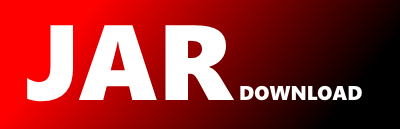
org.wildfly.swarm.config.undertow.Undertow Maven / Gradle / Ivy
package org.wildfly.swarm.config.undertow;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.Address;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.List;
import org.wildfly.config.runtime.Subresource;
import org.wildfly.swarm.config.undertow.subsystem.server.Server;
import org.wildfly.swarm.config.undertow.subsystem.bufferCache.BufferCache;
import org.wildfly.swarm.config.undertow.subsystem.servletContainer.ServletContainer;
import org.wildfly.swarm.config.undertow.subsystem.configuration.Handler;
import org.wildfly.swarm.config.undertow.subsystem.configuration.Filter;
/**
* Undertow subsystem
*/
@Address("/subsystem=undertow")
@Implicit
public class Undertow {
private String key;
private String defaultServer;
private String defaultServletContainer;
private String defaultVirtualHost;
private String instanceId;
private Boolean statisticsEnabled;
private UndertowResources subresources = new UndertowResources();
private Handler handler;
private Filter filter;
public Undertow() {
this.key = "undertow";
}
public String getKey() {
return this.key;
}
/**
* The default server to use for deployments
*/
@ModelNodeBinding(detypedName = "default-server")
public String defaultServer() {
return this.defaultServer;
}
/**
* The default server to use for deployments
*/
@SuppressWarnings("unchecked")
public T defaultServer(String value) {
this.defaultServer = value;
return (T) this;
}
/**
* The default servlet container to use for deployments
*/
@ModelNodeBinding(detypedName = "default-servlet-container")
public String defaultServletContainer() {
return this.defaultServletContainer;
}
/**
* The default servlet container to use for deployments
*/
@SuppressWarnings("unchecked")
public T defaultServletContainer(String value) {
this.defaultServletContainer = value;
return (T) this;
}
/**
* The default virtual host to use for deployments
*/
@ModelNodeBinding(detypedName = "default-virtual-host")
public String defaultVirtualHost() {
return this.defaultVirtualHost;
}
/**
* The default virtual host to use for deployments
*/
@SuppressWarnings("unchecked")
public T defaultVirtualHost(String value) {
this.defaultVirtualHost = value;
return (T) this;
}
/**
* The cluster instance id
*/
@ModelNodeBinding(detypedName = "instance-id")
public String instanceId() {
return this.instanceId;
}
/**
* The cluster instance id
*/
@SuppressWarnings("unchecked")
public T instanceId(String value) {
this.instanceId = value;
return (T) this;
}
/**
* Configures if are statistics enabled
*/
@ModelNodeBinding(detypedName = "statistics-enabled")
public Boolean statisticsEnabled() {
return this.statisticsEnabled;
}
/**
* Configures if are statistics enabled
*/
@SuppressWarnings("unchecked")
public T statisticsEnabled(Boolean value) {
this.statisticsEnabled = value;
return (T) this;
}
public UndertowResources subresources() {
return this.subresources;
}
/**
* Add all Server objects to this subresource
* @return this
* @param value List of Server objects.
*/
@SuppressWarnings("unchecked")
public T servers(List value) {
this.subresources.servers.addAll(value);
return (T) this;
}
/**
* Add the Server object to the list of subresources
* @param value The Server to add
* @return this
*/
@SuppressWarnings("unchecked")
public T server(Server value) {
this.subresources.servers.add(value);
return (T) this;
}
/**
* Add all BufferCache objects to this subresource
* @return this
* @param value List of BufferCache objects.
*/
@SuppressWarnings("unchecked")
public T bufferCaches(List value) {
this.subresources.bufferCaches.addAll(value);
return (T) this;
}
/**
* Add the BufferCache object to the list of subresources
* @param value The BufferCache to add
* @return this
*/
@SuppressWarnings("unchecked")
public T bufferCache(BufferCache value) {
this.subresources.bufferCaches.add(value);
return (T) this;
}
/**
* Add all ServletContainer objects to this subresource
* @return this
* @param value List of ServletContainer objects.
*/
@SuppressWarnings("unchecked")
public T servletContainers(List value) {
this.subresources.servletContainers.addAll(value);
return (T) this;
}
/**
* Add the ServletContainer object to the list of subresources
* @param value The ServletContainer to add
* @return this
*/
@SuppressWarnings("unchecked")
public T servletContainer(ServletContainer value) {
this.subresources.servletContainers.add(value);
return (T) this;
}
/**
* Child mutators for Undertow
*/
public class UndertowResources {
/**
* A server
*/
private List servers = new java.util.ArrayList<>();
/**
* The buffer cache used to cache static content
*/
private List bufferCaches = new java.util.ArrayList<>();
/**
* A servlet container
*/
private List servletContainers = new java.util.ArrayList<>();
/**
* Get the list of Server resources
* @return the list of resources
*/
@Subresource
public List servers() {
return this.servers;
}
/**
* Get the list of BufferCache resources
* @return the list of resources
*/
@Subresource
public List bufferCaches() {
return this.bufferCaches;
}
/**
* Get the list of ServletContainer resources
* @return the list of resources
*/
@Subresource
public List servletContainers() {
return this.servletContainers;
}
}
/**
* Undertow handlers
*/
@Subresource
public Handler handler() {
return this.handler;
}
/**
* Undertow handlers
*/
@SuppressWarnings("unchecked")
public T handler(Handler value) {
this.handler = value;
return (T) this;
}
/**
* Undertow filters
*/
@Subresource
public Filter filter() {
return this.filter;
}
/**
* Undertow filters
*/
@SuppressWarnings("unchecked")
public T filter(Filter value) {
this.filter = value;
return (T) this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy