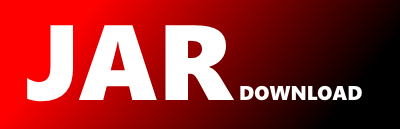
org.wildfly.swarm.config.remoting.SaslSecurity Maven / Gradle / Ivy
package org.wildfly.swarm.config.remoting;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.ResourceType;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.List;
import org.wildfly.config.runtime.Subresource;
/**
* The "sasl" element contains the SASL authentication configuration for this connector.
*/
@ResourceType("security")
@Implicit
public class SaslSecurity {
private String key;
private List includeMechanisms;
private List qop;
private Boolean reuseSession;
private Boolean serverAuth;
private List strength;
private SaslSecurityResources subresources = new SaslSecurityResources();
private PolicySaslPolicy policySaslPolicy;
public SaslSecurity() {
this.key = "sasl";
}
public String getKey() {
return this.key;
}
/**
* The optional nested "include-mechanisms" element contains a whitelist of allowed SASL mechanism names. No mechanisms will be allowed which are not present in this list.
*/
@ModelNodeBinding(detypedName = "include-mechanisms")
public List includeMechanisms() {
return this.includeMechanisms;
}
/**
* The optional nested "include-mechanisms" element contains a whitelist of allowed SASL mechanism names. No mechanisms will be allowed which are not present in this list.
*/
@SuppressWarnings("unchecked")
public SaslSecurity includeMechanisms(List value) {
this.includeMechanisms = value;
return (SaslSecurity) this;
}
/**
* The optional nested "qop" element contains a list of quality-of-protection values, in decreasing order of preference.
*/
@ModelNodeBinding(detypedName = "qop")
public List qop() {
return this.qop;
}
/**
* The optional nested "qop" element contains a list of quality-of-protection values, in decreasing order of preference.
*/
@SuppressWarnings("unchecked")
public SaslSecurity qop(List value) {
this.qop = value;
return (SaslSecurity) this;
}
/**
* The optional nested "reuse-session" boolean element specifies whether or not the server should attempt to reuse previously authenticated session information. The mechanism may or may not support such reuse, and other factors may also prevent it.
*/
@ModelNodeBinding(detypedName = "reuse-session")
public Boolean reuseSession() {
return this.reuseSession;
}
/**
* The optional nested "reuse-session" boolean element specifies whether or not the server should attempt to reuse previously authenticated session information. The mechanism may or may not support such reuse, and other factors may also prevent it.
*/
@SuppressWarnings("unchecked")
public SaslSecurity reuseSession(Boolean value) {
this.reuseSession = value;
return (SaslSecurity) this;
}
/**
* The optional nested "server-auth" boolean element specifies whether the server should authenticate to the client. Not all mechanisms may support this setting.
*/
@ModelNodeBinding(detypedName = "server-auth")
public Boolean serverAuth() {
return this.serverAuth;
}
/**
* The optional nested "server-auth" boolean element specifies whether the server should authenticate to the client. Not all mechanisms may support this setting.
*/
@SuppressWarnings("unchecked")
public SaslSecurity serverAuth(Boolean value) {
this.serverAuth = value;
return (SaslSecurity) this;
}
/**
* The optional nested "strength" element contains a list of cipher strength values, in decreasing order of preference.
*/
@ModelNodeBinding(detypedName = "strength")
public List strength() {
return this.strength;
}
/**
* The optional nested "strength" element contains a list of cipher strength values, in decreasing order of preference.
*/
@SuppressWarnings("unchecked")
public SaslSecurity strength(List value) {
this.strength = value;
return (SaslSecurity) this;
}
public SaslSecurityResources subresources() {
return this.subresources;
}
/**
* Add all org.wildfly.swarm.config.remoting.Property objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.remoting.Property objects.
*/
@SuppressWarnings("unchecked")
public SaslSecurity propertys(
List value) {
this.subresources.propertys.addAll(value);
return (SaslSecurity) this;
}
/**
* Add the org.wildfly.swarm.config.remoting.Property object to the list of subresources
* @param value The org.wildfly.swarm.config.remoting.Property to add
* @return this
*/
@SuppressWarnings("unchecked")
public SaslSecurity property(Property value) {
this.subresources.propertys.add(value);
return (SaslSecurity) this;
}
/**
* Child mutators for SaslSecurity
*/
public class SaslSecurityResources {
/**
* Properties supported by the underlying provider. The property name is inferred from the last element of the properties address.
*/
private List propertys = new java.util.ArrayList<>();
/**
* Get the list of org.wildfly.swarm.config.remoting.Property resources
* @return the list of resources
*/
@Subresource
public List propertys() {
return this.propertys;
}
}
/**
* The policy configuration.
*/
@Subresource
public PolicySaslPolicy policySaslPolicy() {
return this.policySaslPolicy;
}
/**
* The policy configuration.
*/
@SuppressWarnings("unchecked")
public SaslSecurity policySaslPolicy(PolicySaslPolicy value) {
this.policySaslPolicy = value;
return (SaslSecurity) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy