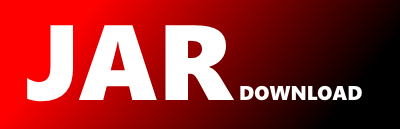
org.wildfly.swarm.config.mail.MailSession Maven / Gradle / Ivy
package org.wildfly.swarm.config.mail;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.ResourceType;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.List;
import org.wildfly.config.runtime.Subresource;
import org.wildfly.swarm.config.mail.mail_session.Custom;
import org.wildfly.swarm.config.mail.mail_session.Pop3Server;
import org.wildfly.swarm.config.mail.mail_session.SmtpServer;
import org.wildfly.swarm.config.mail.mail_session.ImapServer;
/**
* Mail session definition
*/
@ResourceType("mail-session")
public class MailSession {
private String key;
private Boolean debug;
private String from;
private String jndiName;
private MailSessionResources subresources = new MailSessionResources();
private Pop3Server pop3Server;
private SmtpServer smtpServer;
private ImapServer imapServer;
public MailSession(String key) {
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* Enables JavaMail debugging
*/
@ModelNodeBinding(detypedName = "debug")
public Boolean debug() {
return this.debug;
}
/**
* Enables JavaMail debugging
*/
@SuppressWarnings("unchecked")
public MailSession debug(Boolean value) {
this.debug = value;
return (MailSession) this;
}
/**
* From address that is used as default from, if not set when sending
*/
@ModelNodeBinding(detypedName = "from")
public String from() {
return this.from;
}
/**
* From address that is used as default from, if not set when sending
*/
@SuppressWarnings("unchecked")
public MailSession from(String value) {
this.from = value;
return (MailSession) this;
}
/**
* JNDI name to where mail session should be bound
*/
@ModelNodeBinding(detypedName = "jndi-name")
public String jndiName() {
return this.jndiName;
}
/**
* JNDI name to where mail session should be bound
*/
@SuppressWarnings("unchecked")
public MailSession jndiName(String value) {
this.jndiName = value;
return (MailSession) this;
}
public MailSessionResources subresources() {
return this.subresources;
}
/**
* Add all org.wildfly.swarm.config.mail.mail_session.Custom objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.mail.mail_session.Custom objects.
*/
@SuppressWarnings("unchecked")
public MailSession customs(
List value) {
this.subresources.customs.addAll(value);
return (MailSession) this;
}
/**
* Add the org.wildfly.swarm.config.mail.mail_session.Custom object to the list of subresources
* @param value The org.wildfly.swarm.config.mail.mail_session.Custom to add
* @return this
*/
@SuppressWarnings("unchecked")
public MailSession custom(Custom value) {
this.subresources.customs.add(value);
return (MailSession) this;
}
/**
* Child mutators for MailSession
*/
public class MailSessionResources {
/**
* Mail session server
*/
private List customs = new java.util.ArrayList<>();
/**
* Get the list of org.wildfly.swarm.config.mail.mail_session.Custom resources
* @return the list of resources
*/
@Subresource
public List customs() {
return this.customs;
}
}
/**
* Mail session server
*/
@Subresource
public Pop3Server pop3Server() {
return this.pop3Server;
}
/**
* Mail session server
*/
@SuppressWarnings("unchecked")
public MailSession pop3Server(Pop3Server value) {
this.pop3Server = value;
return (MailSession) this;
}
/**
* Mail session server
*/
@Subresource
public SmtpServer smtpServer() {
return this.smtpServer;
}
/**
* Mail session server
*/
@SuppressWarnings("unchecked")
public MailSession smtpServer(SmtpServer value) {
this.smtpServer = value;
return (MailSession) this;
}
/**
* Mail session server
*/
@Subresource
public ImapServer imapServer() {
return this.imapServer;
}
/**
* Mail session server
*/
@SuppressWarnings("unchecked")
public MailSession imapServer(ImapServer value) {
this.imapServer = value;
return (MailSession) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy