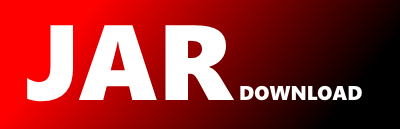
org.wildfly.swarm.config.jca.Jca Maven / Gradle / Ivy
package org.wildfly.swarm.config.jca;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.Address;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.List;
import org.wildfly.config.runtime.Subresource;
import org.wildfly.swarm.config.jca.subsystem.distributedWorkmanager.DistributedWorkmanager;
import org.wildfly.swarm.config.jca.subsystem.workmanager.Workmanager;
import org.wildfly.swarm.config.jca.subsystem.bootstrapContext.BootstrapContext;
import org.wildfly.swarm.config.jca.subsystem.cachedConnectionManager.CachedConnectionManager;
import org.wildfly.swarm.config.jca.subsystem.beanValidation.BeanValidation;
import org.wildfly.swarm.config.jca.subsystem.tracer.Tracer;
import org.wildfly.swarm.config.jca.subsystem.archiveValidation.ArchiveValidation;
/**
* The Java EE Connector Architecture (JCA) subsystem providing general configuration for resource adapters
*/
@Address("/subsystem=jca")
@Implicit
public class Jca {
private String key;
private JcaResources subresources = new JcaResources();
private CachedConnectionManager cachedConnectionManager;
private BeanValidation beanValidation;
private Tracer tracer;
private ArchiveValidation archiveValidation;
public Jca() {
this.key = "jca";
}
public String getKey() {
return this.key;
}
public JcaResources subresources() {
return this.subresources;
}
/**
* Add all DistributedWorkmanager objects to this subresource
* @return this
* @param value List of DistributedWorkmanager objects.
*/
@SuppressWarnings("unchecked")
public T distributedWorkmanagers(List value) {
this.subresources.distributedWorkmanagers.addAll(value);
return (T) this;
}
/**
* Add the DistributedWorkmanager object to the list of subresources
* @param value The DistributedWorkmanager to add
* @return this
*/
@SuppressWarnings("unchecked")
public T distributedWorkmanager(DistributedWorkmanager value) {
this.subresources.distributedWorkmanagers.add(value);
return (T) this;
}
/**
* Add all Workmanager objects to this subresource
* @return this
* @param value List of Workmanager objects.
*/
@SuppressWarnings("unchecked")
public T workmanagers(List value) {
this.subresources.workmanagers.addAll(value);
return (T) this;
}
/**
* Add the Workmanager object to the list of subresources
* @param value The Workmanager to add
* @return this
*/
@SuppressWarnings("unchecked")
public T workmanager(Workmanager value) {
this.subresources.workmanagers.add(value);
return (T) this;
}
/**
* Add all BootstrapContext objects to this subresource
* @return this
* @param value List of BootstrapContext objects.
*/
@SuppressWarnings("unchecked")
public T bootstrapContexts(List value) {
this.subresources.bootstrapContexts.addAll(value);
return (T) this;
}
/**
* Add the BootstrapContext object to the list of subresources
* @param value The BootstrapContext to add
* @return this
*/
@SuppressWarnings("unchecked")
public T bootstrapContext(BootstrapContext value) {
this.subresources.bootstrapContexts.add(value);
return (T) this;
}
/**
* Child mutators for Jca
*/
public class JcaResources {
/**
* DistributedWorkManager for resource adapters
*/
private List distributedWorkmanagers = new java.util.ArrayList<>();
/**
* WorkManager for resource adapters
*/
private List workmanagers = new java.util.ArrayList<>();
/**
* Bootstrap context for resource adapters
*/
private List bootstrapContexts = new java.util.ArrayList<>();
/**
* Get the list of DistributedWorkmanager resources
* @return the list of resources
*/
@Subresource
public List distributedWorkmanagers() {
return this.distributedWorkmanagers;
}
/**
* Get the list of Workmanager resources
* @return the list of resources
*/
@Subresource
public List workmanagers() {
return this.workmanagers;
}
/**
* Get the list of BootstrapContext resources
* @return the list of resources
*/
@Subresource
public List bootstrapContexts() {
return this.bootstrapContexts;
}
}
/**
* Cached connection manager for resource adapters
*/
@Subresource
public CachedConnectionManager cachedConnectionManager() {
return this.cachedConnectionManager;
}
/**
* Cached connection manager for resource adapters
*/
@SuppressWarnings("unchecked")
public T cachedConnectionManager(CachedConnectionManager value) {
this.cachedConnectionManager = value;
return (T) this;
}
/**
* Bean validation (JSR-303) for resource adapters
*/
@Subresource
public BeanValidation beanValidation() {
return this.beanValidation;
}
/**
* Bean validation (JSR-303) for resource adapters
*/
@SuppressWarnings("unchecked")
public T beanValidation(BeanValidation value) {
this.beanValidation = value;
return (T) this;
}
/**
* Tracer for resource adapters
*/
@Subresource
public Tracer tracer() {
return this.tracer;
}
/**
* Tracer for resource adapters
*/
@SuppressWarnings("unchecked")
public T tracer(Tracer value) {
this.tracer = value;
return (T) this;
}
/**
* Archive validation for resource adapters
*/
@Subresource
public ArchiveValidation archiveValidation() {
return this.archiveValidation;
}
/**
* Archive validation for resource adapters
*/
@SuppressWarnings("unchecked")
public T archiveValidation(ArchiveValidation value) {
this.archiveValidation = value;
return (T) this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy