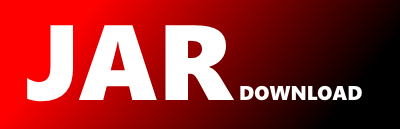
org.wildfly.swarm.config.undertow.Server Maven / Gradle / Ivy
package org.wildfly.swarm.config.undertow;
import org.wildfly.config.runtime.Implicit;
import org.wildfly.config.runtime.ResourceType;
import org.wildfly.config.runtime.ModelNodeBinding;
import java.util.List;
import org.wildfly.config.runtime.Subresource;
import org.wildfly.swarm.config.undertow.server.Host;
import org.wildfly.swarm.config.undertow.server.HttpListener;
import org.wildfly.swarm.config.undertow.server.AjpListener;
import org.wildfly.swarm.config.undertow.server.HttpsListener;
/**
* A server
*/
@ResourceType("server")
public class Server {
private String key;
private String defaultHost;
private String servletContainer;
private ServerResources subresources = new ServerResources();
public Server(String key) {
this.key = key;
}
public String getKey() {
return this.key;
}
/**
* The servers default virtual host
*/
@ModelNodeBinding(detypedName = "default-host")
public String defaultHost() {
return this.defaultHost;
}
/**
* The servers default virtual host
*/
@SuppressWarnings("unchecked")
public Server defaultHost(String value) {
this.defaultHost = value;
return (Server) this;
}
/**
* The servers default servlet container
*/
@ModelNodeBinding(detypedName = "servlet-container")
public String servletContainer() {
return this.servletContainer;
}
/**
* The servers default servlet container
*/
@SuppressWarnings("unchecked")
public Server servletContainer(String value) {
this.servletContainer = value;
return (Server) this;
}
public ServerResources subresources() {
return this.subresources;
}
/**
* Add all org.wildfly.swarm.config.undertow.server.Host objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.undertow.server.Host objects.
*/
@SuppressWarnings("unchecked")
public Server hosts(
List value) {
this.subresources.hosts.addAll(value);
return (Server) this;
}
/**
* Add the org.wildfly.swarm.config.undertow.server.Host object to the list of subresources
* @param value The org.wildfly.swarm.config.undertow.server.Host to add
* @return this
*/
@SuppressWarnings("unchecked")
public Server host(Host value) {
this.subresources.hosts.add(value);
return (Server) this;
}
/**
* Add all org.wildfly.swarm.config.undertow.server.HttpListener objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.undertow.server.HttpListener objects.
*/
@SuppressWarnings("unchecked")
public Server httpListeners(
List value) {
this.subresources.httpListeners.addAll(value);
return (Server) this;
}
/**
* Add the org.wildfly.swarm.config.undertow.server.HttpListener object to the list of subresources
* @param value The org.wildfly.swarm.config.undertow.server.HttpListener to add
* @return this
*/
@SuppressWarnings("unchecked")
public Server httpListener(HttpListener value) {
this.subresources.httpListeners.add(value);
return (Server) this;
}
/**
* Add all org.wildfly.swarm.config.undertow.server.AjpListener objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.undertow.server.AjpListener objects.
*/
@SuppressWarnings("unchecked")
public Server ajpListeners(
List value) {
this.subresources.ajpListeners.addAll(value);
return (Server) this;
}
/**
* Add the org.wildfly.swarm.config.undertow.server.AjpListener object to the list of subresources
* @param value The org.wildfly.swarm.config.undertow.server.AjpListener to add
* @return this
*/
@SuppressWarnings("unchecked")
public Server ajpListener(AjpListener value) {
this.subresources.ajpListeners.add(value);
return (Server) this;
}
/**
* Add all org.wildfly.swarm.config.undertow.server.HttpsListener objects to this subresource
* @return this
* @param value List of org.wildfly.swarm.config.undertow.server.HttpsListener objects.
*/
@SuppressWarnings("unchecked")
public Server httpsListeners(
List value) {
this.subresources.httpsListeners.addAll(value);
return (Server) this;
}
/**
* Add the org.wildfly.swarm.config.undertow.server.HttpsListener object to the list of subresources
* @param value The org.wildfly.swarm.config.undertow.server.HttpsListener to add
* @return this
*/
@SuppressWarnings("unchecked")
public Server httpsListener(HttpsListener value) {
this.subresources.httpsListeners.add(value);
return (Server) this;
}
/**
* Child mutators for Server
*/
public class ServerResources {
/**
* An Undertow host
*/
private List hosts = new java.util.ArrayList<>();
/**
* http listener
*/
private List httpListeners = new java.util.ArrayList<>();
/**
* http listener
*/
private List ajpListeners = new java.util.ArrayList<>();
/**
* http listener
*/
private List httpsListeners = new java.util.ArrayList<>();
/**
* Get the list of org.wildfly.swarm.config.undertow.server.Host resources
* @return the list of resources
*/
@Subresource
public List hosts() {
return this.hosts;
}
/**
* Get the list of org.wildfly.swarm.config.undertow.server.HttpListener resources
* @return the list of resources
*/
@Subresource
public List httpListeners() {
return this.httpListeners;
}
/**
* Get the list of org.wildfly.swarm.config.undertow.server.AjpListener resources
* @return the list of resources
*/
@Subresource
public List ajpListeners() {
return this.ajpListeners;
}
/**
* Get the list of org.wildfly.swarm.config.undertow.server.HttpsListener resources
* @return the list of resources
*/
@Subresource
public List httpsListeners() {
return this.httpsListeners;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy