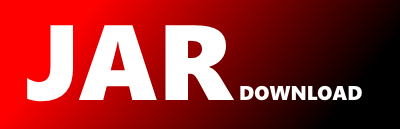
org.wildfly.swarm.config.ejb3.StrictMaxBeanInstancePool Maven / Gradle / Ivy
package org.wildfly.swarm.config.ejb3;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.ResourceType;
import org.wildfly.config.runtime.ModelNodeBinding;
/**
* A bean instance pool with a strict upper limit
*/
@ResourceType("strict-max-bean-instance-pool")
public class StrictMaxBeanInstancePool {
private String key;
private String deriveSize;
private Integer maxPoolSize;
private Long timeout;
private String timeoutUnit;
public StrictMaxBeanInstancePool(String key) {
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* Specifies if and what the max pool size should be derived from. A value of 'none', the default, indicates that the explicit value of max-pool-size should be used. A value of 'from-worker-pools' indicates that the max pool size should be derived from the size of the total threads for all worker pools configured on the system. A value of 'from-cpu-count' indicates that the max pool size should be derived from the total number of processors available on the system. Note that the computation isn't a 1:1 mapping, the values may or may not be augmented by other factors.
*/
@ModelNodeBinding(detypedName = "derive-size")
public String deriveSize() {
return this.deriveSize;
}
/**
* Specifies if and what the max pool size should be derived from. A value of 'none', the default, indicates that the explicit value of max-pool-size should be used. A value of 'from-worker-pools' indicates that the max pool size should be derived from the size of the total threads for all worker pools configured on the system. A value of 'from-cpu-count' indicates that the max pool size should be derived from the total number of processors available on the system. Note that the computation isn't a 1:1 mapping, the values may or may not be augmented by other factors.
*/
@SuppressWarnings("unchecked")
public StrictMaxBeanInstancePool deriveSize(String value) {
this.deriveSize = value;
return (StrictMaxBeanInstancePool) this;
}
/**
* The maximum number of bean instances that the pool can hold at a given point in time
*/
@ModelNodeBinding(detypedName = "max-pool-size")
public Integer maxPoolSize() {
return this.maxPoolSize;
}
/**
* The maximum number of bean instances that the pool can hold at a given point in time
*/
@SuppressWarnings("unchecked")
public StrictMaxBeanInstancePool maxPoolSize(Integer value) {
this.maxPoolSize = value;
return (StrictMaxBeanInstancePool) this;
}
/**
* The maximum amount of time to wait for a bean instance to be available from the pool
*/
@ModelNodeBinding(detypedName = "timeout")
public Long timeout() {
return this.timeout;
}
/**
* The maximum amount of time to wait for a bean instance to be available from the pool
*/
@SuppressWarnings("unchecked")
public StrictMaxBeanInstancePool timeout(Long value) {
this.timeout = value;
return (StrictMaxBeanInstancePool) this;
}
/**
* The instance acquisition timeout unit
*/
@ModelNodeBinding(detypedName = "timeout-unit")
public String timeoutUnit() {
return this.timeoutUnit;
}
/**
* The instance acquisition timeout unit
*/
@SuppressWarnings("unchecked")
public StrictMaxBeanInstancePool timeoutUnit(String value) {
this.timeoutUnit = value;
return (StrictMaxBeanInstancePool) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy