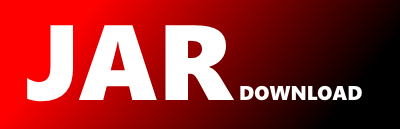
org.wildfly.swarm.config.ejb3.ThreadPool Maven / Gradle / Ivy
package org.wildfly.swarm.config.ejb3;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.ResourceType;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.Map;
/**
* A thread pool executor with an unbounded queue. Such a thread pool has a core size and a queue with no upper bound. When a task is submitted, if the number of running threads is less than the core size, a new thread is created. Otherwise, the task is placed in queue. If too many tasks are allowed to be submitted to this type of executor, an out of memory condition may occur.
*/
@ResourceType("thread-pool")
public class ThreadPool {
private String key;
private Integer activeCount;
private Integer completedTaskCount;
private Integer currentThreadCount;
private Map keepaliveTime;
private Integer largestThreadCount;
private Integer maxThreads;
private String name;
private Integer queueSize;
private Integer rejectedCount;
private Integer taskCount;
private String threadFactory;
public ThreadPool(String key) {
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* The approximate number of threads that are actively executing tasks.
*/
@ModelNodeBinding(detypedName = "active-count")
public Integer activeCount() {
return this.activeCount;
}
/**
* The approximate number of threads that are actively executing tasks.
*/
@SuppressWarnings("unchecked")
public ThreadPool activeCount(Integer value) {
this.activeCount = value;
return (ThreadPool) this;
}
/**
* The approximate total number of tasks that have completed execution.
*/
@ModelNodeBinding(detypedName = "completed-task-count")
public Integer completedTaskCount() {
return this.completedTaskCount;
}
/**
* The approximate total number of tasks that have completed execution.
*/
@SuppressWarnings("unchecked")
public ThreadPool completedTaskCount(Integer value) {
this.completedTaskCount = value;
return (ThreadPool) this;
}
/**
* The current number of threads in the pool.
*/
@ModelNodeBinding(detypedName = "current-thread-count")
public Integer currentThreadCount() {
return this.currentThreadCount;
}
/**
* The current number of threads in the pool.
*/
@SuppressWarnings("unchecked")
public ThreadPool currentThreadCount(Integer value) {
this.currentThreadCount = value;
return (ThreadPool) this;
}
/**
* Used to specify the amount of time that pool threads should be kept running when idle; if not specified, threads will run until the executor is shut down.
*/
@ModelNodeBinding(detypedName = "keepalive-time")
public Map keepaliveTime() {
return this.keepaliveTime;
}
/**
* Used to specify the amount of time that pool threads should be kept running when idle; if not specified, threads will run until the executor is shut down.
*/
@SuppressWarnings("unchecked")
public ThreadPool keepaliveTime(Map value) {
this.keepaliveTime = value;
return (ThreadPool) this;
}
/**
* The largest number of threads that have ever simultaneously been in the pool.
*/
@ModelNodeBinding(detypedName = "largest-thread-count")
public Integer largestThreadCount() {
return this.largestThreadCount;
}
/**
* The largest number of threads that have ever simultaneously been in the pool.
*/
@SuppressWarnings("unchecked")
public ThreadPool largestThreadCount(Integer value) {
this.largestThreadCount = value;
return (ThreadPool) this;
}
/**
* The maximum thread pool size.
*/
@ModelNodeBinding(detypedName = "max-threads")
public Integer maxThreads() {
return this.maxThreads;
}
/**
* The maximum thread pool size.
*/
@SuppressWarnings("unchecked")
public ThreadPool maxThreads(Integer value) {
this.maxThreads = value;
return (ThreadPool) this;
}
/**
* The name of the thread pool.
*/
@ModelNodeBinding(detypedName = "name")
public String name() {
return this.name;
}
/**
* The name of the thread pool.
*/
@SuppressWarnings("unchecked")
public ThreadPool name(String value) {
this.name = value;
return (ThreadPool) this;
}
/**
* The queue size.
*/
@ModelNodeBinding(detypedName = "queue-size")
public Integer queueSize() {
return this.queueSize;
}
/**
* The queue size.
*/
@SuppressWarnings("unchecked")
public ThreadPool queueSize(Integer value) {
this.queueSize = value;
return (ThreadPool) this;
}
/**
* The number of tasks that have been rejected.
*/
@ModelNodeBinding(detypedName = "rejected-count")
public Integer rejectedCount() {
return this.rejectedCount;
}
/**
* The number of tasks that have been rejected.
*/
@SuppressWarnings("unchecked")
public ThreadPool rejectedCount(Integer value) {
this.rejectedCount = value;
return (ThreadPool) this;
}
/**
* The approximate total number of tasks that have ever been scheduled for execution.
*/
@ModelNodeBinding(detypedName = "task-count")
public Integer taskCount() {
return this.taskCount;
}
/**
* The approximate total number of tasks that have ever been scheduled for execution.
*/
@SuppressWarnings("unchecked")
public ThreadPool taskCount(Integer value) {
this.taskCount = value;
return (ThreadPool) this;
}
/**
* Specifies the name of a specific thread factory to use to create worker threads. If not defined an appropriate default thread factory will be used.
*/
@ModelNodeBinding(detypedName = "thread-factory")
public String threadFactory() {
return this.threadFactory;
}
/**
* Specifies the name of a specific thread factory to use to create worker threads. If not defined an appropriate default thread factory will be used.
*/
@SuppressWarnings("unchecked")
public ThreadPool threadFactory(String value) {
this.threadFactory = value;
return (ThreadPool) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy