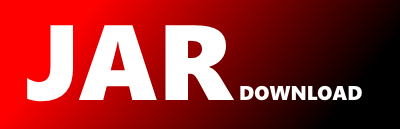
org.wildfly.swarm.config.ee.Ee Maven / Gradle / Ivy
package org.wildfly.swarm.config.ee;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.Address;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.List;
import java.util.Map;
import org.wildfly.config.runtime.Subresource;
import org.wildfly.swarm.config.ee.subsystem.managedThreadFactory.ManagedThreadFactory;
import org.wildfly.swarm.config.ee.subsystem.contextService.ContextService;
import org.wildfly.swarm.config.ee.subsystem.managedExecutorService.ManagedExecutorService;
import org.wildfly.swarm.config.ee.subsystem.managedScheduledExecutorService.ManagedScheduledExecutorService;
import org.wildfly.swarm.config.ee.subsystem.service.DefaultBindings;
/**
* The configuration of the EE subsystem.
*/
@Address("/subsystem=ee")
@Implicit
public class Ee {
private String key;
private Boolean annotationPropertyReplacement;
private Boolean earSubdeploymentsIsolated;
private List globalModules;
private Boolean jbossDescriptorPropertyReplacement;
private Boolean specDescriptorPropertyReplacement;
private EeResources subresources = new EeResources();
private DefaultBindings defaultBindings;
public Ee() {
this.key = "ee";
}
public String getKey() {
return this.key;
}
/**
* Flag indicating whether Java EE annotations will have property replacements applied
*/
@ModelNodeBinding(detypedName = "annotation-property-replacement")
public Boolean annotationPropertyReplacement() {
return this.annotationPropertyReplacement;
}
/**
* Flag indicating whether Java EE annotations will have property replacements applied
*/
@SuppressWarnings("unchecked")
public T annotationPropertyReplacement(Boolean value) {
this.annotationPropertyReplacement = value;
return (T) this;
}
/**
* Flag indicating whether each of the subdeployments within a .ear can access classes belonging to another subdeployment within the same .ear. A value of false means the subdeployments can see classes belonging to other subdeployments within the .ear.
*/
@ModelNodeBinding(detypedName = "ear-subdeployments-isolated")
public Boolean earSubdeploymentsIsolated() {
return this.earSubdeploymentsIsolated;
}
/**
* Flag indicating whether each of the subdeployments within a .ear can access classes belonging to another subdeployment within the same .ear. A value of false means the subdeployments can see classes belonging to other subdeployments within the .ear.
*/
@SuppressWarnings("unchecked")
public T earSubdeploymentsIsolated(Boolean value) {
this.earSubdeploymentsIsolated = value;
return (T) this;
}
/**
* A list of modules that should be made available to all deployments.
*/
@ModelNodeBinding(detypedName = "global-modules")
public List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy