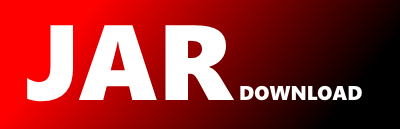
org.wildfly.swarm.config.remoting.HttpConnector Maven / Gradle / Ivy
package org.wildfly.swarm.config.remoting;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.ResourceType;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.List;
import org.wildfly.config.runtime.Subresource;
/**
* The configuration of a HTTP Upgrade based Remoting connector.
*/
@ResourceType("http-connector")
public class HttpConnector {
private String key;
private String authenticationProvider;
private String connectorRef;
private String saslProtocol;
private String securityRealm;
private String serverName;
private HttpConnectorResources subresources = new HttpConnectorResources();
private SaslSecurity saslSecurity;
public HttpConnector(String key) {
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* The "authentication-provider" element contains the name of the authentication provider to use for incoming connections.
*/
@ModelNodeBinding(detypedName = "authentication-provider")
public String authenticationProvider() {
return this.authenticationProvider;
}
/**
* The "authentication-provider" element contains the name of the authentication provider to use for incoming connections.
*/
@SuppressWarnings("unchecked")
public HttpConnector authenticationProvider(String value) {
this.authenticationProvider = value;
return (HttpConnector) this;
}
/**
* The name (or names) of a connector in the Undertow subsystem to connect to.
*/
@ModelNodeBinding(detypedName = "connector-ref")
public String connectorRef() {
return this.connectorRef;
}
/**
* The name (or names) of a connector in the Undertow subsystem to connect to.
*/
@SuppressWarnings("unchecked")
public HttpConnector connectorRef(String value) {
this.connectorRef = value;
return (HttpConnector) this;
}
/**
* The protocol to pass into the SASL mechanisms used for authentication.
*/
@ModelNodeBinding(detypedName = "sasl-protocol")
public String saslProtocol() {
return this.saslProtocol;
}
/**
* The protocol to pass into the SASL mechanisms used for authentication.
*/
@SuppressWarnings("unchecked")
public HttpConnector saslProtocol(String value) {
this.saslProtocol = value;
return (HttpConnector) this;
}
/**
* The associated security realm to use for authentication for this connector.
*/
@ModelNodeBinding(detypedName = "security-realm")
public String securityRealm() {
return this.securityRealm;
}
/**
* The associated security realm to use for authentication for this connector.
*/
@SuppressWarnings("unchecked")
public HttpConnector securityRealm(String value) {
this.securityRealm = value;
return (HttpConnector) this;
}
/**
* The server name to send in the initial message exchange and for SASL based authentication.
*/
@ModelNodeBinding(detypedName = "server-name")
public String serverName() {
return this.serverName;
}
/**
* The server name to send in the initial message exchange and for SASL based authentication.
*/
@SuppressWarnings("unchecked")
public HttpConnector serverName(String value) {
this.serverName = value;
return (HttpConnector) this;
}
public HttpConnectorResources subresources() {
return this.subresources;
}
/**
* Add all org.wildfly.swarm.config.remoting.Property objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.remoting.Property objects.
*/
@SuppressWarnings("unchecked")
public HttpConnector propertys(
List value) {
this.subresources.propertys.addAll(value);
return (HttpConnector) this;
}
/**
* Add the org.wildfly.swarm.config.remoting.Property object to the list of subresources
* @param value The org.wildfly.swarm.config.remoting.Property to add
* @return this
*/
@SuppressWarnings("unchecked")
public HttpConnector property(Property value) {
this.subresources.propertys.add(value);
return (HttpConnector) this;
}
/**
* Child mutators for HttpConnector
*/
public class HttpConnectorResources {
/**
* Properties supported by the underlying provider. The property name is inferred from the last element of the properties address.
*/
private List propertys = new java.util.ArrayList<>();
/**
* Get the list of org.wildfly.swarm.config.remoting.Property resources
* @return the list of resources
*/
@Subresource
public List propertys() {
return this.propertys;
}
}
/**
* The "sasl" element contains the SASL authentication configuration for this connector.
*/
@Subresource
public SaslSecurity saslSecurity() {
return this.saslSecurity;
}
/**
* The "sasl" element contains the SASL authentication configuration for this connector.
*/
@SuppressWarnings("unchecked")
public HttpConnector saslSecurity(SaslSecurity value) {
this.saslSecurity = value;
return (HttpConnector) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy