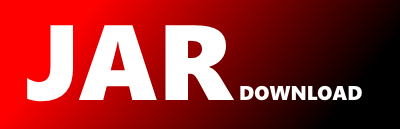
org.wildfly.clustering.context.ContextualExecutor Maven / Gradle / Ivy
/*
* Copyright The WildFly Authors
* SPDX-License-Identifier: Apache-2.0
*/
package org.wildfly.clustering.context;
import java.util.concurrent.Callable;
import java.util.concurrent.Executor;
import java.util.function.BiConsumer;
import java.util.function.BiFunction;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Supplier;
import org.wildfly.common.function.ExceptionBiConsumer;
import org.wildfly.common.function.ExceptionBiFunction;
import org.wildfly.common.function.ExceptionConsumer;
import org.wildfly.common.function.ExceptionFunction;
import org.wildfly.common.function.ExceptionRunnable;
import org.wildfly.common.function.ExceptionSupplier;
/**
* Facility for contextual execution.
* @author Paul Ferraro
*/
public interface ContextualExecutor extends Executor {
@Override
default void execute(Runnable runner) {
ExceptionRunnable r = runner::run;
this.execute(r);
}
/**
* Executes the specified runner.
* @param the exception type
* @param runner a runnable task
* @throws E if execution fails
*/
void execute(ExceptionRunnable runner) throws E;
/**
* Executes the specified consumer using the specified value.
* @param the consumed value type
* @param consumer a consumer
* @param value the consumed value
*/
default void execute(Consumer consumer, V value) {
ExceptionConsumer c = consumer::accept;
this.execute(c, value);
}
/**
* Executes the specified consumer using the specified value.
* @param the consumed value type
* @param the exception type
* @param consumer a consumer
* @param value the consumed value
* @throws E if execution fails
*/
void execute(ExceptionConsumer consumer, V value) throws E;
/**
* Executes the specified runner.
* @param the 1st consumed value type
* @param the 2nd consumed value type
* @param consumer a consumer
* @param value1 the 1st consumed value
* @param value2 the 2nd consumed value
*/
default void execute(BiConsumer consumer, V1 value1, V2 value2) {
ExceptionBiConsumer c = consumer::accept;
this.execute(c, value1, value2);
}
/**
* Executes the specified runner.
* @param the 1st consumed value type
* @param the 2nd consumed value type
* @param the exception type
* @param consumer a consumer
* @param value1 the 1st consumed value
* @param value2 the 2nd consumed value
* @throws E if execution fails
*/
void execute(ExceptionBiConsumer consumer, T value1, V value2) throws E;
/**
* Executes the specified caller with a given context.
* @param the return type
* @param caller a callable task
* @return the result of the caller
* @throws Exception if execution fails
*/
default T execute(Callable caller) throws Exception {
ExceptionSupplier supplier = caller::call;
return this.execute(supplier);
}
/**
* Executes the specified supplier with a given context.
* @param the return type
* @param supplier a supplier task
* @return the result of the supplier
*/
default T execute(Supplier supplier) {
ExceptionSupplier s = supplier::get;
return this.execute(s);
}
/**
* Executes the specified supplier with a given context.
* @param the return type
* @param the exception type
* @param supplier a supplier task
* @return the result of the supplier
* @throws E if execution fails
*/
T execute(ExceptionSupplier supplier) throws E;
/**
* Executes the specified supplier with a given context.
* @param the function parameter type
* @param the function return type
* @param function a function to apply
* @param value the function parameter
* @return the result of the function
*/
default R execute(Function function, V value) {
ExceptionFunction f = function::apply;
return this.execute(f, value);
}
/**
* Executes the specified supplier with a given context.
* @param the function parameter type
* @param the function return type
* @param the exception type
* @param function a function to apply
* @param value the function parameter
* @return the result of the function
* @throws E if execution fails
*/
R execute(ExceptionFunction function, V value) throws E;
/**
* Executes the specified supplier with a given context.
* @param the 1st function parameter type
* @param the 2nd function parameter type
* @param the function return type
* @param function a function to apply
* @param value1 the 1st function parameter
* @param value2 the 2nd function parameter
* @return the result of the function
*/
default R execute(BiFunction function, V1 value1, V2 value2) {
ExceptionBiFunction f = function::apply;
return this.execute(f, value1, value2);
}
/**
* Executes the specified supplier with a given context.
* @param the 1st function parameter type
* @param the 2nd function parameter type
* @param the function return type
* @param the exception type
* @param function a function to apply
* @param value1 the 1st function parameter
* @param value2 the 2nd function parameter
* @return the result of the function
* @throws E if execution fails
*/
R execute(ExceptionBiFunction function, V1 value1, V2 value2) throws E;
static ContextualExecutor withContextProvider(Supplier provider) {
return new ContextualExecutor() {
@Override
public void execute(ExceptionRunnable runner) throws E {
try (Context context = provider.get()) {
runner.run();
}
}
@Override
public void execute(ExceptionConsumer consumer, V value) throws E {
try (Context context = provider.get()) {
consumer.accept(value);
}
}
@Override
public void execute(ExceptionBiConsumer consumer, T value1, V value2) throws E {
try (Context context = provider.get()) {
consumer.accept(value1, value2);
}
}
@Override
public T execute(ExceptionSupplier supplier) throws E {
try (Context context = provider.get()) {
return supplier.get();
}
}
@Override
public R execute(ExceptionFunction function, V value) throws E {
try (Context context = provider.get()) {
return function.apply(value);
}
}
@Override
public R execute(ExceptionBiFunction function, V1 value1, V2 value2) throws E {
try (Context context = provider.get()) {
return function.apply(value1, value2);
}
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy