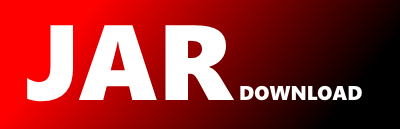
org.wildfly.swarm.config.Logging Maven / Gradle / Ivy
package org.wildfly.swarm.config;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.ResourceType;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.List;
import org.wildfly.config.runtime.Subresource;
import org.wildfly.swarm.config.logging.PeriodicRotatingFileHandler;
import org.wildfly.swarm.config.logging.Logger;
import org.wildfly.swarm.config.logging.AsyncHandler;
import org.wildfly.swarm.config.logging.FileHandler;
import org.wildfly.swarm.config.logging.SizeRotatingFileHandler;
import org.wildfly.swarm.config.logging.SyslogHandler;
import org.wildfly.swarm.config.logging.LoggingProfile;
import org.wildfly.swarm.config.logging.CustomFormatter;
import org.wildfly.swarm.config.logging.PeriodicSizeRotatingFileHandler;
import org.wildfly.swarm.config.logging.ConsoleHandler;
import org.wildfly.swarm.config.logging.LogFile;
import org.wildfly.swarm.config.logging.PatternFormatter;
import org.wildfly.swarm.config.logging.CustomHandler;
import org.wildfly.swarm.config.logging.RootLogger;
/**
* The configuration of the logging subsystem.
*/
@ResourceType("subsystem")
@Implicit
public class Logging {
private String key;
private Boolean addLoggingApiDependencies;
private Boolean useDeploymentLoggingConfig;
private LoggingResources subresources = new LoggingResources();
private RootLogger rootLogger;
public Logging() {
this.key = "logging";
}
public String getKey() {
return this.key;
}
/**
* Indicates whether or not logging API dependencies should be added to deployments during the deployment process. A value of true will add the dependencies to the deployment. A value of false will skip the deployment from being processed for logging API dependencies.
*/
@ModelNodeBinding(detypedName = "add-logging-api-dependencies")
public Boolean addLoggingApiDependencies() {
return this.addLoggingApiDependencies;
}
/**
* Indicates whether or not logging API dependencies should be added to deployments during the deployment process. A value of true will add the dependencies to the deployment. A value of false will skip the deployment from being processed for logging API dependencies.
*/
@SuppressWarnings("unchecked")
public T addLoggingApiDependencies(Boolean value) {
this.addLoggingApiDependencies = value;
return (T) this;
}
/**
* Indicates whether or not deployments should use a logging configuration file found in the deployment to configure the log manager. If set to true and a logging configuration file was found in the deployments META-INF or WEB-INF/classes directory, then a log manager will be configured with those settings. If set false the servers logging configuration will be used regardless of any logging configuration files supplied in the deployment.
*/
@ModelNodeBinding(detypedName = "use-deployment-logging-config")
public Boolean useDeploymentLoggingConfig() {
return this.useDeploymentLoggingConfig;
}
/**
* Indicates whether or not deployments should use a logging configuration file found in the deployment to configure the log manager. If set to true and a logging configuration file was found in the deployments META-INF or WEB-INF/classes directory, then a log manager will be configured with those settings. If set false the servers logging configuration will be used regardless of any logging configuration files supplied in the deployment.
*/
@SuppressWarnings("unchecked")
public T useDeploymentLoggingConfig(Boolean value) {
this.useDeploymentLoggingConfig = value;
return (T) this;
}
public LoggingResources subresources() {
return this.subresources;
}
/**
* Add all org.wildfly.swarm.config.logging.PeriodicRotatingFileHandler objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.logging.PeriodicRotatingFileHandler objects.
*/
@SuppressWarnings("unchecked")
public T periodicRotatingFileHandlers(
List value) {
this.subresources.periodicRotatingFileHandlers.addAll(value);
return (T) this;
}
/**
* Add the org.wildfly.swarm.config.logging.PeriodicRotatingFileHandler object to the list of subresources
* @param value The org.wildfly.swarm.config.logging.PeriodicRotatingFileHandler to add
* @return this
*/
@SuppressWarnings("unchecked")
public T periodicRotatingFileHandler(PeriodicRotatingFileHandler value) {
this.subresources.periodicRotatingFileHandlers.add(value);
return (T) this;
}
/**
* Add all org.wildfly.swarm.config.logging.Logger objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.logging.Logger objects.
*/
@SuppressWarnings("unchecked")
public T loggers(List value) {
this.subresources.loggers.addAll(value);
return (T) this;
}
/**
* Add the org.wildfly.swarm.config.logging.Logger object to the list of subresources
* @param value The org.wildfly.swarm.config.logging.Logger to add
* @return this
*/
@SuppressWarnings("unchecked")
public T logger(Logger value) {
this.subresources.loggers.add(value);
return (T) this;
}
/**
* Add all org.wildfly.swarm.config.logging.AsyncHandler objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.logging.AsyncHandler objects.
*/
@SuppressWarnings("unchecked")
public T asyncHandlers(
List value) {
this.subresources.asyncHandlers.addAll(value);
return (T) this;
}
/**
* Add the org.wildfly.swarm.config.logging.AsyncHandler object to the list of subresources
* @param value The org.wildfly.swarm.config.logging.AsyncHandler to add
* @return this
*/
@SuppressWarnings("unchecked")
public T asyncHandler(AsyncHandler value) {
this.subresources.asyncHandlers.add(value);
return (T) this;
}
/**
* Add all org.wildfly.swarm.config.logging.FileHandler objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.logging.FileHandler objects.
*/
@SuppressWarnings("unchecked")
public T fileHandlers(
List value) {
this.subresources.fileHandlers.addAll(value);
return (T) this;
}
/**
* Add the org.wildfly.swarm.config.logging.FileHandler object to the list of subresources
* @param value The org.wildfly.swarm.config.logging.FileHandler to add
* @return this
*/
@SuppressWarnings("unchecked")
public T fileHandler(FileHandler value) {
this.subresources.fileHandlers.add(value);
return (T) this;
}
/**
* Add all org.wildfly.swarm.config.logging.SizeRotatingFileHandler objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.logging.SizeRotatingFileHandler objects.
*/
@SuppressWarnings("unchecked")
public T sizeRotatingFileHandlers(
List value) {
this.subresources.sizeRotatingFileHandlers.addAll(value);
return (T) this;
}
/**
* Add the org.wildfly.swarm.config.logging.SizeRotatingFileHandler object to the list of subresources
* @param value The org.wildfly.swarm.config.logging.SizeRotatingFileHandler to add
* @return this
*/
@SuppressWarnings("unchecked")
public T sizeRotatingFileHandler(SizeRotatingFileHandler value) {
this.subresources.sizeRotatingFileHandlers.add(value);
return (T) this;
}
/**
* Add all org.wildfly.swarm.config.logging.SyslogHandler objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.logging.SyslogHandler objects.
*/
@SuppressWarnings("unchecked")
public T syslogHandlers(
List value) {
this.subresources.syslogHandlers.addAll(value);
return (T) this;
}
/**
* Add the org.wildfly.swarm.config.logging.SyslogHandler object to the list of subresources
* @param value The org.wildfly.swarm.config.logging.SyslogHandler to add
* @return this
*/
@SuppressWarnings("unchecked")
public T syslogHandler(SyslogHandler value) {
this.subresources.syslogHandlers.add(value);
return (T) this;
}
/**
* Add all org.wildfly.swarm.config.logging.LoggingProfile objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.logging.LoggingProfile objects.
*/
@SuppressWarnings("unchecked")
public T loggingProfiles(
List value) {
this.subresources.loggingProfiles.addAll(value);
return (T) this;
}
/**
* Add the org.wildfly.swarm.config.logging.LoggingProfile object to the list of subresources
* @param value The org.wildfly.swarm.config.logging.LoggingProfile to add
* @return this
*/
@SuppressWarnings("unchecked")
public T loggingProfile(LoggingProfile value) {
this.subresources.loggingProfiles.add(value);
return (T) this;
}
/**
* Add all org.wildfly.swarm.config.logging.CustomFormatter objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.logging.CustomFormatter objects.
*/
@SuppressWarnings("unchecked")
public T customFormatters(
List value) {
this.subresources.customFormatters.addAll(value);
return (T) this;
}
/**
* Add the org.wildfly.swarm.config.logging.CustomFormatter object to the list of subresources
* @param value The org.wildfly.swarm.config.logging.CustomFormatter to add
* @return this
*/
@SuppressWarnings("unchecked")
public T customFormatter(CustomFormatter value) {
this.subresources.customFormatters.add(value);
return (T) this;
}
/**
* Add all org.wildfly.swarm.config.logging.PeriodicSizeRotatingFileHandler objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.logging.PeriodicSizeRotatingFileHandler objects.
*/
@SuppressWarnings("unchecked")
public T periodicSizeRotatingFileHandlers(
List value) {
this.subresources.periodicSizeRotatingFileHandlers.addAll(value);
return (T) this;
}
/**
* Add the org.wildfly.swarm.config.logging.PeriodicSizeRotatingFileHandler object to the list of subresources
* @param value The org.wildfly.swarm.config.logging.PeriodicSizeRotatingFileHandler to add
* @return this
*/
@SuppressWarnings("unchecked")
public T periodicSizeRotatingFileHandler(
PeriodicSizeRotatingFileHandler value) {
this.subresources.periodicSizeRotatingFileHandlers.add(value);
return (T) this;
}
/**
* Add all org.wildfly.swarm.config.logging.ConsoleHandler objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.logging.ConsoleHandler objects.
*/
@SuppressWarnings("unchecked")
public T consoleHandlers(
List value) {
this.subresources.consoleHandlers.addAll(value);
return (T) this;
}
/**
* Add the org.wildfly.swarm.config.logging.ConsoleHandler object to the list of subresources
* @param value The org.wildfly.swarm.config.logging.ConsoleHandler to add
* @return this
*/
@SuppressWarnings("unchecked")
public T consoleHandler(ConsoleHandler value) {
this.subresources.consoleHandlers.add(value);
return (T) this;
}
/**
* Add all org.wildfly.swarm.config.logging.LogFile objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.logging.LogFile objects.
*/
@SuppressWarnings("unchecked")
public T logFiles(List value) {
this.subresources.logFiles.addAll(value);
return (T) this;
}
/**
* Add the org.wildfly.swarm.config.logging.LogFile object to the list of subresources
* @param value The org.wildfly.swarm.config.logging.LogFile to add
* @return this
*/
@SuppressWarnings("unchecked")
public T logFile(LogFile value) {
this.subresources.logFiles.add(value);
return (T) this;
}
/**
* Add all org.wildfly.swarm.config.logging.PatternFormatter objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.logging.PatternFormatter objects.
*/
@SuppressWarnings("unchecked")
public T patternFormatters(
List value) {
this.subresources.patternFormatters.addAll(value);
return (T) this;
}
/**
* Add the org.wildfly.swarm.config.logging.PatternFormatter object to the list of subresources
* @param value The org.wildfly.swarm.config.logging.PatternFormatter to add
* @return this
*/
@SuppressWarnings("unchecked")
public T patternFormatter(PatternFormatter value) {
this.subresources.patternFormatters.add(value);
return (T) this;
}
/**
* Add all org.wildfly.swarm.config.logging.CustomHandler objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.logging.CustomHandler objects.
*/
@SuppressWarnings("unchecked")
public T customHandlers(
List value) {
this.subresources.customHandlers.addAll(value);
return (T) this;
}
/**
* Add the org.wildfly.swarm.config.logging.CustomHandler object to the list of subresources
* @param value The org.wildfly.swarm.config.logging.CustomHandler to add
* @return this
*/
@SuppressWarnings("unchecked")
public T customHandler(CustomHandler value) {
this.subresources.customHandlers.add(value);
return (T) this;
}
/**
* Child mutators for Logging
*/
public class LoggingResources {
/**
* Defines a handler which writes to a file, rotating the log after a time period derived from the given suffix string, which should be in a format understood by java.text.SimpleDateFormat.
*/
private List periodicRotatingFileHandlers = new java.util.ArrayList<>();
/**
* Defines a logger category.
*/
private List loggers = new java.util.ArrayList<>();
/**
* Defines a handler which writes to the sub-handlers in an asynchronous thread. Used for handlers which introduce a substantial amount of lag.
*/
private List asyncHandlers = new java.util.ArrayList<>();
/**
* Defines a handler which writes to a file.
*/
private List fileHandlers = new java.util.ArrayList<>();
/**
* Defines a handler which writes to a file, rotating the log after the size of the file grows beyond a certain point and keeping a fixed number of backups.
*/
private List sizeRotatingFileHandlers = new java.util.ArrayList<>();
/**
* Defines a syslog handler.
*/
private List syslogHandlers = new java.util.ArrayList<>();
/**
* The configuration of the logging subsystem.
*/
private List loggingProfiles = new java.util.ArrayList<>();
/**
* A custom formatter to be used with handlers. Note that most log records are formatted in the printf format. Formatters may require invocation of the org.jboss.logmanager.ExtLogRecord#getFormattedMessage() for the message to be properly formatted.
*/
private List customFormatters = new java.util.ArrayList<>();
/**
* Defines a handler which writes to a file, rotating the log after a time period derived from the given suffix string or after the size of the file grows beyond a certain point and keeping a fixed number of backups. The suffix should be in a format understood by the java.text.SimpleDateFormat. Any backups rotated by the suffix will not be purged during a size rotation.
*/
private List periodicSizeRotatingFileHandlers = new java.util.ArrayList<>();
/**
* Defines a handler which writes to the console.
*/
private List consoleHandlers = new java.util.ArrayList<>();
/**
* Log files that are available to be read.
*/
private List logFiles = new java.util.ArrayList<>();
/**
* A pattern formatter to be used with handlers.
*/
private List patternFormatters = new java.util.ArrayList<>();
/**
* Defines a custom logging handler. The custom handler must extend java.util.logging.Handler.
*/
private List customHandlers = new java.util.ArrayList<>();
/**
* Get the list of org.wildfly.swarm.config.logging.PeriodicRotatingFileHandler resources
* @return the list of resources
*/
@Subresource
public List periodicRotatingFileHandlers() {
return this.periodicRotatingFileHandlers;
}
/**
* Get the list of org.wildfly.swarm.config.logging.Logger resources
* @return the list of resources
*/
@Subresource
public List loggers() {
return this.loggers;
}
/**
* Get the list of org.wildfly.swarm.config.logging.AsyncHandler resources
* @return the list of resources
*/
@Subresource
public List asyncHandlers() {
return this.asyncHandlers;
}
/**
* Get the list of org.wildfly.swarm.config.logging.FileHandler resources
* @return the list of resources
*/
@Subresource
public List fileHandlers() {
return this.fileHandlers;
}
/**
* Get the list of org.wildfly.swarm.config.logging.SizeRotatingFileHandler resources
* @return the list of resources
*/
@Subresource
public List sizeRotatingFileHandlers() {
return this.sizeRotatingFileHandlers;
}
/**
* Get the list of org.wildfly.swarm.config.logging.SyslogHandler resources
* @return the list of resources
*/
@Subresource
public List syslogHandlers() {
return this.syslogHandlers;
}
/**
* Get the list of org.wildfly.swarm.config.logging.LoggingProfile resources
* @return the list of resources
*/
@Subresource
public List loggingProfiles() {
return this.loggingProfiles;
}
/**
* Get the list of org.wildfly.swarm.config.logging.CustomFormatter resources
* @return the list of resources
*/
@Subresource
public List customFormatters() {
return this.customFormatters;
}
/**
* Get the list of org.wildfly.swarm.config.logging.PeriodicSizeRotatingFileHandler resources
* @return the list of resources
*/
@Subresource
public List periodicSizeRotatingFileHandlers() {
return this.periodicSizeRotatingFileHandlers;
}
/**
* Get the list of org.wildfly.swarm.config.logging.ConsoleHandler resources
* @return the list of resources
*/
@Subresource
public List consoleHandlers() {
return this.consoleHandlers;
}
/**
* Get the list of org.wildfly.swarm.config.logging.LogFile resources
* @return the list of resources
*/
@Subresource
public List logFiles() {
return this.logFiles;
}
/**
* Get the list of org.wildfly.swarm.config.logging.PatternFormatter resources
* @return the list of resources
*/
@Subresource
public List patternFormatters() {
return this.patternFormatters;
}
/**
* Get the list of org.wildfly.swarm.config.logging.CustomHandler resources
* @return the list of resources
*/
@Subresource
public List customHandlers() {
return this.customHandlers;
}
}
/**
* Defines the root logger for this log context.
*/
@Subresource
public RootLogger rootLogger() {
return this.rootLogger;
}
/**
* Defines the root logger for this log context.
*/
@SuppressWarnings("unchecked")
public T rootLogger(RootLogger value) {
this.rootLogger = value;
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy