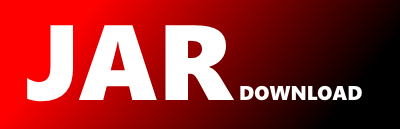
org.wildfly.swarm.config.remoting.Remoting Maven / Gradle / Ivy
package org.wildfly.swarm.config.remoting;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.Address;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.List;
import org.wildfly.config.runtime.Subresource;
import org.wildfly.swarm.config.remoting.subsystem.httpConnector.HttpConnector;
import org.wildfly.swarm.config.remoting.subsystem.connector.Connector;
import org.wildfly.swarm.config.remoting.subsystem.outboundConnection.OutboundConnection;
import org.wildfly.swarm.config.remoting.subsystem.localOutboundConnection.LocalOutboundConnection;
import org.wildfly.swarm.config.remoting.subsystem.remoteOutboundConnection.RemoteOutboundConnection;
import org.wildfly.swarm.config.remoting.subsystem.configuration.Endpoint;
/**
* The configuration of the Remoting subsystem.
*/
@Address("/subsystem=remoting")
@Implicit
public class Remoting {
private String key;
private Integer workerReadThreads;
private Integer workerTaskCoreThreads;
private Integer workerTaskKeepalive;
private Integer workerTaskLimit;
private Integer workerTaskMaxThreads;
private Integer workerWriteThreads;
private RemotingResources subresources = new RemotingResources();
private Endpoint endpoint;
public Remoting() {
this.key = "remoting";
}
public String getKey() {
return this.key;
}
/**
* The number of read threads to create for the remoting worker.
*/
@ModelNodeBinding(detypedName = "worker-read-threads")
public Integer workerReadThreads() {
return this.workerReadThreads;
}
/**
* The number of read threads to create for the remoting worker.
*/
@SuppressWarnings("unchecked")
public T workerReadThreads(Integer value) {
this.workerReadThreads = value;
return (T) this;
}
/**
* The number of core threads for the remoting worker task thread pool.
*/
@ModelNodeBinding(detypedName = "worker-task-core-threads")
public Integer workerTaskCoreThreads() {
return this.workerTaskCoreThreads;
}
/**
* The number of core threads for the remoting worker task thread pool.
*/
@SuppressWarnings("unchecked")
public T workerTaskCoreThreads(Integer value) {
this.workerTaskCoreThreads = value;
return (T) this;
}
/**
* The number of milliseconds to keep non-core remoting worker task threads alive.
*/
@ModelNodeBinding(detypedName = "worker-task-keepalive")
public Integer workerTaskKeepalive() {
return this.workerTaskKeepalive;
}
/**
* The number of milliseconds to keep non-core remoting worker task threads alive.
*/
@SuppressWarnings("unchecked")
public T workerTaskKeepalive(Integer value) {
this.workerTaskKeepalive = value;
return (T) this;
}
/**
* The maximum number of remoting worker tasks to allow before rejecting.
*/
@ModelNodeBinding(detypedName = "worker-task-limit")
public Integer workerTaskLimit() {
return this.workerTaskLimit;
}
/**
* The maximum number of remoting worker tasks to allow before rejecting.
*/
@SuppressWarnings("unchecked")
public T workerTaskLimit(Integer value) {
this.workerTaskLimit = value;
return (T) this;
}
/**
* The maximum number of threads for the remoting worker task thread pool.
*/
@ModelNodeBinding(detypedName = "worker-task-max-threads")
public Integer workerTaskMaxThreads() {
return this.workerTaskMaxThreads;
}
/**
* The maximum number of threads for the remoting worker task thread pool.
*/
@SuppressWarnings("unchecked")
public T workerTaskMaxThreads(Integer value) {
this.workerTaskMaxThreads = value;
return (T) this;
}
/**
* The number of write threads to create for the remoting worker.
*/
@ModelNodeBinding(detypedName = "worker-write-threads")
public Integer workerWriteThreads() {
return this.workerWriteThreads;
}
/**
* The number of write threads to create for the remoting worker.
*/
@SuppressWarnings("unchecked")
public T workerWriteThreads(Integer value) {
this.workerWriteThreads = value;
return (T) this;
}
public RemotingResources subresources() {
return this.subresources;
}
/**
* Add all HttpConnector objects to this subresource
* @return this
* @param value List of HttpConnector objects.
*/
@SuppressWarnings("unchecked")
public T httpConnectors(List value) {
this.subresources.httpConnectors.addAll(value);
return (T) this;
}
/**
* Add the HttpConnector object to the list of subresources
* @param value The HttpConnector to add
* @return this
*/
@SuppressWarnings("unchecked")
public T httpConnector(HttpConnector value) {
this.subresources.httpConnectors.add(value);
return (T) this;
}
/**
* Add all Connector objects to this subresource
* @return this
* @param value List of Connector objects.
*/
@SuppressWarnings("unchecked")
public T connectors(List value) {
this.subresources.connectors.addAll(value);
return (T) this;
}
/**
* Add the Connector object to the list of subresources
* @param value The Connector to add
* @return this
*/
@SuppressWarnings("unchecked")
public T connector(Connector value) {
this.subresources.connectors.add(value);
return (T) this;
}
/**
* Add all OutboundConnection objects to this subresource
* @return this
* @param value List of OutboundConnection objects.
*/
@SuppressWarnings("unchecked")
public T outboundConnections(List value) {
this.subresources.outboundConnections.addAll(value);
return (T) this;
}
/**
* Add the OutboundConnection object to the list of subresources
* @param value The OutboundConnection to add
* @return this
*/
@SuppressWarnings("unchecked")
public T outboundConnection(OutboundConnection value) {
this.subresources.outboundConnections.add(value);
return (T) this;
}
/**
* Add all LocalOutboundConnection objects to this subresource
* @return this
* @param value List of LocalOutboundConnection objects.
*/
@SuppressWarnings("unchecked")
public T localOutboundConnections(List value) {
this.subresources.localOutboundConnections.addAll(value);
return (T) this;
}
/**
* Add the LocalOutboundConnection object to the list of subresources
* @param value The LocalOutboundConnection to add
* @return this
*/
@SuppressWarnings("unchecked")
public T localOutboundConnection(LocalOutboundConnection value) {
this.subresources.localOutboundConnections.add(value);
return (T) this;
}
/**
* Add all RemoteOutboundConnection objects to this subresource
* @return this
* @param value List of RemoteOutboundConnection objects.
*/
@SuppressWarnings("unchecked")
public T remoteOutboundConnections(List value) {
this.subresources.remoteOutboundConnections.addAll(value);
return (T) this;
}
/**
* Add the RemoteOutboundConnection object to the list of subresources
* @param value The RemoteOutboundConnection to add
* @return this
*/
@SuppressWarnings("unchecked")
public T remoteOutboundConnection(RemoteOutboundConnection value) {
this.subresources.remoteOutboundConnections.add(value);
return (T) this;
}
/**
* Child mutators for Remoting
*/
public class RemotingResources {
/**
* The configuration of a HTTP Upgrade based Remoting connector.
*/
private List httpConnectors = new java.util.ArrayList<>();
/**
* The configuration of a Remoting connector.
*/
private List connectors = new java.util.ArrayList<>();
/**
* Remoting outbound connection.
*/
private List outboundConnections = new java.util.ArrayList<>();
/**
* Remoting outbound connection with an implicit local:// URI scheme.
*/
private List localOutboundConnections = new java.util.ArrayList<>();
/**
* Remoting outbound connection with an implicit remote:// URI scheme.
*/
private List remoteOutboundConnections = new java.util.ArrayList<>();
/**
* Get the list of HttpConnector resources
* @return the list of resources
*/
@Subresource
public List httpConnectors() {
return this.httpConnectors;
}
/**
* Get the list of Connector resources
* @return the list of resources
*/
@Subresource
public List connectors() {
return this.connectors;
}
/**
* Get the list of OutboundConnection resources
* @return the list of resources
*/
@Subresource
public List outboundConnections() {
return this.outboundConnections;
}
/**
* Get the list of LocalOutboundConnection resources
* @return the list of resources
*/
@Subresource
public List localOutboundConnections() {
return this.localOutboundConnections;
}
/**
* Get the list of RemoteOutboundConnection resources
* @return the list of resources
*/
@Subresource
public List remoteOutboundConnections() {
return this.remoteOutboundConnections;
}
}
/**
* Endpoint configuration
*/
@Subresource
public Endpoint endpoint() {
return this.endpoint;
}
/**
* Endpoint configuration
*/
@SuppressWarnings("unchecked")
public T endpoint(Endpoint value) {
this.endpoint = value;
return (T) this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy