFunction;
import org.wildfly.common.function.ExceptionConsumer;
import org.wildfly.common.function.ExceptionFunction;
import org.wildfly.common.function.ExceptionRunnable;
import org.wildfly.common.function.ExceptionSupplier;
/**
* Facility for creating contextual tasks.
* @author Paul Ferraro
*/
public interface Contextualizer {
/**
* Decorates the specified runner with a given context.
* @param runner a runnable task
* @return a contextual runner
*/
Runnable contextualize(Runnable runner);
/**
* Decorates the specified runner with a given context.
* @param the exception type
* @param runner a runnable task
* @return a contextual runner
*/
ExceptionRunnable contextualize(ExceptionRunnable runner);
/**
* Decorates the specified caller with a given context.
* @param the return type
* @param caller a callable task
* @return a contextual caller
*/
Callable contextualize(Callable caller);
/**
* Decorates the specified supplier with a given context.
* @param the return type
* @param supplier a supplier task
* @return a contextual supplier
*/
Supplier contextualize(Supplier supplier);
/**
* Decorates the specified supplier with a given context.
* @param the return type
* @param the exception type
* @param supplier a supplier task
* @return a contextual supplier
*/
ExceptionSupplier contextualize(ExceptionSupplier supplier);
/**
* Decorates the specified consumer with a given context.
* @param the consumed value type
* @param consumer a consumer
* @return a contextual consumer
*/
Consumer contextualize(Consumer consumer);
/**
* Decorates the specified consumer with a given context.
* @param the consumed value type
* @param the exception type
* @param consumer a consumer
* @return a contextual consumer
*/
ExceptionConsumer contextualize(ExceptionConsumer consumer);
/**
* Decorates the specified consumer with a given context.
* @param the 1st consumed value type
* @param the 2nd consumed value type
* @param consumer a consumer
* @return a contextual consumer
*/
BiConsumer contextualize(BiConsumer consumer);
/**
* Decorates the specified consumer with a given context.
* @param the 1st consumed value type
* @param the 2nd consumed value type
* @param the exception type
* @param consumer a consumer
* @return a contextual consumer
*/
ExceptionBiConsumer contextualize(ExceptionBiConsumer consumer);
/**
* Decorates the specified function with a given context.
* @param the function parameter type
* @param the function return type
* @param function a function
* @return a contextual function
*/
Function contextualize(Function function);
/**
* Decorates the specified function with a given context.
* @param the function parameter type
* @param the function return type
* @param the exception type
* @param function a function
* @return a contextual function
*/
ExceptionFunction contextualize(ExceptionFunction function);
/**
* Decorates the specified function with a given context.
* @param the 1st function parameter type
* @param the 2nd function parameter type
* @param the function return type
* @param function a function
* @return a contextual function
*/
BiFunction contextualize(BiFunction function);
/**
* Decorates the specified function with a given context.
* @param the 1st function parameter type
* @param the 2nd function parameter type
* @param the function return type
* @param the exception type
* @param function a function
* @return a contextual function
*/
ExceptionBiFunction contextualize(ExceptionBiFunction function);
Contextualizer NONE = new Contextualizer() {
@Override
public Runnable contextualize(Runnable runner) {
return runner;
}
@Override
public ExceptionRunnable contextualize(ExceptionRunnable runner) {
return runner;
}
@Override
public Callable contextualize(Callable caller) {
return caller;
}
@Override
public Supplier contextualize(Supplier supplier) {
return supplier;
}
@Override
public ExceptionSupplier contextualize(ExceptionSupplier supplier) {
return supplier;
}
@Override
public Consumer contextualize(Consumer consumer) {
return consumer;
}
@Override
public ExceptionConsumer contextualize(ExceptionConsumer consumer) {
return consumer;
}
@Override
public BiConsumer contextualize(BiConsumer consumer) {
return consumer;
}
@Override
public ExceptionBiConsumer contextualize(ExceptionBiConsumer consumer) {
return consumer;
}
@Override
public Function contextualize(Function function) {
return function;
}
@Override
public ExceptionFunction contextualize(ExceptionFunction function) {
return function;
}
@Override
public BiFunction contextualize(BiFunction function) {
return function;
}
@Override
public ExceptionBiFunction contextualize(ExceptionBiFunction function) {
return function;
}
};
static Contextualizer withContextProvider(Supplier provider) {
ContextualExecutor executor = ContextualExecutor.withContextProvider(provider);
return new Contextualizer() {
@Override
public Runnable contextualize(Runnable runner) {
return new Runnable() {
@Override
public void run() {
executor.execute(runner);
}
};
}
@Override
public ExceptionRunnable contextualize(ExceptionRunnable runner) {
return new ExceptionRunnable<>() {
@Override
public void run() throws E {
executor.execute(runner);
}
};
}
@Override
public Callable contextualize(Callable caller) {
return new Callable<>() {
@Override
public T call() throws Exception {
return executor.execute(caller);
}
};
}
@Override
public Supplier contextualize(Supplier supplier) {
return new Supplier<>() {
@Override
public T get() {
return executor.execute(supplier);
}
};
}
@Override
public ExceptionSupplier contextualize(ExceptionSupplier supplier) {
return new ExceptionSupplier<>() {
@Override
public T get() throws E {
return executor.execute(supplier);
}
};
}
@Override
public Consumer contextualize(Consumer consumer) {
return new Consumer<>() {
@Override
public void accept(V value) {
executor.execute(consumer, value);
}
};
}
@Override
public ExceptionConsumer contextualize(ExceptionConsumer consumer) {
return new ExceptionConsumer<>() {
@Override
public void accept(V value) throws E {
executor.execute(consumer, value);
}
};
}
@Override
public BiConsumer contextualize(BiConsumer consumer) {
return new BiConsumer<>() {
@Override
public void accept(V1 value1, V2 value2) {
executor.execute(consumer, value1, value2);
}
};
}
@Override
public ExceptionBiConsumer contextualize(ExceptionBiConsumer consumer) {
return new ExceptionBiConsumer<>() {
@Override
public void accept(V1 value1, V2 value2) throws E {
executor.execute(consumer, value1, value2);
}
};
}
@Override
public Function contextualize(Function function) {
return new Function<>() {
@Override
public R apply(V value) {
return executor.execute(function, value);
}
};
}
@Override
public ExceptionFunction contextualize(ExceptionFunction function) {
return new ExceptionFunction<>() {
@Override
public R apply(V value) throws E {
return executor.execute(function, value);
}
};
}
@Override
public BiFunction contextualize(BiFunction function) {
return new BiFunction<>() {
@Override
public R apply(V1 value1, V2 value2) {
return executor.execute(function, value1, value2);
}
};
}
@Override
public ExceptionBiFunction contextualize(ExceptionBiFunction function) {
return new ExceptionBiFunction<>() {
@Override
public R apply(V1 value1, V2 value2) throws E {
return executor.execute(function, value1, value2);
}
};
}
};
}
static Contextualizer composite(List contextualizers) {
return new Contextualizer() {
@Override
public Runnable contextualize(Runnable runner) {
Runnable result = runner;
for (Contextualizer contextualizer : contextualizers) {
result = contextualizer.contextualize(result);
}
return result;
}
@Override
public ExceptionRunnable contextualize(ExceptionRunnable runner) {
ExceptionRunnable result = runner;
for (Contextualizer contextualizer : contextualizers) {
result = contextualizer.contextualize(result);
}
return result;
}
@Override
public Callable contextualize(Callable caller) {
Callable result = caller;
for (Contextualizer contextualizer : contextualizers) {
result = contextualizer.contextualize(result);
}
return result;
}
@Override
public Supplier contextualize(Supplier supplier) {
Supplier result = supplier;
for (Contextualizer contextualizer : contextualizers) {
result = contextualizer.contextualize(result);
}
return result;
}
@Override
public ExceptionSupplier contextualize(ExceptionSupplier supplier) {
ExceptionSupplier result = supplier;
for (Contextualizer contextualizer : contextualizers) {
result = contextualizer.contextualize(result);
}
return result;
}
@Override
public Consumer contextualize(Consumer consumer) {
Consumer result = consumer;
for (Contextualizer contextualizer : contextualizers) {
result = contextualizer.contextualize(result);
}
return result;
}
@Override
public ExceptionConsumer contextualize(ExceptionConsumer consumer) {
ExceptionConsumer result = consumer;
for (Contextualizer contextualizer : contextualizers) {
result = contextualizer.contextualize(result);
}
return result;
}
@Override
public BiConsumer contextualize(BiConsumer consumer) {
BiConsumer result = consumer;
for (Contextualizer contextualizer : contextualizers) {
result = contextualizer.contextualize(result);
}
return result;
}
@Override
public ExceptionBiConsumer contextualize(ExceptionBiConsumer consumer) {
ExceptionBiConsumer result = consumer;
for (Contextualizer contextualizer : contextualizers) {
result = contextualizer.contextualize(result);
}
return result;
}
@Override
public Function contextualize(Function function) {
Function result = function;
for (Contextualizer contextualizer : contextualizers) {
result = contextualizer.contextualize(result);
}
return result;
}
@Override
public ExceptionFunction contextualize(ExceptionFunction function) {
ExceptionFunction result = function;
for (Contextualizer contextualizer : contextualizers) {
result = contextualizer.contextualize(result);
}
return result;
}
@Override
public BiFunction contextualize(BiFunction function) {
BiFunction result = function;
for (Contextualizer contextualizer : contextualizers) {
result = contextualizer.contextualize(result);
}
return result;
}
@Override
public ExceptionBiFunction contextualize(ExceptionBiFunction function) {
ExceptionBiFunction result = function;
for (Contextualizer contextualizer : contextualizers) {
result = contextualizer.contextualize(result);
}
return result;
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy