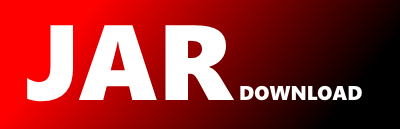
org.wildfly.swarm.config.logging.CustomHandler Maven / Gradle / Ivy
package org.wildfly.swarm.config.logging;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.ResourceType;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.Map;
/**
* Defines a custom logging handler. The custom handler must extend java.util.logging.Handler.
*/
@ResourceType("custom-handler")
public class CustomHandler {
private String key;
private String attributeClass;
private Boolean enabled;
private String encoding;
private String filterSpec;
private String formatter;
private String level;
private String module;
private String namedFormatter;
private Map properties;
public CustomHandler(String key) {
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* The logging handler class to be used.
*/
@ModelNodeBinding(detypedName = "class")
public String attributeClass() {
return this.attributeClass;
}
/**
* The logging handler class to be used.
*/
@SuppressWarnings("unchecked")
public CustomHandler attributeClass(String value) {
this.attributeClass = value;
return (CustomHandler) this;
}
/**
* If set to true the handler is enabled and functioning as normal, if set to false the handler is ignored when processing log messages.
*/
@ModelNodeBinding(detypedName = "enabled")
public Boolean enabled() {
return this.enabled;
}
/**
* If set to true the handler is enabled and functioning as normal, if set to false the handler is ignored when processing log messages.
*/
@SuppressWarnings("unchecked")
public CustomHandler enabled(Boolean value) {
this.enabled = value;
return (CustomHandler) this;
}
/**
* The character encoding used by this Handler.
*/
@ModelNodeBinding(detypedName = "encoding")
public String encoding() {
return this.encoding;
}
/**
* The character encoding used by this Handler.
*/
@SuppressWarnings("unchecked")
public CustomHandler encoding(String value) {
this.encoding = value;
return (CustomHandler) this;
}
/**
* A filter expression value to define a filter. Example for a filter that does not match a pattern: not(match("JBAS.*"))
*/
@ModelNodeBinding(detypedName = "filter-spec")
public String filterSpec() {
return this.filterSpec;
}
/**
* A filter expression value to define a filter. Example for a filter that does not match a pattern: not(match("JBAS.*"))
*/
@SuppressWarnings("unchecked")
public CustomHandler filterSpec(String value) {
this.filterSpec = value;
return (CustomHandler) this;
}
/**
* Defines a pattern for the formatter.
*/
@ModelNodeBinding(detypedName = "formatter")
public String formatter() {
return this.formatter;
}
/**
* Defines a pattern for the formatter.
*/
@SuppressWarnings("unchecked")
public CustomHandler formatter(String value) {
this.formatter = value;
return (CustomHandler) this;
}
/**
* The log level specifying which message levels will be logged by this logger. Message levels lower than this value will be discarded.
*/
@ModelNodeBinding(detypedName = "level")
public String level() {
return this.level;
}
/**
* The log level specifying which message levels will be logged by this logger. Message levels lower than this value will be discarded.
*/
@SuppressWarnings("unchecked")
public CustomHandler level(String value) {
this.level = value;
return (CustomHandler) this;
}
/**
* The module that the logging handler depends on.
*/
@ModelNodeBinding(detypedName = "module")
public String module() {
return this.module;
}
/**
* The module that the logging handler depends on.
*/
@SuppressWarnings("unchecked")
public CustomHandler module(String value) {
this.module = value;
return (CustomHandler) this;
}
/**
* The name of the defined formatter to be used on the handler.
*/
@ModelNodeBinding(detypedName = "named-formatter")
public String namedFormatter() {
return this.namedFormatter;
}
/**
* The name of the defined formatter to be used on the handler.
*/
@SuppressWarnings("unchecked")
public CustomHandler namedFormatter(String value) {
this.namedFormatter = value;
return (CustomHandler) this;
}
/**
* Defines the properties used for the logging handler. All properties must be accessible via a setter method.
*/
@ModelNodeBinding(detypedName = "properties")
public Map properties() {
return this.properties;
}
/**
* Defines the properties used for the logging handler. All properties must be accessible via a setter method.
*/
@SuppressWarnings("unchecked")
public CustomHandler properties(Map value) {
this.properties = value;
return (CustomHandler) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy