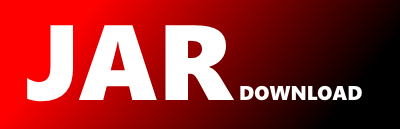
org.wildfly.swarm.config.naming.Binding Maven / Gradle / Ivy
package org.wildfly.swarm.config.naming;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.ResourceType;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.Map;
/**
* JNDI bindings for primitive types
*/
@ResourceType("binding")
public class Binding {
private String key;
private String bindingType;
private Boolean cache;
private String attributeClass;
private Map environment;
private String lookup;
private String module;
private String type;
private String value;
public Binding(String key) {
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* The type of binding to create, may be simple, lookup, external-context or object-factory
*/
@ModelNodeBinding(detypedName = "binding-type")
public String bindingType() {
return this.bindingType;
}
/**
* The type of binding to create, may be simple, lookup, external-context or object-factory
*/
@SuppressWarnings("unchecked")
public Binding bindingType(String value) {
this.bindingType = value;
return (Binding) this;
}
/**
* If the external context should be cached
*/
@ModelNodeBinding(detypedName = "cache")
public Boolean cache() {
return this.cache;
}
/**
* If the external context should be cached
*/
@SuppressWarnings("unchecked")
public Binding cache(Boolean value) {
this.cache = value;
return (Binding) this;
}
/**
* The object factory class name for object factory bindings
*/
@ModelNodeBinding(detypedName = "class")
public String attributeClass() {
return this.attributeClass;
}
/**
* The object factory class name for object factory bindings
*/
@SuppressWarnings("unchecked")
public Binding attributeClass(String value) {
this.attributeClass = value;
return (Binding) this;
}
/**
* The environment to use on object factory instance retrieval
*/
@ModelNodeBinding(detypedName = "environment")
public Map environment() {
return this.environment;
}
/**
* The environment to use on object factory instance retrieval
*/
@SuppressWarnings("unchecked")
public Binding environment(Map value) {
this.environment = value;
return (Binding) this;
}
/**
* The entry to lookup in JNDI for lookup bindings
*/
@ModelNodeBinding(detypedName = "lookup")
public String lookup() {
return this.lookup;
}
/**
* The entry to lookup in JNDI for lookup bindings
*/
@SuppressWarnings("unchecked")
public Binding lookup(String value) {
this.lookup = value;
return (Binding) this;
}
/**
* The module to load the object factory from for object factory bindings
*/
@ModelNodeBinding(detypedName = "module")
public String module() {
return this.module;
}
/**
* The module to load the object factory from for object factory bindings
*/
@SuppressWarnings("unchecked")
public Binding module(String value) {
this.module = value;
return (Binding) this;
}
/**
* The type of the value to bind for simple bindings, this must be a primitive type
*/
@ModelNodeBinding(detypedName = "type")
public String type() {
return this.type;
}
/**
* The type of the value to bind for simple bindings, this must be a primitive type
*/
@SuppressWarnings("unchecked")
public Binding type(String value) {
this.type = value;
return (Binding) this;
}
/**
* The value to bind for simple bindings
*/
@ModelNodeBinding(detypedName = "value")
public String value() {
return this.value;
}
/**
* The value to bind for simple bindings
*/
@SuppressWarnings("unchecked")
public Binding value(String value) {
this.value = value;
return (Binding) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy