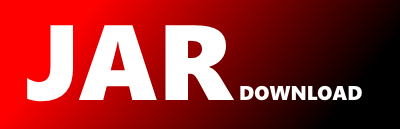
org.wildfly.swarm.config.ejb3.TimerService Maven / Gradle / Ivy
package org.wildfly.swarm.config.ejb3;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.ResourceType;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.List;
import org.wildfly.config.runtime.Subresource;
import org.wildfly.swarm.config.ejb3.service.FileDataStore;
import org.wildfly.swarm.config.ejb3.service.DatabaseDataStore;
/**
* The EJB timer service
*/
@ResourceType("service")
@Implicit
public class TimerService {
private String key;
private String defaultDataStore;
private String threadPoolName;
private TimerServiceResources subresources = new TimerServiceResources();
public TimerService() {
this.key = "timer-service";
}
public String getKey() {
return this.key;
}
/**
* The default data store used for persistent timers
*/
@ModelNodeBinding(detypedName = "default-data-store")
public String defaultDataStore() {
return this.defaultDataStore;
}
/**
* The default data store used for persistent timers
*/
@SuppressWarnings("unchecked")
public TimerService defaultDataStore(String value) {
this.defaultDataStore = value;
return (TimerService) this;
}
/**
* The name of the thread pool used to run timer service invocations
*/
@ModelNodeBinding(detypedName = "thread-pool-name")
public String threadPoolName() {
return this.threadPoolName;
}
/**
* The name of the thread pool used to run timer service invocations
*/
@SuppressWarnings("unchecked")
public TimerService threadPoolName(String value) {
this.threadPoolName = value;
return (TimerService) this;
}
public TimerServiceResources subresources() {
return this.subresources;
}
/**
* Add all org.wildfly.swarm.config.ejb3.service.FileDataStore objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.ejb3.service.FileDataStore objects.
*/
@SuppressWarnings("unchecked")
public TimerService fileDataStores(
List value) {
this.subresources.fileDataStores.addAll(value);
return (TimerService) this;
}
/**
* Add the org.wildfly.swarm.config.ejb3.service.FileDataStore object to the list of subresources
* @param value The org.wildfly.swarm.config.ejb3.service.FileDataStore to add
* @return this
*/
@SuppressWarnings("unchecked")
public TimerService fileDataStore(FileDataStore value) {
this.subresources.fileDataStores.add(value);
return (TimerService) this;
}
/**
* Add all org.wildfly.swarm.config.ejb3.service.DatabaseDataStore objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.ejb3.service.DatabaseDataStore objects.
*/
@SuppressWarnings("unchecked")
public TimerService databaseDataStores(
List value) {
this.subresources.databaseDataStores.addAll(value);
return (TimerService) this;
}
/**
* Add the org.wildfly.swarm.config.ejb3.service.DatabaseDataStore object to the list of subresources
* @param value The org.wildfly.swarm.config.ejb3.service.DatabaseDataStore to add
* @return this
*/
@SuppressWarnings("unchecked")
public TimerService databaseDataStore(DatabaseDataStore value) {
this.subresources.databaseDataStores.add(value);
return (TimerService) this;
}
/**
* Child mutators for TimerService
*/
public class TimerServiceResources {
/**
* A JVM local file store that stores persistent EJB timers
*/
private List fileDataStores = new java.util.ArrayList<>();
/**
* An database based store for persistent EJB timers.
*/
private List databaseDataStores = new java.util.ArrayList<>();
/**
* Get the list of org.wildfly.swarm.config.ejb3.service.FileDataStore resources
* @return the list of resources
*/
@Subresource
public List fileDataStores() {
return this.fileDataStores;
}
/**
* Get the list of org.wildfly.swarm.config.ejb3.service.DatabaseDataStore resources
* @return the list of resources
*/
@Subresource
public List databaseDataStores() {
return this.databaseDataStores;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy