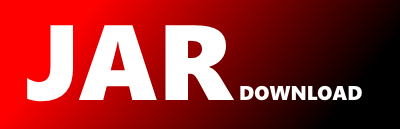
org.wildfly.swarm.config.Remoting Maven / Gradle / Ivy
package org.wildfly.swarm.config;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.ResourceType;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.List;
import org.wildfly.config.runtime.Subresource;
import org.wildfly.swarm.config.remoting.HttpConnector;
import org.wildfly.swarm.config.remoting.Connector;
import org.wildfly.swarm.config.remoting.OutboundConnection;
import org.wildfly.swarm.config.remoting.LocalOutboundConnection;
import org.wildfly.swarm.config.remoting.RemoteOutboundConnection;
import org.wildfly.swarm.config.remoting.EndpointConfiguration;
/**
* The configuration of the Remoting subsystem.
*/
@ResourceType("subsystem")
@Implicit
public class Remoting {
private String key;
private RemotingResources subresources = new RemotingResources();
private EndpointConfiguration endpointConfiguration;
public Remoting() {
this.key = "remoting";
}
public String getKey() {
return this.key;
}
public RemotingResources subresources() {
return this.subresources;
}
/**
* Add all org.wildfly.swarm.config.remoting.HttpConnector objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.remoting.HttpConnector objects.
*/
@SuppressWarnings("unchecked")
public T httpConnectors(
List value) {
this.subresources.httpConnectors.addAll(value);
return (T) this;
}
/**
* Add the org.wildfly.swarm.config.remoting.HttpConnector object to the list of subresources
* @param value The org.wildfly.swarm.config.remoting.HttpConnector to add
* @return this
*/
@SuppressWarnings("unchecked")
public T httpConnector(HttpConnector value) {
this.subresources.httpConnectors.add(value);
return (T) this;
}
/**
* Add all org.wildfly.swarm.config.remoting.Connector objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.remoting.Connector objects.
*/
@SuppressWarnings("unchecked")
public T connectors(List value) {
this.subresources.connectors.addAll(value);
return (T) this;
}
/**
* Add the org.wildfly.swarm.config.remoting.Connector object to the list of subresources
* @param value The org.wildfly.swarm.config.remoting.Connector to add
* @return this
*/
@SuppressWarnings("unchecked")
public T connector(Connector value) {
this.subresources.connectors.add(value);
return (T) this;
}
/**
* Add all org.wildfly.swarm.config.remoting.OutboundConnection objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.remoting.OutboundConnection objects.
*/
@SuppressWarnings("unchecked")
public T outboundConnections(
List value) {
this.subresources.outboundConnections.addAll(value);
return (T) this;
}
/**
* Add the org.wildfly.swarm.config.remoting.OutboundConnection object to the list of subresources
* @param value The org.wildfly.swarm.config.remoting.OutboundConnection to add
* @return this
*/
@SuppressWarnings("unchecked")
public T outboundConnection(OutboundConnection value) {
this.subresources.outboundConnections.add(value);
return (T) this;
}
/**
* Add all org.wildfly.swarm.config.remoting.LocalOutboundConnection objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.remoting.LocalOutboundConnection objects.
*/
@SuppressWarnings("unchecked")
public T localOutboundConnections(
List value) {
this.subresources.localOutboundConnections.addAll(value);
return (T) this;
}
/**
* Add the org.wildfly.swarm.config.remoting.LocalOutboundConnection object to the list of subresources
* @param value The org.wildfly.swarm.config.remoting.LocalOutboundConnection to add
* @return this
*/
@SuppressWarnings("unchecked")
public T localOutboundConnection(LocalOutboundConnection value) {
this.subresources.localOutboundConnections.add(value);
return (T) this;
}
/**
* Add all org.wildfly.swarm.config.remoting.RemoteOutboundConnection objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.remoting.RemoteOutboundConnection objects.
*/
@SuppressWarnings("unchecked")
public T remoteOutboundConnections(
List value) {
this.subresources.remoteOutboundConnections.addAll(value);
return (T) this;
}
/**
* Add the org.wildfly.swarm.config.remoting.RemoteOutboundConnection object to the list of subresources
* @param value The org.wildfly.swarm.config.remoting.RemoteOutboundConnection to add
* @return this
*/
@SuppressWarnings("unchecked")
public T remoteOutboundConnection(RemoteOutboundConnection value) {
this.subresources.remoteOutboundConnections.add(value);
return (T) this;
}
/**
* Child mutators for Remoting
*/
public class RemotingResources {
/**
* The configuration of a HTTP Upgrade based Remoting connector.
*/
private List httpConnectors = new java.util.ArrayList<>();
/**
* The configuration of a Remoting connector.
*/
private List connectors = new java.util.ArrayList<>();
/**
* Remoting outbound connection.
*/
private List outboundConnections = new java.util.ArrayList<>();
/**
* Remoting outbound connection with an implicit local:// URI scheme.
*/
private List localOutboundConnections = new java.util.ArrayList<>();
/**
* Remoting outbound connection with an implicit remote:// URI scheme.
*/
private List remoteOutboundConnections = new java.util.ArrayList<>();
/**
* Get the list of org.wildfly.swarm.config.remoting.HttpConnector resources
* @return the list of resources
*/
@Subresource
public List httpConnectors() {
return this.httpConnectors;
}
/**
* Get the list of org.wildfly.swarm.config.remoting.Connector resources
* @return the list of resources
*/
@Subresource
public List connectors() {
return this.connectors;
}
/**
* Get the list of org.wildfly.swarm.config.remoting.OutboundConnection resources
* @return the list of resources
*/
@Subresource
public List outboundConnections() {
return this.outboundConnections;
}
/**
* Get the list of org.wildfly.swarm.config.remoting.LocalOutboundConnection resources
* @return the list of resources
*/
@Subresource
public List localOutboundConnections() {
return this.localOutboundConnections;
}
/**
* Get the list of org.wildfly.swarm.config.remoting.RemoteOutboundConnection resources
* @return the list of resources
*/
@Subresource
public List remoteOutboundConnections() {
return this.remoteOutboundConnections;
}
}
/**
* Endpoint configuration
*/
@Subresource
public EndpointConfiguration endpointConfiguration() {
return this.endpointConfiguration;
}
/**
* Endpoint configuration
*/
@SuppressWarnings("unchecked")
public T endpointConfiguration(EndpointConfiguration value) {
this.endpointConfiguration = value;
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy