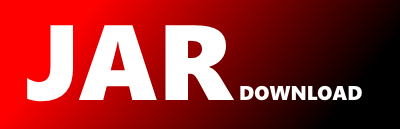
org.wildfly.swarm.config.datasources.JdbcDriver Maven / Gradle / Ivy
package org.wildfly.swarm.config.datasources;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.ResourceType;
import org.wildfly.config.runtime.ModelNodeBinding;
/**
* Service that make a JDBC driver available for use in the runtime
*/
@ResourceType("jdbc-driver")
public class JdbcDriver {
private String key;
private String deploymentName;
private String driverClassName;
private String driverDatasourceClassName;
private Integer driverMajorVersion;
private Integer driverMinorVersion;
private String driverModuleName;
private String driverName;
private String driverXaDatasourceClassName;
private Boolean jdbcCompliant;
private String moduleSlot;
private String profile;
private String xaDatasourceClass;
public JdbcDriver(String key) {
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* The name of the deployment unit from which the driver was loaded
*/
@ModelNodeBinding(detypedName = "deployment-name")
public String deploymentName() {
return this.deploymentName;
}
/**
* The name of the deployment unit from which the driver was loaded
*/
@SuppressWarnings("unchecked")
public JdbcDriver deploymentName(String value) {
this.deploymentName = value;
return (JdbcDriver) this;
}
/**
* The fully qualified class name of the java.sql.Driver implementation
*/
@ModelNodeBinding(detypedName = "driver-class-name")
public String driverClassName() {
return this.driverClassName;
}
/**
* The fully qualified class name of the java.sql.Driver implementation
*/
@SuppressWarnings("unchecked")
public JdbcDriver driverClassName(String value) {
this.driverClassName = value;
return (JdbcDriver) this;
}
/**
* The fully qualified class name of the javax.sql.DataSource implementation
*/
@ModelNodeBinding(detypedName = "driver-datasource-class-name")
public String driverDatasourceClassName() {
return this.driverDatasourceClassName;
}
/**
* The fully qualified class name of the javax.sql.DataSource implementation
*/
@SuppressWarnings("unchecked")
public JdbcDriver driverDatasourceClassName(String value) {
this.driverDatasourceClassName = value;
return (JdbcDriver) this;
}
/**
* The driver's major version number
*/
@ModelNodeBinding(detypedName = "driver-major-version")
public Integer driverMajorVersion() {
return this.driverMajorVersion;
}
/**
* The driver's major version number
*/
@SuppressWarnings("unchecked")
public JdbcDriver driverMajorVersion(Integer value) {
this.driverMajorVersion = value;
return (JdbcDriver) this;
}
/**
* The driver's minor version number
*/
@ModelNodeBinding(detypedName = "driver-minor-version")
public Integer driverMinorVersion() {
return this.driverMinorVersion;
}
/**
* The driver's minor version number
*/
@SuppressWarnings("unchecked")
public JdbcDriver driverMinorVersion(Integer value) {
this.driverMinorVersion = value;
return (JdbcDriver) this;
}
/**
* The name of the module from which the driver was loaded, if it was loaded from the module path
*/
@ModelNodeBinding(detypedName = "driver-module-name")
public String driverModuleName() {
return this.driverModuleName;
}
/**
* The name of the module from which the driver was loaded, if it was loaded from the module path
*/
@SuppressWarnings("unchecked")
public JdbcDriver driverModuleName(String value) {
this.driverModuleName = value;
return (JdbcDriver) this;
}
/**
* Defines the JDBC driver the datasource should use. It is a symbolic name matching the the name of installed driver. In case the driver is deployed as jar, the name is the name of deployment unit
*/
@ModelNodeBinding(detypedName = "driver-name")
public String driverName() {
return this.driverName;
}
/**
* Defines the JDBC driver the datasource should use. It is a symbolic name matching the the name of installed driver. In case the driver is deployed as jar, the name is the name of deployment unit
*/
@SuppressWarnings("unchecked")
public JdbcDriver driverName(String value) {
this.driverName = value;
return (JdbcDriver) this;
}
/**
* The fully qualified class name of the javax.sql.XADataSource implementation
*/
@ModelNodeBinding(detypedName = "driver-xa-datasource-class-name")
public String driverXaDatasourceClassName() {
return this.driverXaDatasourceClassName;
}
/**
* The fully qualified class name of the javax.sql.XADataSource implementation
*/
@SuppressWarnings("unchecked")
public JdbcDriver driverXaDatasourceClassName(String value) {
this.driverXaDatasourceClassName = value;
return (JdbcDriver) this;
}
/**
* Whether or not the driver is JDBC compliant
*/
@ModelNodeBinding(detypedName = "jdbc-compliant")
public Boolean jdbcCompliant() {
return this.jdbcCompliant;
}
/**
* Whether or not the driver is JDBC compliant
*/
@SuppressWarnings("unchecked")
public JdbcDriver jdbcCompliant(Boolean value) {
this.jdbcCompliant = value;
return (JdbcDriver) this;
}
/**
* The slot of the module from which the driver was loaded, if it was loaded from the module path
*/
@ModelNodeBinding(detypedName = "module-slot")
public String moduleSlot() {
return this.moduleSlot;
}
/**
* The slot of the module from which the driver was loaded, if it was loaded from the module path
*/
@SuppressWarnings("unchecked")
public JdbcDriver moduleSlot(String value) {
this.moduleSlot = value;
return (JdbcDriver) this;
}
/**
* Domain Profile in which driver is defined. Null in case of standalone server
*/
@ModelNodeBinding(detypedName = "profile")
public String profile() {
return this.profile;
}
/**
* Domain Profile in which driver is defined. Null in case of standalone server
*/
@SuppressWarnings("unchecked")
public JdbcDriver profile(String value) {
this.profile = value;
return (JdbcDriver) this;
}
/**
* XA datasource class
*/
@ModelNodeBinding(detypedName = "xa-datasource-class")
public String xaDatasourceClass() {
return this.xaDatasourceClass;
}
/**
* XA datasource class
*/
@SuppressWarnings("unchecked")
public JdbcDriver xaDatasourceClass(String value) {
this.xaDatasourceClass = value;
return (JdbcDriver) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy