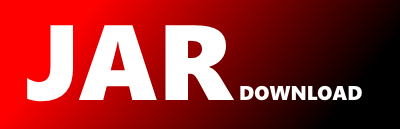
org.wildfly.swarm.config.remoting.PolicySaslPolicy Maven / Gradle / Ivy
package org.wildfly.swarm.config.remoting;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.ResourceType;
import org.wildfly.config.runtime.ModelNodeBinding;
/**
* The policy configuration.
*/
@ResourceType("sasl-policy")
@Implicit
public class PolicySaslPolicy {
private String key;
private Boolean forwardSecrecy;
private Boolean noActive;
private Boolean noAnonymous;
private Boolean noDictionary;
private Boolean noPlainText;
private Boolean passCredentials;
public PolicySaslPolicy() {
this.key = "policy";
}
public String getKey() {
return this.key;
}
/**
* The optional nested "forward-secrecy" element contains a boolean value which specifies whether mechanisms that implement forward secrecy between sessions are required. Forward secrecy means that breaking into one session will not automatically provide information for breaking into future sessions.
*/
@ModelNodeBinding(detypedName = "forward-secrecy")
public Boolean forwardSecrecy() {
return this.forwardSecrecy;
}
/**
* The optional nested "forward-secrecy" element contains a boolean value which specifies whether mechanisms that implement forward secrecy between sessions are required. Forward secrecy means that breaking into one session will not automatically provide information for breaking into future sessions.
*/
@SuppressWarnings("unchecked")
public PolicySaslPolicy forwardSecrecy(Boolean value) {
this.forwardSecrecy = value;
return (PolicySaslPolicy) this;
}
/**
* The optional nested "no-active" element contains a boolean value which specifies whether mechanisms susceptible to active (non-dictionary) attacks are not permitted. "false" to permit, "true" to deny.
*/
@ModelNodeBinding(detypedName = "no-active")
public Boolean noActive() {
return this.noActive;
}
/**
* The optional nested "no-active" element contains a boolean value which specifies whether mechanisms susceptible to active (non-dictionary) attacks are not permitted. "false" to permit, "true" to deny.
*/
@SuppressWarnings("unchecked")
public PolicySaslPolicy noActive(Boolean value) {
this.noActive = value;
return (PolicySaslPolicy) this;
}
/**
* The optional nested "no-anonymous" element contains a boolean value which specifies whether mechanisms that accept anonymous login are permitted. "false" to permit, "true" to deny.
*/
@ModelNodeBinding(detypedName = "no-anonymous")
public Boolean noAnonymous() {
return this.noAnonymous;
}
/**
* The optional nested "no-anonymous" element contains a boolean value which specifies whether mechanisms that accept anonymous login are permitted. "false" to permit, "true" to deny.
*/
@SuppressWarnings("unchecked")
public PolicySaslPolicy noAnonymous(Boolean value) {
this.noAnonymous = value;
return (PolicySaslPolicy) this;
}
/**
* The optional nested "no-dictionary" element contains a boolean value which specifies whether mechanisms susceptible to passive dictionary attacks are permitted. "false" to permit, "true" to deny.
*/
@ModelNodeBinding(detypedName = "no-dictionary")
public Boolean noDictionary() {
return this.noDictionary;
}
/**
* The optional nested "no-dictionary" element contains a boolean value which specifies whether mechanisms susceptible to passive dictionary attacks are permitted. "false" to permit, "true" to deny.
*/
@SuppressWarnings("unchecked")
public PolicySaslPolicy noDictionary(Boolean value) {
this.noDictionary = value;
return (PolicySaslPolicy) this;
}
/**
* The optional nested "no-plain-text" element contains a boolean value which specifies whether mechanisms susceptible to simple plain passive attacks (e.g., "PLAIN") are not permitted. "false" to permit, "true" to deny.
*/
@ModelNodeBinding(detypedName = "no-plain-text")
public Boolean noPlainText() {
return this.noPlainText;
}
/**
* The optional nested "no-plain-text" element contains a boolean value which specifies whether mechanisms susceptible to simple plain passive attacks (e.g., "PLAIN") are not permitted. "false" to permit, "true" to deny.
*/
@SuppressWarnings("unchecked")
public PolicySaslPolicy noPlainText(Boolean value) {
this.noPlainText = value;
return (PolicySaslPolicy) this;
}
/**
* The optional nested "pass-credentials" element contains a boolean value which specifies whether mechanisms that pass client credentials are required.
*/
@ModelNodeBinding(detypedName = "pass-credentials")
public Boolean passCredentials() {
return this.passCredentials;
}
/**
* The optional nested "pass-credentials" element contains a boolean value which specifies whether mechanisms that pass client credentials are required.
*/
@SuppressWarnings("unchecked")
public PolicySaslPolicy passCredentials(Boolean value) {
this.passCredentials = value;
return (PolicySaslPolicy) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy