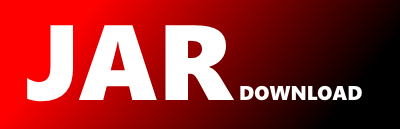
org.wildfly.swarm.config.jca.Workmanager Maven / Gradle / Ivy
package org.wildfly.swarm.config.jca;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.ResourceType;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.List;
import org.wildfly.config.runtime.Subresource;
/**
* WorkManager for resource adapters
*/
@ResourceType("workmanager")
public class Workmanager {
private String key;
private String name;
private WorkmanagerResources subresources = new WorkmanagerResources();
public Workmanager(String key) {
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* The name of the WorkManager
*/
@ModelNodeBinding(detypedName = "name")
public String name() {
return this.name;
}
/**
* The name of the WorkManager
*/
@SuppressWarnings("unchecked")
public Workmanager name(String value) {
this.name = value;
return (Workmanager) this;
}
public WorkmanagerResources subresources() {
return this.subresources;
}
/**
* Add all org.wildfly.swarm.config.jca.LongRunningThreads objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.jca.LongRunningThreads objects.
*/
@SuppressWarnings("unchecked")
public Workmanager longRunningThreads(
List value) {
this.subresources.longRunningThreads.addAll(value);
return (Workmanager) this;
}
/**
* Add the org.wildfly.swarm.config.jca.LongRunningThreads object to the list of subresources
* @param value The org.wildfly.swarm.config.jca.LongRunningThreads to add
* @return this
*/
@SuppressWarnings("unchecked")
public Workmanager longRunningThreads(LongRunningThreads value) {
this.subresources.longRunningThreads.add(value);
return (Workmanager) this;
}
/**
* Add all org.wildfly.swarm.config.jca.ShortRunningThreads objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.jca.ShortRunningThreads objects.
*/
@SuppressWarnings("unchecked")
public Workmanager shortRunningThreads(
List value) {
this.subresources.shortRunningThreads.addAll(value);
return (Workmanager) this;
}
/**
* Add the org.wildfly.swarm.config.jca.ShortRunningThreads object to the list of subresources
* @param value The org.wildfly.swarm.config.jca.ShortRunningThreads to add
* @return this
*/
@SuppressWarnings("unchecked")
public Workmanager shortRunningThreads(ShortRunningThreads value) {
this.subresources.shortRunningThreads.add(value);
return (Workmanager) this;
}
/**
* Child mutators for Workmanager
*/
public class WorkmanagerResources {
/**
* A thread pool executor with a bounded queue where threads submittings tasks will not block. Such a thread pool has a core and maximum size and a specified queue length. When a task is submitted, if the number of running threads is less than the core size, a new thread is created. Otherwise, if there is room in the queue, the task is enqueued. Otherwise, if the number of running threads is less than the maximum size, a new thread is created. Otherwise, the task is handed off to the designated handoff executor, if one is specified. Otherwise, the task is discarded.
*/
private List longRunningThreads = new java.util.ArrayList<>();
/**
* A thread pool executor with a bounded queue where threads submittings tasks will not block. Such a thread pool has a core and maximum size and a specified queue length. When a task is submitted, if the number of running threads is less than the core size, a new thread is created. Otherwise, if there is room in the queue, the task is enqueued. Otherwise, if the number of running threads is less than the maximum size, a new thread is created. Otherwise, the task is handed off to the designated handoff executor, if one is specified. Otherwise, the task is discarded.
*/
private List shortRunningThreads = new java.util.ArrayList<>();
/**
* Get the list of org.wildfly.swarm.config.jca.LongRunningThreads resources
* @return the list of resources
*/
@Subresource
public List longRunningThreads() {
return this.longRunningThreads;
}
/**
* Get the list of org.wildfly.swarm.config.jca.ShortRunningThreads resources
* @return the list of resources
*/
@Subresource
public List shortRunningThreads() {
return this.shortRunningThreads;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy