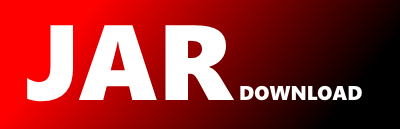
org.wildfly.swarm.config.infinispan.CacheContainer Maven / Gradle / Ivy
package org.wildfly.swarm.config.infinispan;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.ResourceType;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.List;
import org.wildfly.config.runtime.Subresource;
import org.wildfly.swarm.config.infinispan.cache_container.InvalidationCache;
import org.wildfly.swarm.config.infinispan.cache_container.LocalCache;
import org.wildfly.swarm.config.infinispan.cache_container.ReplicatedCache;
import org.wildfly.swarm.config.infinispan.cache_container.DistributedCache;
import org.wildfly.swarm.config.infinispan.cache_container.JgroupsTransport;
import org.wildfly.swarm.config.infinispan.cache_container.RemoteCommandThreadPool;
import org.wildfly.swarm.config.infinispan.cache_container.AsyncOperationsThreadPool;
import org.wildfly.swarm.config.infinispan.cache_container.ExpirationThreadPool;
import org.wildfly.swarm.config.infinispan.cache_container.NoneTransport;
import org.wildfly.swarm.config.infinispan.cache_container.TransportThreadPool;
import org.wildfly.swarm.config.infinispan.cache_container.PersistenceThreadPool;
import org.wildfly.swarm.config.infinispan.cache_container.ListenerThreadPool;
import org.wildfly.swarm.config.infinispan.cache_container.StateTransferThreadPool;
/**
* The configuration of an infinispan cache container
*/
@ResourceType("cache-container")
public class CacheContainer {
private String key;
private List aliases;
private String cacheManagerStatus;
private String clusterName;
private String coordinatorAddress;
private String defaultCache;
private Boolean isCoordinator;
private String jndiName;
private String localAddress;
private String module;
private Boolean statisticsEnabled;
private CacheContainerResources subresources = new CacheContainerResources();
private JgroupsTransport jgroupsTransport;
private RemoteCommandThreadPool remoteCommandThreadPool;
private AsyncOperationsThreadPool asyncOperationsThreadPool;
private ExpirationThreadPool expirationThreadPool;
private NoneTransport noneTransport;
private TransportThreadPool transportThreadPool;
private PersistenceThreadPool persistenceThreadPool;
private ListenerThreadPool listenerThreadPool;
private StateTransferThreadPool stateTransferThreadPool;
public CacheContainer(String key) {
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* The list of aliases for this cache container
*/
@ModelNodeBinding(detypedName = "aliases")
public List aliases() {
return this.aliases;
}
/**
* The list of aliases for this cache container
*/
@SuppressWarnings("unchecked")
public CacheContainer aliases(List value) {
this.aliases = value;
return (CacheContainer) this;
}
/**
* The status of the cache manager component. May return null if the cache manager is not started.
*/
@ModelNodeBinding(detypedName = "cache-manager-status")
public String cacheManagerStatus() {
return this.cacheManagerStatus;
}
/**
* The status of the cache manager component. May return null if the cache manager is not started.
*/
@SuppressWarnings("unchecked")
public CacheContainer cacheManagerStatus(String value) {
this.cacheManagerStatus = value;
return (CacheContainer) this;
}
/**
* The name of the cluster this node belongs to. May return null if the cache manager is not started.
*/
@ModelNodeBinding(detypedName = "cluster-name")
public String clusterName() {
return this.clusterName;
}
/**
* The name of the cluster this node belongs to. May return null if the cache manager is not started.
*/
@SuppressWarnings("unchecked")
public CacheContainer clusterName(String value) {
this.clusterName = value;
return (CacheContainer) this;
}
/**
* The logical address of the cluster's coordinator. May return null if the cache manager is not started.
*/
@ModelNodeBinding(detypedName = "coordinator-address")
public String coordinatorAddress() {
return this.coordinatorAddress;
}
/**
* The logical address of the cluster's coordinator. May return null if the cache manager is not started.
*/
@SuppressWarnings("unchecked")
public CacheContainer coordinatorAddress(String value) {
this.coordinatorAddress = value;
return (CacheContainer) this;
}
/**
* The default infinispan cache
*/
@ModelNodeBinding(detypedName = "default-cache")
public String defaultCache() {
return this.defaultCache;
}
/**
* The default infinispan cache
*/
@SuppressWarnings("unchecked")
public CacheContainer defaultCache(String value) {
this.defaultCache = value;
return (CacheContainer) this;
}
/**
* Set to true if this node is the cluster's coordinator. May return null if the cache manager is not started.
*/
@ModelNodeBinding(detypedName = "is-coordinator")
public Boolean isCoordinator() {
return this.isCoordinator;
}
/**
* Set to true if this node is the cluster's coordinator. May return null if the cache manager is not started.
*/
@SuppressWarnings("unchecked")
public CacheContainer isCoordinator(Boolean value) {
this.isCoordinator = value;
return (CacheContainer) this;
}
/**
* The jndi name to which to bind this cache container
*/
@ModelNodeBinding(detypedName = "jndi-name")
public String jndiName() {
return this.jndiName;
}
/**
* The jndi name to which to bind this cache container
*/
@SuppressWarnings("unchecked")
public CacheContainer jndiName(String value) {
this.jndiName = value;
return (CacheContainer) this;
}
/**
* The local address of the node. May return null if the cache manager is not started.
*/
@ModelNodeBinding(detypedName = "local-address")
public String localAddress() {
return this.localAddress;
}
/**
* The local address of the node. May return null if the cache manager is not started.
*/
@SuppressWarnings("unchecked")
public CacheContainer localAddress(String value) {
this.localAddress = value;
return (CacheContainer) this;
}
/**
* The module whose class loader should be used when building this cache container's configuration.
*/
@ModelNodeBinding(detypedName = "module")
public String module() {
return this.module;
}
/**
* The module whose class loader should be used when building this cache container's configuration.
*/
@SuppressWarnings("unchecked")
public CacheContainer module(String value) {
this.module = value;
return (CacheContainer) this;
}
/**
* If enabled, statistics will be collected for this cache container
*/
@ModelNodeBinding(detypedName = "statistics-enabled")
public Boolean statisticsEnabled() {
return this.statisticsEnabled;
}
/**
* If enabled, statistics will be collected for this cache container
*/
@SuppressWarnings("unchecked")
public CacheContainer statisticsEnabled(Boolean value) {
this.statisticsEnabled = value;
return (CacheContainer) this;
}
public CacheContainerResources subresources() {
return this.subresources;
}
/**
* Add all org.wildfly.swarm.config.infinispan.cache_container.InvalidationCache objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.infinispan.cache_container.InvalidationCache objects.
*/
@SuppressWarnings("unchecked")
public CacheContainer invalidationCaches(
List value) {
this.subresources.invalidationCaches.addAll(value);
return (CacheContainer) this;
}
/**
* Add the org.wildfly.swarm.config.infinispan.cache_container.InvalidationCache object to the list of subresources
* @param value The org.wildfly.swarm.config.infinispan.cache_container.InvalidationCache to add
* @return this
*/
@SuppressWarnings("unchecked")
public CacheContainer invalidationCache(InvalidationCache value) {
this.subresources.invalidationCaches.add(value);
return (CacheContainer) this;
}
/**
* Add all org.wildfly.swarm.config.infinispan.cache_container.LocalCache objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.infinispan.cache_container.LocalCache objects.
*/
@SuppressWarnings("unchecked")
public CacheContainer localCaches(
List value) {
this.subresources.localCaches.addAll(value);
return (CacheContainer) this;
}
/**
* Add the org.wildfly.swarm.config.infinispan.cache_container.LocalCache object to the list of subresources
* @param value The org.wildfly.swarm.config.infinispan.cache_container.LocalCache to add
* @return this
*/
@SuppressWarnings("unchecked")
public CacheContainer localCache(LocalCache value) {
this.subresources.localCaches.add(value);
return (CacheContainer) this;
}
/**
* Add all org.wildfly.swarm.config.infinispan.cache_container.ReplicatedCache objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.infinispan.cache_container.ReplicatedCache objects.
*/
@SuppressWarnings("unchecked")
public CacheContainer replicatedCaches(
List value) {
this.subresources.replicatedCaches.addAll(value);
return (CacheContainer) this;
}
/**
* Add the org.wildfly.swarm.config.infinispan.cache_container.ReplicatedCache object to the list of subresources
* @param value The org.wildfly.swarm.config.infinispan.cache_container.ReplicatedCache to add
* @return this
*/
@SuppressWarnings("unchecked")
public CacheContainer replicatedCache(ReplicatedCache value) {
this.subresources.replicatedCaches.add(value);
return (CacheContainer) this;
}
/**
* Add all org.wildfly.swarm.config.infinispan.cache_container.DistributedCache objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.infinispan.cache_container.DistributedCache objects.
*/
@SuppressWarnings("unchecked")
public CacheContainer distributedCaches(
List value) {
this.subresources.distributedCaches.addAll(value);
return (CacheContainer) this;
}
/**
* Add the org.wildfly.swarm.config.infinispan.cache_container.DistributedCache object to the list of subresources
* @param value The org.wildfly.swarm.config.infinispan.cache_container.DistributedCache to add
* @return this
*/
@SuppressWarnings("unchecked")
public CacheContainer distributedCache(DistributedCache value) {
this.subresources.distributedCaches.add(value);
return (CacheContainer) this;
}
/**
* Child mutators for CacheContainer
*/
public class CacheContainerResources {
/**
* An invalidation cache
*/
private List invalidationCaches = new java.util.ArrayList<>();
/**
* A local cache configuration
*/
private List localCaches = new java.util.ArrayList<>();
/**
* A replicated cache configuration
*/
private List replicatedCaches = new java.util.ArrayList<>();
/**
* A distributed cache configuration.
*/
private List distributedCaches = new java.util.ArrayList<>();
/**
* Get the list of org.wildfly.swarm.config.infinispan.cache_container.InvalidationCache resources
* @return the list of resources
*/
@Subresource
public List invalidationCaches() {
return this.invalidationCaches;
}
/**
* Get the list of org.wildfly.swarm.config.infinispan.cache_container.LocalCache resources
* @return the list of resources
*/
@Subresource
public List localCaches() {
return this.localCaches;
}
/**
* Get the list of org.wildfly.swarm.config.infinispan.cache_container.ReplicatedCache resources
* @return the list of resources
*/
@Subresource
public List replicatedCaches() {
return this.replicatedCaches;
}
/**
* Get the list of org.wildfly.swarm.config.infinispan.cache_container.DistributedCache resources
* @return the list of resources
*/
@Subresource
public List distributedCaches() {
return this.distributedCaches;
}
}
/**
* The description of the transport used by this cache container
*/
@Subresource
public JgroupsTransport jgroupsTransport() {
return this.jgroupsTransport;
}
/**
* The description of the transport used by this cache container
*/
@SuppressWarnings("unchecked")
public CacheContainer jgroupsTransport(JgroupsTransport value) {
this.jgroupsTransport = value;
return (CacheContainer) this;
}
/**
* Defines a thread pool used to execute remote commands.
*/
@Subresource
public RemoteCommandThreadPool remoteCommandThreadPool() {
return this.remoteCommandThreadPool;
}
/**
* Defines a thread pool used to execute remote commands.
*/
@SuppressWarnings("unchecked")
public CacheContainer remoteCommandThreadPool(RemoteCommandThreadPool value) {
this.remoteCommandThreadPool = value;
return (CacheContainer) this;
}
/**
* Defines a thread pool used for asynchronous operations.
*/
@Subresource
public AsyncOperationsThreadPool asyncOperationsThreadPool() {
return this.asyncOperationsThreadPool;
}
/**
* Defines a thread pool used for asynchronous operations.
*/
@SuppressWarnings("unchecked")
public CacheContainer asyncOperationsThreadPool(
AsyncOperationsThreadPool value) {
this.asyncOperationsThreadPool = value;
return (CacheContainer) this;
}
/**
* Defines a thread pool used for for evictions.
*/
@Subresource
public ExpirationThreadPool expirationThreadPool() {
return this.expirationThreadPool;
}
/**
* Defines a thread pool used for for evictions.
*/
@SuppressWarnings("unchecked")
public CacheContainer expirationThreadPool(ExpirationThreadPool value) {
this.expirationThreadPool = value;
return (CacheContainer) this;
}
/**
* A local-only transport used by this cache-container
*/
@Subresource
public NoneTransport noneTransport() {
return this.noneTransport;
}
/**
* A local-only transport used by this cache-container
*/
@SuppressWarnings("unchecked")
public CacheContainer noneTransport(NoneTransport value) {
this.noneTransport = value;
return (CacheContainer) this;
}
/**
* Defines a thread pool used for asynchronous transport communication.
*/
@Subresource
public TransportThreadPool transportThreadPool() {
return this.transportThreadPool;
}
/**
* Defines a thread pool used for asynchronous transport communication.
*/
@SuppressWarnings("unchecked")
public CacheContainer transportThreadPool(TransportThreadPool value) {
this.transportThreadPool = value;
return (CacheContainer) this;
}
/**
* Defines a thread pool used for interacting with the persistent store.
*/
@Subresource
public PersistenceThreadPool persistenceThreadPool() {
return this.persistenceThreadPool;
}
/**
* Defines a thread pool used for interacting with the persistent store.
*/
@SuppressWarnings("unchecked")
public CacheContainer persistenceThreadPool(PersistenceThreadPool value) {
this.persistenceThreadPool = value;
return (CacheContainer) this;
}
/**
* Defines a thread pool used for asynchronous cache listener notifications.
*/
@Subresource
public ListenerThreadPool listenerThreadPool() {
return this.listenerThreadPool;
}
/**
* Defines a thread pool used for asynchronous cache listener notifications.
*/
@SuppressWarnings("unchecked")
public CacheContainer listenerThreadPool(ListenerThreadPool value) {
this.listenerThreadPool = value;
return (CacheContainer) this;
}
/**
* Defines a thread pool used for for state transfer.
*/
@Subresource
public StateTransferThreadPool stateTransferThreadPool() {
return this.stateTransferThreadPool;
}
/**
* Defines a thread pool used for for state transfer.
*/
@SuppressWarnings("unchecked")
public CacheContainer stateTransferThreadPool(StateTransferThreadPool value) {
this.stateTransferThreadPool = value;
return (CacheContainer) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy