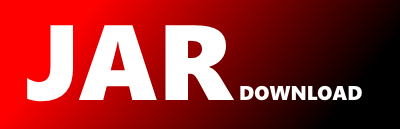
org.wildfly.swarm.config.jca.ShortRunningThreads Maven / Gradle / Ivy
package org.wildfly.swarm.config.jca;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.ResourceType;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.Map;
/**
* A thread pool executor with a bounded queue where threads submittings tasks will not block. Such a thread pool has a core and maximum size and a specified queue length. When a task is submitted, if the number of running threads is less than the core size, a new thread is created. Otherwise, if there is room in the queue, the task is enqueued. Otherwise, if the number of running threads is less than the maximum size, a new thread is created. Otherwise, the task is handed off to the designated handoff executor, if one is specified. Otherwise, the task is discarded.
*/
@ResourceType("short-running-threads")
public class ShortRunningThreads {
private String key;
private Boolean allowCoreTimeout;
private Integer coreThreads;
private Integer currentThreadCount;
private String handoffExecutor;
private Map keepaliveTime;
private Integer largestThreadCount;
private Integer maxThreads;
private String name;
private Integer queueLength;
private Integer queueSize;
private Integer rejectedCount;
private String threadFactory;
public ShortRunningThreads(String key) {
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* Whether core threads may time out.
*/
@ModelNodeBinding(detypedName = "allow-core-timeout")
public Boolean allowCoreTimeout() {
return this.allowCoreTimeout;
}
/**
* Whether core threads may time out.
*/
@SuppressWarnings("unchecked")
public ShortRunningThreads allowCoreTimeout(Boolean value) {
this.allowCoreTimeout = value;
return (ShortRunningThreads) this;
}
/**
* The core thread pool size which is smaller than the maximum pool size. If undefined, the core thread pool size is the same as the maximum thread pool size.
*/
@ModelNodeBinding(detypedName = "core-threads")
public Integer coreThreads() {
return this.coreThreads;
}
/**
* The core thread pool size which is smaller than the maximum pool size. If undefined, the core thread pool size is the same as the maximum thread pool size.
*/
@SuppressWarnings("unchecked")
public ShortRunningThreads coreThreads(Integer value) {
this.coreThreads = value;
return (ShortRunningThreads) this;
}
/**
* The current number of threads in the pool.
*/
@ModelNodeBinding(detypedName = "current-thread-count")
public Integer currentThreadCount() {
return this.currentThreadCount;
}
/**
* The current number of threads in the pool.
*/
@SuppressWarnings("unchecked")
public ShortRunningThreads currentThreadCount(Integer value) {
this.currentThreadCount = value;
return (ShortRunningThreads) this;
}
/**
* An executor to delegate tasks to in the event that a task cannot be accepted. If not specified, tasks that cannot be accepted will be silently discarded.
*/
@ModelNodeBinding(detypedName = "handoff-executor")
public String handoffExecutor() {
return this.handoffExecutor;
}
/**
* An executor to delegate tasks to in the event that a task cannot be accepted. If not specified, tasks that cannot be accepted will be silently discarded.
*/
@SuppressWarnings("unchecked")
public ShortRunningThreads handoffExecutor(String value) {
this.handoffExecutor = value;
return (ShortRunningThreads) this;
}
/**
* Used to specify the amount of time that pool threads should be kept running when idle; if not specified, threads will run until the executor is shut down.
*/
@ModelNodeBinding(detypedName = "keepalive-time")
public Map keepaliveTime() {
return this.keepaliveTime;
}
/**
* Used to specify the amount of time that pool threads should be kept running when idle; if not specified, threads will run until the executor is shut down.
*/
@SuppressWarnings("unchecked")
public ShortRunningThreads keepaliveTime(Map value) {
this.keepaliveTime = value;
return (ShortRunningThreads) this;
}
/**
* The largest number of threads that have ever simultaneously been in the pool.
*/
@ModelNodeBinding(detypedName = "largest-thread-count")
public Integer largestThreadCount() {
return this.largestThreadCount;
}
/**
* The largest number of threads that have ever simultaneously been in the pool.
*/
@SuppressWarnings("unchecked")
public ShortRunningThreads largestThreadCount(Integer value) {
this.largestThreadCount = value;
return (ShortRunningThreads) this;
}
/**
* The maximum thread pool size.
*/
@ModelNodeBinding(detypedName = "max-threads")
public Integer maxThreads() {
return this.maxThreads;
}
/**
* The maximum thread pool size.
*/
@SuppressWarnings("unchecked")
public ShortRunningThreads maxThreads(Integer value) {
this.maxThreads = value;
return (ShortRunningThreads) this;
}
/**
* The name of the thread pool.
*/
@ModelNodeBinding(detypedName = "name")
public String name() {
return this.name;
}
/**
* The name of the thread pool.
*/
@SuppressWarnings("unchecked")
public ShortRunningThreads name(String value) {
this.name = value;
return (ShortRunningThreads) this;
}
/**
* The queue length.
*/
@ModelNodeBinding(detypedName = "queue-length")
public Integer queueLength() {
return this.queueLength;
}
/**
* The queue length.
*/
@SuppressWarnings("unchecked")
public ShortRunningThreads queueLength(Integer value) {
this.queueLength = value;
return (ShortRunningThreads) this;
}
/**
* The queue size.
*/
@ModelNodeBinding(detypedName = "queue-size")
public Integer queueSize() {
return this.queueSize;
}
/**
* The queue size.
*/
@SuppressWarnings("unchecked")
public ShortRunningThreads queueSize(Integer value) {
this.queueSize = value;
return (ShortRunningThreads) this;
}
/**
* The number of tasks that have been passed to the handoff-executor (if one is specified) or discarded.
*/
@ModelNodeBinding(detypedName = "rejected-count")
public Integer rejectedCount() {
return this.rejectedCount;
}
/**
* The number of tasks that have been passed to the handoff-executor (if one is specified) or discarded.
*/
@SuppressWarnings("unchecked")
public ShortRunningThreads rejectedCount(Integer value) {
this.rejectedCount = value;
return (ShortRunningThreads) this;
}
/**
* Specifies the name of a specific thread factory to use to create worker threads. If not defined an appropriate default thread factory will be used.
*/
@ModelNodeBinding(detypedName = "thread-factory")
public String threadFactory() {
return this.threadFactory;
}
/**
* Specifies the name of a specific thread factory to use to create worker threads. If not defined an appropriate default thread factory will be used.
*/
@SuppressWarnings("unchecked")
public ShortRunningThreads threadFactory(String value) {
this.threadFactory = value;
return (ShortRunningThreads) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy