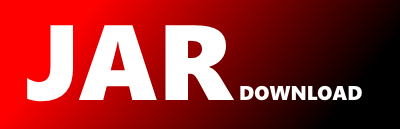
org.wildfly.swarm.config.datasources.Datasources Maven / Gradle / Ivy
package org.wildfly.swarm.config.datasources;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.Address;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.List;
import java.util.Map;
import org.wildfly.config.runtime.Subresource;
import org.wildfly.swarm.config.datasources.subsystem.jdbcDriver.JdbcDriver;
import org.wildfly.swarm.config.datasources.subsystem.xaDataSource.XaDataSource;
import org.wildfly.swarm.config.datasources.subsystem.dataSource.DataSource;
/**
* The data-sources subsystem, used to declare JDBC data-sources
*/
@Address("/subsystem=datasources")
@Implicit
public class Datasources {
private String key;
private List installedDrivers;
private DatasourcesResources subresources = new DatasourcesResources();
public Datasources() {
this.key = "datasources";
}
public String getKey() {
return this.key;
}
/**
* List of JDBC drivers that have been installed in the runtime
*/
@ModelNodeBinding(detypedName = "installed-drivers")
public List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy