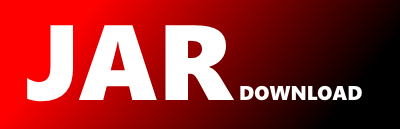
org.wildfly.swarm.config.logging.AsyncHandler Maven / Gradle / Ivy
package org.wildfly.swarm.config.logging;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.ResourceType;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.List;
/**
* Defines a handler which writes to the sub-handlers in an asynchronous thread. Used for handlers which introduce a substantial amount of lag.
*/
@ResourceType("async-handler")
public class AsyncHandler {
private String key;
private Boolean enabled;
private String filterSpec;
private String level;
private String overflowAction;
private Integer queueLength;
private List subhandlers;
public AsyncHandler(String key) {
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* If set to true the handler is enabled and functioning as normal, if set to false the handler is ignored when processing log messages.
*/
@ModelNodeBinding(detypedName = "enabled")
public Boolean enabled() {
return this.enabled;
}
/**
* If set to true the handler is enabled and functioning as normal, if set to false the handler is ignored when processing log messages.
*/
@SuppressWarnings("unchecked")
public AsyncHandler enabled(Boolean value) {
this.enabled = value;
return (AsyncHandler) this;
}
/**
* A filter expression value to define a filter. Example for a filter that does not match a pattern: not(match("JBAS.*"))
*/
@ModelNodeBinding(detypedName = "filter-spec")
public String filterSpec() {
return this.filterSpec;
}
/**
* A filter expression value to define a filter. Example for a filter that does not match a pattern: not(match("JBAS.*"))
*/
@SuppressWarnings("unchecked")
public AsyncHandler filterSpec(String value) {
this.filterSpec = value;
return (AsyncHandler) this;
}
/**
* The log level specifying which message levels will be logged by this handler. Message levels lower than this value will be discarded.
*/
@ModelNodeBinding(detypedName = "level")
public String level() {
return this.level;
}
/**
* The log level specifying which message levels will be logged by this handler. Message levels lower than this value will be discarded.
*/
@SuppressWarnings("unchecked")
public AsyncHandler level(String value) {
this.level = value;
return (AsyncHandler) this;
}
/**
* Specify what action to take when the overflowing. The valid options are 'block' and 'discard'
*/
@ModelNodeBinding(detypedName = "overflow-action")
public String overflowAction() {
return this.overflowAction;
}
/**
* Specify what action to take when the overflowing. The valid options are 'block' and 'discard'
*/
@SuppressWarnings("unchecked")
public AsyncHandler overflowAction(String value) {
this.overflowAction = value;
return (AsyncHandler) this;
}
/**
* The queue length to use before flushing writing
*/
@ModelNodeBinding(detypedName = "queue-length")
public Integer queueLength() {
return this.queueLength;
}
/**
* The queue length to use before flushing writing
*/
@SuppressWarnings("unchecked")
public AsyncHandler queueLength(Integer value) {
this.queueLength = value;
return (AsyncHandler) this;
}
/**
* The Handlers associated with this async handler.
*/
@ModelNodeBinding(detypedName = "subhandlers")
public List subhandlers() {
return this.subhandlers;
}
/**
* The Handlers associated with this async handler.
*/
@SuppressWarnings("unchecked")
public AsyncHandler subhandlers(List value) {
this.subhandlers = value;
return (AsyncHandler) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy