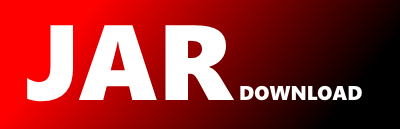
org.wildfly.swarm.config.ejb3.RemoteService Maven / Gradle / Ivy
package org.wildfly.swarm.config.ejb3;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.ResourceType;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.List;
import org.wildfly.config.runtime.Subresource;
/**
* The EJB3 Remote Service
*/
@ResourceType("service")
@Implicit
public class RemoteService {
private String key;
private String cluster;
private String connectorRef;
private String threadPoolName;
private RemoteServiceResources subresources = new RemoteServiceResources();
public RemoteService() {
this.key = "remote";
}
public String getKey() {
return this.key;
}
/**
* The name of the clustered cache container which will be used to store/access the client-mappings of the EJB remoting connector's socket-binding on each node, in the cluster
*/
@ModelNodeBinding(detypedName = "cluster")
public String cluster() {
return this.cluster;
}
/**
* The name of the clustered cache container which will be used to store/access the client-mappings of the EJB remoting connector's socket-binding on each node, in the cluster
*/
@SuppressWarnings("unchecked")
public RemoteService cluster(String value) {
this.cluster = value;
return (RemoteService) this;
}
/**
* The name of the connector on which the EJB3 remoting channel is registered
*/
@ModelNodeBinding(detypedName = "connector-ref")
public String connectorRef() {
return this.connectorRef;
}
/**
* The name of the connector on which the EJB3 remoting channel is registered
*/
@SuppressWarnings("unchecked")
public RemoteService connectorRef(String value) {
this.connectorRef = value;
return (RemoteService) this;
}
/**
* The name of the thread pool that handles remote invocations
*/
@ModelNodeBinding(detypedName = "thread-pool-name")
public String threadPoolName() {
return this.threadPoolName;
}
/**
* The name of the thread pool that handles remote invocations
*/
@SuppressWarnings("unchecked")
public RemoteService threadPoolName(String value) {
this.threadPoolName = value;
return (RemoteService) this;
}
public RemoteServiceResources subresources() {
return this.subresources;
}
/**
* Add all org.wildfly.swarm.config.ejb3.ChannelCreationOptions objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.ejb3.ChannelCreationOptions objects.
*/
@SuppressWarnings("unchecked")
public RemoteService channelCreationOptions(
List value) {
this.subresources.channelCreationOptions.addAll(value);
return (RemoteService) this;
}
/**
* Add the org.wildfly.swarm.config.ejb3.ChannelCreationOptions object to the list of subresources
* @param value The org.wildfly.swarm.config.ejb3.ChannelCreationOptions to add
* @return this
*/
@SuppressWarnings("unchecked")
public RemoteService channelCreationOptions(ChannelCreationOptions value) {
this.subresources.channelCreationOptions.add(value);
return (RemoteService) this;
}
/**
* Child mutators for RemoteService
*/
public class RemoteServiceResources {
/**
* The options that will be used during the EJB remote channel creation
*/
private List channelCreationOptions = new java.util.ArrayList<>();
/**
* Get the list of org.wildfly.swarm.config.ejb3.ChannelCreationOptions resources
* @return the list of resources
*/
@Subresource
public List channelCreationOptions() {
return this.channelCreationOptions;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy