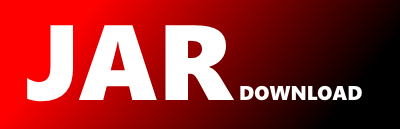
org.wildfly.swarm.config.messaging_activemq.JmsBridge Maven / Gradle / Ivy
package org.wildfly.swarm.config.messaging_activemq;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.ResourceType;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.Map;
/**
* A JMS bridge instance.
*/
@ResourceType("jms-bridge")
public class JmsBridge {
private String key;
private Boolean addMessageidInHeader;
private String clientId;
private Long failureRetryInterval;
private Integer maxBatchSize;
private Long maxBatchTime;
private Integer maxRetries;
private String module;
private Boolean paused;
private String qualityOfService;
private String selector;
private Boolean started;
private String subscriptionName;
private String sourceConnectionFactory;
private Map sourceContext;
private String sourceDestination;
private String sourcePassword;
private String sourceUser;
private String targetConnectionFactory;
private Map targetContext;
private String targetDestination;
private String targetPassword;
private String targetUser;
public JmsBridge(String key) {
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* If true, then the original message's message ID will be appended in the message sent to the destination in the header AMQ_BRIDGE_MSG_ID_LIST. If the message is bridged more than once, each message ID will be appended.
*/
@ModelNodeBinding(detypedName = "add-messageID-in-header")
public Boolean addMessageidInHeader() {
return this.addMessageidInHeader;
}
/**
* If true, then the original message's message ID will be appended in the message sent to the destination in the header AMQ_BRIDGE_MSG_ID_LIST. If the message is bridged more than once, each message ID will be appended.
*/
@SuppressWarnings("unchecked")
public JmsBridge addMessageidInHeader(Boolean value) {
this.addMessageidInHeader = value;
return (JmsBridge) this;
}
/**
* The JMS client ID to use when creating/looking up the subscription if it is durable and the source destination is a topic.
*/
@ModelNodeBinding(detypedName = "client-id")
public String clientId() {
return this.clientId;
}
/**
* The JMS client ID to use when creating/looking up the subscription if it is durable and the source destination is a topic.
*/
@SuppressWarnings("unchecked")
public JmsBridge clientId(String value) {
this.clientId = value;
return (JmsBridge) this;
}
/**
* The amount of time in milliseconds to wait between trying to recreate connections to the source or target servers when the bridge has detected they have failed.
*/
@ModelNodeBinding(detypedName = "failure-retry-interval")
public Long failureRetryInterval() {
return this.failureRetryInterval;
}
/**
* The amount of time in milliseconds to wait between trying to recreate connections to the source or target servers when the bridge has detected they have failed.
*/
@SuppressWarnings("unchecked")
public JmsBridge failureRetryInterval(Long value) {
this.failureRetryInterval = value;
return (JmsBridge) this;
}
/**
* The maximum number of messages to consume from the source destination before sending them in a batch to the target destination. Its value must >= 1.
*/
@ModelNodeBinding(detypedName = "max-batch-size")
public Integer maxBatchSize() {
return this.maxBatchSize;
}
/**
* The maximum number of messages to consume from the source destination before sending them in a batch to the target destination. Its value must >= 1.
*/
@SuppressWarnings("unchecked")
public JmsBridge maxBatchSize(Integer value) {
this.maxBatchSize = value;
return (JmsBridge) this;
}
/**
* The maximum number of milliseconds to wait before sending a batch to target, even if the number of messages consumed has not reached max-batch-size. Its value must be -1 to represent 'wait forever', or >= 1 to specify an actual time.
*/
@ModelNodeBinding(detypedName = "max-batch-time")
public Long maxBatchTime() {
return this.maxBatchTime;
}
/**
* The maximum number of milliseconds to wait before sending a batch to target, even if the number of messages consumed has not reached max-batch-size. Its value must be -1 to represent 'wait forever', or >= 1 to specify an actual time.
*/
@SuppressWarnings("unchecked")
public JmsBridge maxBatchTime(Long value) {
this.maxBatchTime = value;
return (JmsBridge) this;
}
/**
* The number of times to attempt to recreate connections to the source or target servers when the bridge has detected they have failed. The bridge will give up after trying this number of times. -1 represents 'try forever'.
*/
@ModelNodeBinding(detypedName = "max-retries")
public Integer maxRetries() {
return this.maxRetries;
}
/**
* The number of times to attempt to recreate connections to the source or target servers when the bridge has detected they have failed. The bridge will give up after trying this number of times. -1 represents 'try forever'.
*/
@SuppressWarnings("unchecked")
public JmsBridge maxRetries(Integer value) {
this.maxRetries = value;
return (JmsBridge) this;
}
/**
* The name of AS7 module containing the resources required to lookup source and target JMS resources.
*/
@ModelNodeBinding(detypedName = "module")
public String module() {
return this.module;
}
/**
* The name of AS7 module containing the resources required to lookup source and target JMS resources.
*/
@SuppressWarnings("unchecked")
public JmsBridge module(String value) {
this.module = value;
return (JmsBridge) this;
}
/**
* Whether the JMS bridge is paused.
*/
@ModelNodeBinding(detypedName = "paused")
public Boolean paused() {
return this.paused;
}
/**
* Whether the JMS bridge is paused.
*/
@SuppressWarnings("unchecked")
public JmsBridge paused(Boolean value) {
this.paused = value;
return (JmsBridge) this;
}
/**
* The desired quality of service mode (AT_MOST_ONCE, DUPLICATES_OK or ONCE_AND_ONLY_ONCE).
*/
@ModelNodeBinding(detypedName = "quality-of-service")
public String qualityOfService() {
return this.qualityOfService;
}
/**
* The desired quality of service mode (AT_MOST_ONCE, DUPLICATES_OK or ONCE_AND_ONLY_ONCE).
*/
@SuppressWarnings("unchecked")
public JmsBridge qualityOfService(String value) {
this.qualityOfService = value;
return (JmsBridge) this;
}
/**
* A JMS selector expression used for consuming messages from the source destination. Only messages that match the selector expression will be bridged from the source to the target destination.
*/
@ModelNodeBinding(detypedName = "selector")
public String selector() {
return this.selector;
}
/**
* A JMS selector expression used for consuming messages from the source destination. Only messages that match the selector expression will be bridged from the source to the target destination.
*/
@SuppressWarnings("unchecked")
public JmsBridge selector(String value) {
this.selector = value;
return (JmsBridge) this;
}
/**
* Whether the JMS bridge is started.
*/
@ModelNodeBinding(detypedName = "started")
public Boolean started() {
return this.started;
}
/**
* Whether the JMS bridge is started.
*/
@SuppressWarnings("unchecked")
public JmsBridge started(Boolean value) {
this.started = value;
return (JmsBridge) this;
}
/**
* The name of the subscription if it is durable and the source destination is a topic.
*/
@ModelNodeBinding(detypedName = "subscription-name")
public String subscriptionName() {
return this.subscriptionName;
}
/**
* The name of the subscription if it is durable and the source destination is a topic.
*/
@SuppressWarnings("unchecked")
public JmsBridge subscriptionName(String value) {
this.subscriptionName = value;
return (JmsBridge) this;
}
/**
* The name of the source connection factory to lookup on the source messaging server.
*/
@ModelNodeBinding(detypedName = "source-connection-factory")
public String sourceConnectionFactory() {
return this.sourceConnectionFactory;
}
/**
* The name of the source connection factory to lookup on the source messaging server.
*/
@SuppressWarnings("unchecked")
public JmsBridge sourceConnectionFactory(String value) {
this.sourceConnectionFactory = value;
return (JmsBridge) this;
}
/**
* The properties used to configure the source JNDI initial context.
*/
@ModelNodeBinding(detypedName = "source-context")
public Map sourceContext() {
return this.sourceContext;
}
/**
* The properties used to configure the source JNDI initial context.
*/
@SuppressWarnings("unchecked")
public JmsBridge sourceContext(Map value) {
this.sourceContext = value;
return (JmsBridge) this;
}
/**
* The name of the source destination to lookup on the source messaging server.
*/
@ModelNodeBinding(detypedName = "source-destination")
public String sourceDestination() {
return this.sourceDestination;
}
/**
* The name of the source destination to lookup on the source messaging server.
*/
@SuppressWarnings("unchecked")
public JmsBridge sourceDestination(String value) {
this.sourceDestination = value;
return (JmsBridge) this;
}
/**
* The password for creating the source connection.
*/
@ModelNodeBinding(detypedName = "source-password")
public String sourcePassword() {
return this.sourcePassword;
}
/**
* The password for creating the source connection.
*/
@SuppressWarnings("unchecked")
public JmsBridge sourcePassword(String value) {
this.sourcePassword = value;
return (JmsBridge) this;
}
/**
* The name of the user for creating the source connection.
*/
@ModelNodeBinding(detypedName = "source-user")
public String sourceUser() {
return this.sourceUser;
}
/**
* The name of the user for creating the source connection.
*/
@SuppressWarnings("unchecked")
public JmsBridge sourceUser(String value) {
this.sourceUser = value;
return (JmsBridge) this;
}
/**
* The name of the target connection factory to lookup on the target messaging server.
*/
@ModelNodeBinding(detypedName = "target-connection-factory")
public String targetConnectionFactory() {
return this.targetConnectionFactory;
}
/**
* The name of the target connection factory to lookup on the target messaging server.
*/
@SuppressWarnings("unchecked")
public JmsBridge targetConnectionFactory(String value) {
this.targetConnectionFactory = value;
return (JmsBridge) this;
}
/**
* The properties used to configure the target JNDI initial context.
*/
@ModelNodeBinding(detypedName = "target-context")
public Map targetContext() {
return this.targetContext;
}
/**
* The properties used to configure the target JNDI initial context.
*/
@SuppressWarnings("unchecked")
public JmsBridge targetContext(Map value) {
this.targetContext = value;
return (JmsBridge) this;
}
/**
* The name of the target destination to lookup on the target messaging server.
*/
@ModelNodeBinding(detypedName = "target-destination")
public String targetDestination() {
return this.targetDestination;
}
/**
* The name of the target destination to lookup on the target messaging server.
*/
@SuppressWarnings("unchecked")
public JmsBridge targetDestination(String value) {
this.targetDestination = value;
return (JmsBridge) this;
}
/**
* The password for creating the target connection.
*/
@ModelNodeBinding(detypedName = "target-password")
public String targetPassword() {
return this.targetPassword;
}
/**
* The password for creating the target connection.
*/
@SuppressWarnings("unchecked")
public JmsBridge targetPassword(String value) {
this.targetPassword = value;
return (JmsBridge) this;
}
/**
* The name of the user for creating the target connection.
*/
@ModelNodeBinding(detypedName = "target-user")
public String targetUser() {
return this.targetUser;
}
/**
* The name of the user for creating the target connection.
*/
@SuppressWarnings("unchecked")
public JmsBridge targetUser(String value) {
this.targetUser = value;
return (JmsBridge) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy