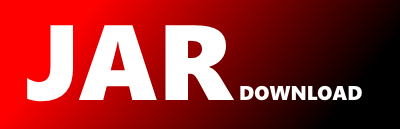
org.wildfly.swarm.config.transactions.Transactions Maven / Gradle / Ivy
package org.wildfly.swarm.config.transactions;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.Address;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.List;
import org.wildfly.config.runtime.Subresource;
import org.wildfly.swarm.config.transactions.subsystem.commitMarkableResource.CommitMarkableResource;
import org.wildfly.swarm.config.transactions.subsystem.logStore.LogStore;
/**
* The configuration of the transaction subsystem.
*/
@Address("/subsystem=transactions")
@Implicit
public class Transactions {
private String key;
private Integer defaultTimeout;
private Boolean enableStatistics;
private Boolean enableTsmStatus;
private Boolean hornetqStoreEnableAsyncIo;
private Boolean jdbcActionStoreDropTable;
private String jdbcActionStoreTablePrefix;
private Boolean jdbcCommunicationStoreDropTable;
private String jdbcCommunicationStoreTablePrefix;
private Boolean jdbcStateStoreDropTable;
private String jdbcStateStoreTablePrefix;
private String jdbcStoreDatasource;
private Boolean journalStoreEnableAsyncIo;
private Boolean jts;
private String nodeIdentifier;
private Long numberOfAbortedTransactions;
private Long numberOfApplicationRollbacks;
private Long numberOfCommittedTransactions;
private Long numberOfHeuristics;
private Long numberOfInflightTransactions;
private Long numberOfNestedTransactions;
private Long numberOfResourceRollbacks;
private Long numberOfTimedOutTransactions;
private Long numberOfTransactions;
private String objectStorePath;
private String objectStoreRelativeTo;
private String processIdSocketBinding;
private Integer processIdSocketMaxPorts;
private Boolean processIdUuid;
private Boolean recoveryListener;
private String socketBinding;
private Boolean statisticsEnabled;
private String statusSocketBinding;
private Boolean useHornetqStore;
private Boolean useJdbcStore;
private Boolean useJournalStore;
private TransactionsResources subresources = new TransactionsResources();
private LogStore logStore;
public Transactions() {
this.key = "transactions";
}
public String getKey() {
return this.key;
}
/**
* The default timeout.
*/
@ModelNodeBinding(detypedName = "default-timeout")
public Integer defaultTimeout() {
return this.defaultTimeout;
}
/**
* The default timeout.
*/
@SuppressWarnings("unchecked")
public T defaultTimeout(Integer value) {
this.defaultTimeout = value;
return (T) this;
}
/**
* Whether statistics should be enabled.
*/
@ModelNodeBinding(detypedName = "enable-statistics")
public Boolean enableStatistics() {
return this.enableStatistics;
}
/**
* Whether statistics should be enabled.
*/
@SuppressWarnings("unchecked")
public T enableStatistics(Boolean value) {
this.enableStatistics = value;
return (T) this;
}
/**
* Whether the transaction status manager (TSM) service, needed for out of process recovery, should be provided or not..
*/
@ModelNodeBinding(detypedName = "enable-tsm-status")
public Boolean enableTsmStatus() {
return this.enableTsmStatus;
}
/**
* Whether the transaction status manager (TSM) service, needed for out of process recovery, should be provided or not..
*/
@SuppressWarnings("unchecked")
public T enableTsmStatus(Boolean value) {
this.enableTsmStatus = value;
return (T) this;
}
/**
* Whether AsyncIO should be enabled for the hornetq store. Default is false. The server should be restarted for this setting to take effect.
*/
@ModelNodeBinding(detypedName = "hornetq-store-enable-async-io")
public Boolean hornetqStoreEnableAsyncIo() {
return this.hornetqStoreEnableAsyncIo;
}
/**
* Whether AsyncIO should be enabled for the hornetq store. Default is false. The server should be restarted for this setting to take effect.
*/
@SuppressWarnings("unchecked")
public T hornetqStoreEnableAsyncIo(Boolean value) {
this.hornetqStoreEnableAsyncIo = value;
return (T) this;
}
/**
* Configure if jdbc action store should drop tables. Default is false. The server should be restarted for this setting to take effect.
*/
@ModelNodeBinding(detypedName = "jdbc-action-store-drop-table")
public Boolean jdbcActionStoreDropTable() {
return this.jdbcActionStoreDropTable;
}
/**
* Configure if jdbc action store should drop tables. Default is false. The server should be restarted for this setting to take effect.
*/
@SuppressWarnings("unchecked")
public T jdbcActionStoreDropTable(Boolean value) {
this.jdbcActionStoreDropTable = value;
return (T) this;
}
/**
* Optional prefix for table used to write transcation logs in configured jdbc action store. The server should be restarted for this setting to take effect.
*/
@ModelNodeBinding(detypedName = "jdbc-action-store-table-prefix")
public String jdbcActionStoreTablePrefix() {
return this.jdbcActionStoreTablePrefix;
}
/**
* Optional prefix for table used to write transcation logs in configured jdbc action store. The server should be restarted for this setting to take effect.
*/
@SuppressWarnings("unchecked")
public T jdbcActionStoreTablePrefix(String value) {
this.jdbcActionStoreTablePrefix = value;
return (T) this;
}
/**
* Configure if jdbc communication store should drop tables. Default is false. The server should be restarted for this setting to take effect.
*/
@ModelNodeBinding(detypedName = "jdbc-communication-store-drop-table")
public Boolean jdbcCommunicationStoreDropTable() {
return this.jdbcCommunicationStoreDropTable;
}
/**
* Configure if jdbc communication store should drop tables. Default is false. The server should be restarted for this setting to take effect.
*/
@SuppressWarnings("unchecked")
public T jdbcCommunicationStoreDropTable(Boolean value) {
this.jdbcCommunicationStoreDropTable = value;
return (T) this;
}
/**
* Optional prefix for table used to write transcation logs in configured jdbc communication store. The server should be restarted for this setting to take effect.
*/
@ModelNodeBinding(detypedName = "jdbc-communication-store-table-prefix")
public String jdbcCommunicationStoreTablePrefix() {
return this.jdbcCommunicationStoreTablePrefix;
}
/**
* Optional prefix for table used to write transcation logs in configured jdbc communication store. The server should be restarted for this setting to take effect.
*/
@SuppressWarnings("unchecked")
public T jdbcCommunicationStoreTablePrefix(String value) {
this.jdbcCommunicationStoreTablePrefix = value;
return (T) this;
}
/**
* Configure if jdbc state store should drop tables. Default is false. The server should be restarted for this setting to take effect.
*/
@ModelNodeBinding(detypedName = "jdbc-state-store-drop-table")
public Boolean jdbcStateStoreDropTable() {
return this.jdbcStateStoreDropTable;
}
/**
* Configure if jdbc state store should drop tables. Default is false. The server should be restarted for this setting to take effect.
*/
@SuppressWarnings("unchecked")
public T jdbcStateStoreDropTable(Boolean value) {
this.jdbcStateStoreDropTable = value;
return (T) this;
}
/**
* Optional prefix for table used to write transcation logs in configured jdbc state store. The server should be restarted for this setting to take effect.
*/
@ModelNodeBinding(detypedName = "jdbc-state-store-table-prefix")
public String jdbcStateStoreTablePrefix() {
return this.jdbcStateStoreTablePrefix;
}
/**
* Optional prefix for table used to write transcation logs in configured jdbc state store. The server should be restarted for this setting to take effect.
*/
@SuppressWarnings("unchecked")
public T jdbcStateStoreTablePrefix(String value) {
this.jdbcStateStoreTablePrefix = value;
return (T) this;
}
/**
* Jndi name of non-XA datasource used. Datasource sghould be define in datasources subsystem. The server should be restarted for this setting to take effect.
*/
@ModelNodeBinding(detypedName = "jdbc-store-datasource")
public String jdbcStoreDatasource() {
return this.jdbcStoreDatasource;
}
/**
* Jndi name of non-XA datasource used. Datasource sghould be define in datasources subsystem. The server should be restarted for this setting to take effect.
*/
@SuppressWarnings("unchecked")
public T jdbcStoreDatasource(String value) {
this.jdbcStoreDatasource = value;
return (T) this;
}
/**
* Whether AsyncIO should be enabled for the journal store. Default is false. The server should be restarted for this setting to take effect.
*/
@ModelNodeBinding(detypedName = "journal-store-enable-async-io")
public Boolean journalStoreEnableAsyncIo() {
return this.journalStoreEnableAsyncIo;
}
/**
* Whether AsyncIO should be enabled for the journal store. Default is false. The server should be restarted for this setting to take effect.
*/
@SuppressWarnings("unchecked")
public T journalStoreEnableAsyncIo(Boolean value) {
this.journalStoreEnableAsyncIo = value;
return (T) this;
}
/**
* If true this enables the Java Transaction Service. NOTE: use of JTS requires configuration of the JacORB subsystem.
*/
@ModelNodeBinding(detypedName = "jts")
public Boolean jts() {
return this.jts;
}
/**
* If true this enables the Java Transaction Service. NOTE: use of JTS requires configuration of the JacORB subsystem.
*/
@SuppressWarnings("unchecked")
public T jts(Boolean value) {
this.jts = value;
return (T) this;
}
/**
* Used to set the node identifier on the core environment.
*/
@ModelNodeBinding(detypedName = "node-identifier")
public String nodeIdentifier() {
return this.nodeIdentifier;
}
/**
* Used to set the node identifier on the core environment.
*/
@SuppressWarnings("unchecked")
public T nodeIdentifier(String value) {
this.nodeIdentifier = value;
return (T) this;
}
/**
* The number of aborted (i.e. rolledback) transactions.
*/
@ModelNodeBinding(detypedName = "number-of-aborted-transactions")
public Long numberOfAbortedTransactions() {
return this.numberOfAbortedTransactions;
}
/**
* The number of aborted (i.e. rolledback) transactions.
*/
@SuppressWarnings("unchecked")
public T numberOfAbortedTransactions(Long value) {
this.numberOfAbortedTransactions = value;
return (T) this;
}
/**
* The number of transactions that have been rolled back by application request. This includes those that timeout, since the timeout behavior is considered an attribute of the application configuration.
*/
@ModelNodeBinding(detypedName = "number-of-application-rollbacks")
public Long numberOfApplicationRollbacks() {
return this.numberOfApplicationRollbacks;
}
/**
* The number of transactions that have been rolled back by application request. This includes those that timeout, since the timeout behavior is considered an attribute of the application configuration.
*/
@SuppressWarnings("unchecked")
public T numberOfApplicationRollbacks(Long value) {
this.numberOfApplicationRollbacks = value;
return (T) this;
}
/**
* The number of committed transactions.
*/
@ModelNodeBinding(detypedName = "number-of-committed-transactions")
public Long numberOfCommittedTransactions() {
return this.numberOfCommittedTransactions;
}
/**
* The number of committed transactions.
*/
@SuppressWarnings("unchecked")
public T numberOfCommittedTransactions(Long value) {
this.numberOfCommittedTransactions = value;
return (T) this;
}
/**
* The number of transactions which have terminated with heuristic outcomes.
*/
@ModelNodeBinding(detypedName = "number-of-heuristics")
public Long numberOfHeuristics() {
return this.numberOfHeuristics;
}
/**
* The number of transactions which have terminated with heuristic outcomes.
*/
@SuppressWarnings("unchecked")
public T numberOfHeuristics(Long value) {
this.numberOfHeuristics = value;
return (T) this;
}
/**
* The number of transactions that have begun but not yet terminated.
*/
@ModelNodeBinding(detypedName = "number-of-inflight-transactions")
public Long numberOfInflightTransactions() {
return this.numberOfInflightTransactions;
}
/**
* The number of transactions that have begun but not yet terminated.
*/
@SuppressWarnings("unchecked")
public T numberOfInflightTransactions(Long value) {
this.numberOfInflightTransactions = value;
return (T) this;
}
/**
* The total number of nested (sub) transactions created.
*/
@ModelNodeBinding(detypedName = "number-of-nested-transactions")
public Long numberOfNestedTransactions() {
return this.numberOfNestedTransactions;
}
/**
* The total number of nested (sub) transactions created.
*/
@SuppressWarnings("unchecked")
public T numberOfNestedTransactions(Long value) {
this.numberOfNestedTransactions = value;
return (T) this;
}
/**
* The number of transactions that rolled back due to resource (participant) failure.
*/
@ModelNodeBinding(detypedName = "number-of-resource-rollbacks")
public Long numberOfResourceRollbacks() {
return this.numberOfResourceRollbacks;
}
/**
* The number of transactions that rolled back due to resource (participant) failure.
*/
@SuppressWarnings("unchecked")
public T numberOfResourceRollbacks(Long value) {
this.numberOfResourceRollbacks = value;
return (T) this;
}
/**
* The number of transactions that have rolled back due to timeout.
*/
@ModelNodeBinding(detypedName = "number-of-timed-out-transactions")
public Long numberOfTimedOutTransactions() {
return this.numberOfTimedOutTransactions;
}
/**
* The number of transactions that have rolled back due to timeout.
*/
@SuppressWarnings("unchecked")
public T numberOfTimedOutTransactions(Long value) {
this.numberOfTimedOutTransactions = value;
return (T) this;
}
/**
* The total number of transactions (top-level and nested) created
*/
@ModelNodeBinding(detypedName = "number-of-transactions")
public Long numberOfTransactions() {
return this.numberOfTransactions;
}
/**
* The total number of transactions (top-level and nested) created
*/
@SuppressWarnings("unchecked")
public T numberOfTransactions(Long value) {
this.numberOfTransactions = value;
return (T) this;
}
/**
* Denotes a relative or absolute filesystem path denoting where the transaction manager object store should store data. By default the value is treated as relative to the path denoted by the "relative-to" attribute.
*/
@ModelNodeBinding(detypedName = "object-store-path")
public String objectStorePath() {
return this.objectStorePath;
}
/**
* Denotes a relative or absolute filesystem path denoting where the transaction manager object store should store data. By default the value is treated as relative to the path denoted by the "relative-to" attribute.
*/
@SuppressWarnings("unchecked")
public T objectStorePath(String value) {
this.objectStorePath = value;
return (T) this;
}
/**
* References a global path configuration in the domain model, defaulting to the JBoss Application Server data directory (jboss.server.data.dir). The value of the "path" attribute will treated as relative to this path. Use an empty string to disable the default behavior and force the value of the "path" attribute to be treated as an absolute path.
*/
@ModelNodeBinding(detypedName = "object-store-relative-to")
public String objectStoreRelativeTo() {
return this.objectStoreRelativeTo;
}
/**
* References a global path configuration in the domain model, defaulting to the JBoss Application Server data directory (jboss.server.data.dir). The value of the "path" attribute will treated as relative to this path. Use an empty string to disable the default behavior and force the value of the "path" attribute to be treated as an absolute path.
*/
@SuppressWarnings("unchecked")
public T objectStoreRelativeTo(String value) {
this.objectStoreRelativeTo = value;
return (T) this;
}
/**
* The name of the socket binding configuration to use if the transaction manager should use a socket-based process id. Will be 'undefined' if 'process-id-uuid' is 'true'; otherwise must be set.
*/
@ModelNodeBinding(detypedName = "process-id-socket-binding")
public String processIdSocketBinding() {
return this.processIdSocketBinding;
}
/**
* The name of the socket binding configuration to use if the transaction manager should use a socket-based process id. Will be 'undefined' if 'process-id-uuid' is 'true'; otherwise must be set.
*/
@SuppressWarnings("unchecked")
public T processIdSocketBinding(String value) {
this.processIdSocketBinding = value;
return (T) this;
}
/**
* The maximum number of ports to search for an open port if the transaction manager should use a socket-based process id. If the port specified by the socket binding referenced in 'process-id-socket-binding' is occupied, the next higher port will be tried until an open port is found or the number of ports specified by this attribute have been tried. Will be 'undefined' if 'process-id-uuid' is 'true'.
*/
@ModelNodeBinding(detypedName = "process-id-socket-max-ports")
public Integer processIdSocketMaxPorts() {
return this.processIdSocketMaxPorts;
}
/**
* The maximum number of ports to search for an open port if the transaction manager should use a socket-based process id. If the port specified by the socket binding referenced in 'process-id-socket-binding' is occupied, the next higher port will be tried until an open port is found or the number of ports specified by this attribute have been tried. Will be 'undefined' if 'process-id-uuid' is 'true'.
*/
@SuppressWarnings("unchecked")
public T processIdSocketMaxPorts(Integer value) {
this.processIdSocketMaxPorts = value;
return (T) this;
}
/**
* Indicates whether the transaction manager should use a UUID based process id.
*/
@ModelNodeBinding(detypedName = "process-id-uuid")
public Boolean processIdUuid() {
return this.processIdUuid;
}
/**
* Indicates whether the transaction manager should use a UUID based process id.
*/
@SuppressWarnings("unchecked")
public T processIdUuid(Boolean value) {
this.processIdUuid = value;
return (T) this;
}
/**
* Used to specify if the recovery system should listen on a network socket or not.
*/
@ModelNodeBinding(detypedName = "recovery-listener")
public Boolean recoveryListener() {
return this.recoveryListener;
}
/**
* Used to specify if the recovery system should listen on a network socket or not.
*/
@SuppressWarnings("unchecked")
public T recoveryListener(Boolean value) {
this.recoveryListener = value;
return (T) this;
}
/**
* Used to reference the correct socket binding to use for the recovery environment.
*/
@ModelNodeBinding(detypedName = "socket-binding")
public String socketBinding() {
return this.socketBinding;
}
/**
* Used to reference the correct socket binding to use for the recovery environment.
*/
@SuppressWarnings("unchecked")
public T socketBinding(String value) {
this.socketBinding = value;
return (T) this;
}
/**
* Whether statistics should be enabled.
*/
@ModelNodeBinding(detypedName = "statistics-enabled")
public Boolean statisticsEnabled() {
return this.statisticsEnabled;
}
/**
* Whether statistics should be enabled.
*/
@SuppressWarnings("unchecked")
public T statisticsEnabled(Boolean value) {
this.statisticsEnabled = value;
return (T) this;
}
/**
* Used to reference the correct socket binding to use for the transaction status manager.
*/
@ModelNodeBinding(detypedName = "status-socket-binding")
public String statusSocketBinding() {
return this.statusSocketBinding;
}
/**
* Used to reference the correct socket binding to use for the transaction status manager.
*/
@SuppressWarnings("unchecked")
public T statusSocketBinding(String value) {
this.statusSocketBinding = value;
return (T) this;
}
/**
* Use the hornetq store for writing transaction logs. Set to true to enable and to false to use the default log store type. The default log store is normally one file system file per transaction log. The server should be restarted for this setting to take effect. It's alternative to jdbc based store.
*/
@ModelNodeBinding(detypedName = "use-hornetq-store")
public Boolean useHornetqStore() {
return this.useHornetqStore;
}
/**
* Use the hornetq store for writing transaction logs. Set to true to enable and to false to use the default log store type. The default log store is normally one file system file per transaction log. The server should be restarted for this setting to take effect. It's alternative to jdbc based store.
*/
@SuppressWarnings("unchecked")
public T useHornetqStore(Boolean value) {
this.useHornetqStore = value;
return (T) this;
}
/**
* Use the jdbc store for writing transaction logs. Set to true to enable and to false to use the default log store type. The default log store is normally one file system file per transaction log. The server should be restarted for this setting to take effect. It's alternative to Horneq based store
*/
@ModelNodeBinding(detypedName = "use-jdbc-store")
public Boolean useJdbcStore() {
return this.useJdbcStore;
}
/**
* Use the jdbc store for writing transaction logs. Set to true to enable and to false to use the default log store type. The default log store is normally one file system file per transaction log. The server should be restarted for this setting to take effect. It's alternative to Horneq based store
*/
@SuppressWarnings("unchecked")
public T useJdbcStore(Boolean value) {
this.useJdbcStore = value;
return (T) this;
}
/**
* Use the journal store for writing transaction logs. Set to true to enable and to false to use the default log store type. The default log store is normally one file system file per transaction log. The server should be restarted for this setting to take effect. It's alternative to jdbc based store.
*/
@ModelNodeBinding(detypedName = "use-journal-store")
public Boolean useJournalStore() {
return this.useJournalStore;
}
/**
* Use the journal store for writing transaction logs. Set to true to enable and to false to use the default log store type. The default log store is normally one file system file per transaction log. The server should be restarted for this setting to take effect. It's alternative to jdbc based store.
*/
@SuppressWarnings("unchecked")
public T useJournalStore(Boolean value) {
this.useJournalStore = value;
return (T) this;
}
public TransactionsResources subresources() {
return this.subresources;
}
/**
* Add all CommitMarkableResource objects to this subresource
* @return this
* @param value List of CommitMarkableResource objects.
*/
@SuppressWarnings("unchecked")
public T commitMarkableResources(List value) {
this.subresources.commitMarkableResources.addAll(value);
return (T) this;
}
/**
* Add the CommitMarkableResource object to the list of subresources
* @param value The CommitMarkableResource to add
* @return this
*/
@SuppressWarnings("unchecked")
public T commitMarkableResource(CommitMarkableResource value) {
this.subresources.commitMarkableResources.add(value);
return (T) this;
}
/**
* Child mutators for Transactions
*/
public class TransactionsResources {
/**
* a CMR resource (i.e. a local resource that can reliably participate in an XA transaction)
*/
private List commitMarkableResources = new java.util.ArrayList<>();
/**
* Get the list of CommitMarkableResource resources
* @return the list of resources
*/
@Subresource
public List commitMarkableResources() {
return this.commitMarkableResources;
}
}
/**
* Representation of the transaction logging storage mechanism.
*/
@Subresource
public LogStore logStore() {
return this.logStore;
}
/**
* Representation of the transaction logging storage mechanism.
*/
@SuppressWarnings("unchecked")
public T logStore(LogStore value) {
this.logStore = value;
return (T) this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy