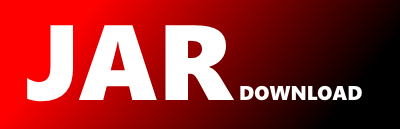
org.wildfly.swarm.config.ee.ManagedScheduledExecutorService Maven / Gradle / Ivy
package org.wildfly.swarm.config.ee;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.ResourceType;
import org.wildfly.config.runtime.ModelNodeBinding;
/**
* A managed scheduled executor service
*/
@ResourceType("managed-scheduled-executor-service")
public class ManagedScheduledExecutorService {
private String key;
private String contextService;
private Integer coreThreads;
private Long hungTaskThreshold;
private String jndiName;
private Long keepaliveTime;
private Boolean longRunningTasks;
private String rejectPolicy;
private String threadFactory;
public ManagedScheduledExecutorService(String key) {
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* The name of the context service to be used by the scheduled executor.
*/
@ModelNodeBinding(detypedName = "context-service")
public String contextService() {
return this.contextService;
}
/**
* The name of the context service to be used by the scheduled executor.
*/
@SuppressWarnings("unchecked")
public ManagedScheduledExecutorService contextService(String value) {
this.contextService = value;
return (ManagedScheduledExecutorService) this;
}
/**
* The minimum number of threads to be used by the scheduled executor.
*/
@ModelNodeBinding(detypedName = "core-threads")
public Integer coreThreads() {
return this.coreThreads;
}
/**
* The minimum number of threads to be used by the scheduled executor.
*/
@SuppressWarnings("unchecked")
public ManagedScheduledExecutorService coreThreads(Integer value) {
this.coreThreads = value;
return (ManagedScheduledExecutorService) this;
}
/**
* The runtime, in milliseconds, for tasks to be considered hung by the scheduled executor. If 0 tasks are never considered hung.
*/
@ModelNodeBinding(detypedName = "hung-task-threshold")
public Long hungTaskThreshold() {
return this.hungTaskThreshold;
}
/**
* The runtime, in milliseconds, for tasks to be considered hung by the scheduled executor. If 0 tasks are never considered hung.
*/
@SuppressWarnings("unchecked")
public ManagedScheduledExecutorService hungTaskThreshold(Long value) {
this.hungTaskThreshold = value;
return (ManagedScheduledExecutorService) this;
}
/**
* The JNDI Name to lookup the managed scheduled executor service.
*/
@ModelNodeBinding(detypedName = "jndi-name")
public String jndiName() {
return this.jndiName;
}
/**
* The JNDI Name to lookup the managed scheduled executor service.
*/
@SuppressWarnings("unchecked")
public ManagedScheduledExecutorService jndiName(String value) {
this.jndiName = value;
return (ManagedScheduledExecutorService) this;
}
/**
* When the number of threads is greater than the core, this is the maximum time, in milliseconds, that excess idle threads will wait for new tasks before terminating.
*/
@ModelNodeBinding(detypedName = "keepalive-time")
public Long keepaliveTime() {
return this.keepaliveTime;
}
/**
* When the number of threads is greater than the core, this is the maximum time, in milliseconds, that excess idle threads will wait for new tasks before terminating.
*/
@SuppressWarnings("unchecked")
public ManagedScheduledExecutorService keepaliveTime(Long value) {
this.keepaliveTime = value;
return (ManagedScheduledExecutorService) this;
}
/**
* Flag which hints the duration of tasks executed by the scheduled executor.
*/
@ModelNodeBinding(detypedName = "long-running-tasks")
public Boolean longRunningTasks() {
return this.longRunningTasks;
}
/**
* Flag which hints the duration of tasks executed by the scheduled executor.
*/
@SuppressWarnings("unchecked")
public ManagedScheduledExecutorService longRunningTasks(Boolean value) {
this.longRunningTasks = value;
return (ManagedScheduledExecutorService) this;
}
/**
* The policy to be applied to aborted tasks.
*/
@ModelNodeBinding(detypedName = "reject-policy")
public String rejectPolicy() {
return this.rejectPolicy;
}
/**
* The policy to be applied to aborted tasks.
*/
@SuppressWarnings("unchecked")
public ManagedScheduledExecutorService rejectPolicy(String value) {
this.rejectPolicy = value;
return (ManagedScheduledExecutorService) this;
}
/**
* The name of the thread factory to be used by the scheduled executor.
*/
@ModelNodeBinding(detypedName = "thread-factory")
public String threadFactory() {
return this.threadFactory;
}
/**
* The name of the thread factory to be used by the scheduled executor.
*/
@SuppressWarnings("unchecked")
public ManagedScheduledExecutorService threadFactory(String value) {
this.threadFactory = value;
return (ManagedScheduledExecutorService) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy